Java SE 8 New Features Ed 1
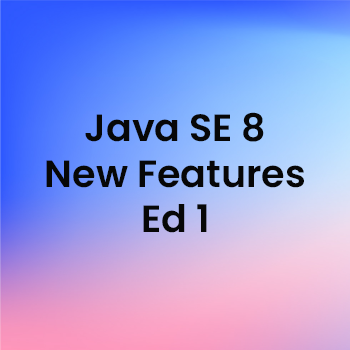
Product
|
Oracle
|
Code
|
D80897GC10
|
Duration
|
2 Days
|
Price (baht)
|
39,200
|
About the course
1. Introduction
- Course Goal 1-2
- Course Objectives 1-3
- Introductions 1-4
- Audience 1-5
- Prerequisites 1-6
- Course Map 1-7
- Schedule 1-8
- Practice Environment 1-10
- Additional Resources 1-11
- What Is not Covered in this Course 1-12
- Compact Profiles 1-13
- Compact Profile Purpose 1-14
- Compact Profile Table 1-15
- Annotations 1-16
- Java FX 1-17
- Security 1-18
- Concurrency 1-19
- Practice 1-20
2. Lambda Introduction
- Objectives 2-2
- Inner Classes 2-3
- Anonymous Inner Class 2-4
- Anonymous Inner Class Drawbacks 2-5
- Lambda Expression 2-6
- Vertical Problem Solved 2-7
- Lambda Expression Defined 2-8
- By What Magic? 2-9
- Functional Interfaces 2-10
- Summary 2-11
- Practices 2-12
3. A Case for Lambda Expressions
- Objectives 3-2
- Why Lambda Now? 3-3
- A Common Example 3-4
- Person Properties 3-5
- Robocall01 Demo 3-6
- RoboCall01 Recap 3-7
- Robocall02 Demo 3-8
- Robocall02 Recap 3-9
- RoboCall03 Demo 3-10
- A Test Interface 3-11
- Test Class Already Exists 3-12
- Robocall03 Recap 3-13
- Robocall04 Demo 3-14
- What Is Replaced? 3-15
- What Is a Lambda Expression? 3-16
- Lambda Expression: Recap 3-18
- Summary 3-19
- Practice 3-20
4. Filtering Collections with Lambdas
- Objectives 4-2
- RoboCall06 Iterating with forEach 4-3
- RoboCallTest07: Stream and Filter 4-4
- RobocallTest08: Stream and Filter Again 4-5
- RoboCall Summary 4-6
- SalesTxn Class 4-7
- Builder Pattern 4-8
- Java Streams 4-9
- The Filter Method 4-10
- Method References 4-11
- Method Chaining 4-12
- Pipeline Defined 4-14
- Summary 4-15
- Practices 4-16
- Java Puzzle Ball 4-17
- Game Debriefing: Quiz 4-18
- Game Debriefing: Concepts 4-19
- Game Debriefing: Quiz 4-20
- Solutions 4-21
5. Using Built-in Lambda Types
- Objectives 5-2
- Why Discuss Functional Interfaces? 5-3
- The java.util.function Package 5-4
- Example Assumptions 5-5
- Predicate 5-6
- Predicate: Example 5-7
- Consumer 5-8
- Consumer: Example 5-9
- Function 5-10
- Function: Example 5-11
- Supplier 5-12
- Supplier: Example 5-13
- Primitive Interface 5-14
- Return a Primitive Type 5-15
- Return a Primitive Type: Example 5-16
- Process a Primitive Type 5-17
- Process Primitive Type: Example 5-18
- Binary Types 5-19
- Binary Type: Example 5-20
- UnaryOperator: Example 5-21
- Wildcard Generics Review 5-22
- Summary 5-23
- Practices 5-24
6. Collection Operations with Lambda
- Objectives 6-2
- Streams API 6-3
- Types of Operations 6-4
- Extracting Data with Map 6-5
- Taking a Peek 6-6
- Search Methods: Overview 6-7
- Search Methods 6-8
- Optional Class 6-9
- Lazy Operations 6-10
- Stream Data Methods 6-11
- Performing Calculations 6-12
- Sorting 6-13
- Comparator Updates 6-14
- Saving Data from a Stream 6-15
- Collectors Class 6-16
- Summary 6-17
- Practices 6-18
7. Parallel Streams
- Objectives 7-2
- Streams Review 7-3
- Old Style Collection Processing 7-4
- New Style Collection Processing 7-5
- Stream Pipeline: Another Look 7-6
- Styles Compared 7-7
- Parallel Stream 7-8
- Using Parallel Streams: Collection 7-9
- Using Parallel Streams: From a Stream 7-10
- Pipelines Fine Print 7-11
- Avoid Statefulness 7-12
- Embrace Statelessness 7-13
- Streams Are Deterministic for Most Part 7-14
- Some Are Not Deterministic 7-15
- Reduction 7-16
- Reduction Fine Print 7-17
- Reduction: Example 7-18
- A Look Under the Hood 7-24
- Illustrating Parallel Execution 7-25
- Performance 7-36
- A Simple Performance Model 7-37
- Summary 7-38
- Practices 7-39
8. Lambda Cookbook
- Objectives 8-2
- ArrayList Enhancements 8-3
- Map Enhancements 8-4
- Map Merge 8-5
- Map Stream 8-6
- Quick Streams with Stream.of 8-7
- BufferedReader File Stream 8-8
- NIO File Stream 8-9
- Read File into ArrayList 8-10
- List the Contents of a Directory 8-11
- Walk the Directory Structure 8-12
- Search a Directory Structure 8-13
- Flatten Data with flatMap 8-14
- Summary 8-15
- Practices 8-16
9. Method Enhancements
- Objectives 9-2
- The Debate: Evolving Technology 9-3
- Pros and Cons 9-4
- Building Good Libraries 9-5
- Evolving Interfaces and the Collections Library 9-6
- static Methods in Interfaces 9-7
- default Methods in Interfaces 9-8
- Multiple Inheritance in Java 9-9
- Inheritance Rules of default Methods 9-10
- Summary 9-13
- Practices 9-14
10. Working with Local Dates and Times
- Objectives 10-2
- Why Is Date and Time Important? 10-3
- Previous Java Date and Time 10-4
- Java Date and Time API Goals 10-5
- Working with Local Date and Time 10-6
- Working with LocalDate 10-7
- LocalDate: Example 10-8
- Working with LocalTime 10-9
- LocalTime: Example 10-10
- Working with LocalDateTime 10-11
- LocalTimeDate: Example 10-12
- Date and Time Methods 10-13
- Summary 10-14
- Practice 10-15
11. Working with Time Zones
- Objectives 11-2
- Working with Time Zones 11-3
- Daylight Savings Time Rules 11-4
- Modeling Time Zones 11-5
- Creating ZonedDateTime Objects 11-6
- Working with ZonedDateTime Gaps/Overlaps 11-7
- ZoneRules 11-8
- Working Across Time Zones 11-9
- Summary 11-10
- Practice 11-11
12. Working with Date and Time Amounts
- Objectives 12-2
- Date and Time Amounts 12-3
- Period 12-4
- Duration 12-5
- Calculating Between Days 12-6
- Making Dates Pretty 12-7
- Using Fluent Notation 12-8
- Summary 12-9
- Practice 12-10
13. JavaScript on Java with Nashorn
- Objectives 13-2
- What Is Nashorn? 13-3
- Nashorn Use Cases 13-4
- Command-line Tool: jjs 13-5
- The jjs Tool 13-6
- Additional Scripting Features 13-8
- Nashorn Global Objects 13-9
- Nashorn Global Functions 13-10
- Additional Resources 13-12
- Summary 13-13
- Practice 13-14
14. JavaScript on Java with Nashorn
- Objectives 14-2
- Using Java in JavaScript 14-3
- Accessing Java Classes 14-4
- Constructing Java Objects 14-5
- Invoking methods 14-6
- Using JavaBeans 14-7
- Working with Java Arrays 14-8
- Working with Java Collections 14-10
- Implementing Java Interfaces 14-11
- Extending Abstract Java Classes 14-13
- Extending Concrete Java Classes 14-14
- Accessing Methods of a SuperClass 14-15
- Accessing a Method Overload Variant 14-16
- Summary 14-17
- Practice 14-18
15. JavaScript on Java with Nashorn
- Objectives 15-2
- Evaluating JavaScript in Java 15-3
- Passing a Java Object to JavaScript 15-4
- Invoking a JavaScript Function 15-6
- Invoking a Method of a JavaScript Object 15-7
- Nashorn Security 15-8
- JavaFX and Nashorn 15-9
- JavaFX: Example 15-10
- JavaFX JavaScript Example 15-12
- JavaFX Example Refined 15-13
- Summary 15-14
- Practices 15-15
16. Java Mission Control
- Objectives 16-2
- Mission Control 16-3
- JMX and MBeans 16-4
- Mission Control Management Console: Overview Tab 16-5
- MBeans: MBean Browser Tab 16-6
- MBeans: Operations Tab 16-7
- MBeans: Notifications Tab 16-8
- MBeans: Metadata Tab 16-9
- MBeans: Triggers Tab 16-10
- Runtime: System Tab 16-11
- Runtime: Memory Tab 16-12
- Runtime: Threads Tab 16-13
- Diagnostics Tab 16-14
- Summary 16-15
- Practices 16-16
17. Java Flight Recorder
- Objectives 17-2
- Java Flight Recorder 17-3
- How Java Flight Recorder Works 17-4
- Flight Recordings 17-5
- Starting a Flight Recording 17-6
- Reviewing the JFR User Interface 17-7
- JVM Browser 17-8
- JFR General Tab Group 17-9
- General: Range Navigator 17-10
- Events: Overview 17-11
- Events: Log 17-12
- Events: Graph 17-13
- Events: Threads 17-14
- Memory: Overview 17-15
- Memory: Garbage Collections 17-16
- Memory: GC Times 17-17
- Memory: GC Configuration 17-18
- Memory: Allocations 17-19
- Memory: Object Statistics 17-20
- Code: Overview 17-21
- Code: Hot Methods 17-22
- Code: Exceptions 17-23
- Threads: Overview 17-24
- Threads: Hot Threads 17-25
- Threads: Latencies 17-26
- Threads: Thread Dumps 17-27
- I/O: Socket Read 17-28
- System: Overview 17-29
- Configuring Java Flight Recorder 17-30
- Avoiding Common JFR Pitfalls 17-31
- Summary 17-32
- Practices 17-33
18. Oracle Cloud
- Agenda 18-2
- What is Cloud? 18-3
- What is Cloud Computing? 18-4
- History – Cloud Evolution 18-5
- Components of Cloud Computing 18-6
- Characteristics of Cloud 18-7
- Cloud Deployment Models 18-8
- Cloud Service Models 18-9
- Industry Shifting from On-Premises to the Cloud 18-13
- Oracle IaaS Overview 18-15
- Oracle PaaS Overview 18-16
- Oracle SaaS Overview 18-17
- Summary 18-18
19. Oracle Application Container Cloud Service Overview
- Objectives 19-2
- Oracle Application Container Cloud Service 19-3
- Oracle Application Container Cloud 19-4
- Polyglot Platform 19-5
- Open Platform 19-6
- Container-based Application Platform as a Service 19-7
- Elastic Scaling 19-8
- Profiling 19-9
- Manageable 19-10
- Deploy—Application Archive (Zip) 19-12
- Application Deployment 19-13
- Application Container Cloud Architecture 19-14
- Load Balancer 19-15
- Oracle Developer Cloud Service 19-16
- Developer Cloud Service – Easy Adoption/Integration 19-17
- Application Container Cloud Service Advantages 19-19
- Summary 19-20
Register for Training
Schedule
Start Date | End Date | Duration (days) | Price | Code |
---|---|---|---|---|
10/7/2024 | 10/11/2024 | 5 | 20,000 | AZ-204T00 |
11/4/2024 | 11/8/2024 | 5 | 20,000 | AZ-204T00 |
12/9/2024 | 12/13/2024 | 5 | 20,000 | AZ-204T00 |
1/6/2025 | 1/10/2025 | 5 | 20,000 | AZ-204T00 |
2/3/2025 | 2/7/2025 | 5 | 20,000 | AZ-204T00 |
3/3/2025 | 3/7/2025 | 5 | 20,000 | AZ-204T00 |
4/21/2025 | 4/25/2025 | 5 | 20,000 | AZ-204T00 |
5/19/2025 | 5/23/2025 | 5 | 20,000 | AZ-204T00 |
6/9/2025 | 6/13/2025 | 5 | 20,000 | AZ-204T00 |
7/14/2025 | 7/18/2025 | 5 | 20,000 | AZ-204T00 |
8/4/2025 | 8/8/2025 | 5 | 20,000 | AZ-204T00 |
9/1/2025 | 9/5/2025 | 5 | 20,000 | AZ-204T00 |
10/6/2025 | 10/10/2025 | 5 | 20,000 | AZ-204T00 |
11/3/2025 | 11/7/2025 | 5 | 20,000 | AZ-204T00 |
12/8/2025 | 12/12/2025 | 5 | 20,000 | AZ-204T00 |
6/6/2023 | 6/9/2023 | 4 | 16,000 | AZ-220T00 |
7/24/2023 | 7/27/2023 | 4 | 16,000 | AZ-220T00 |
8/15/2023 | 8/18/2023 | 4 | 16,000 | AZ-220T00 |
9/5/2023 | 9/8/2023 | 4 | 16,000 | AZ-220T00 |
10/9/2023 | 10/12/2023 | 4 | 16,000 | AZ-220T00 |
11/7/2023 | 11/10/2023 | 4 | 16,000 | AZ-220T00 |
12/12/2023 | 12/15/2023 | 4 | 16,000 | AZ-220T00 |
10/8/2024 | 10/11/2024 | 4 | 16,000 | AZ-400T00 |
11/5/2024 | 11/8/2024 | 4 | 16,000 | AZ-400T00 |
12/10/2024 | 12/13/2024 | 4 | 16,000 | AZ-400T00 |
1/6/2025 | 1/9/2025 | 4 | 16,000 | AZ-400T00 |
2/4/2025 | 2/7/2025 | 4 | 16,000 | AZ-400T00 |
3/4/2025 | 3/7/2025 | 4 | 16,000 | AZ-400T00 |
4/8/2025 | 4/11/2025 | 4 | 16,000 | AZ-400T00 |
5/6/2025 | 5/9/2025 | 4 | 16,000 | AZ-400T00 |
6/2/2025 | 6/6/2025 | 4 | 16,000 | AZ-400T00 |
7/29/2025 | 8/1/2025 | 4 | 16,000 | AZ-400T00 |
8/11/2025 | 8/15/2025 | 4 | 16,000 | AZ-400T00 |
9/9/2025 | 9/12/2025 | 4 | 16,000 | AZ-400T00 |
10/14/2025 | 10/17/2025 | 4 | 16,000 | AZ-400T00 |
11/4/2025 | 11/7/2025 | 4 | 16,000 | AZ-400T00 |
12/1/2025 | 12/4/2025 | 4 | 16,000 | AZ-400T00 |
10/7/2024 | 10/11/2024 | 5 | 20,000 | AZ-040T00 |
11/4/2024 | 11/8/2024 | 5 | 20,000 | AZ-040T00 |
12/9/2024 | 12/13/2024 | 5 | 20,000 | AZ-040T00 |
1/6/2025 | 1/10/2025 | 5 | 20,000 | AZ-040T00 |
2/3/2025 | 2/7/2025 | 5 | 20,000 | AZ-040T00 |
3/24/2025 | 3/28/2025 | 5 | 20,000 | AZ-040T00 |
4/21/2025 | 4/25/2025 | 5 | 20,000 | AZ-040T00 |
5/19/2025 | 5/23/2025 | 5 | 20,000 | AZ-040T00 |
6/9/2025 | 6/13/2025 | 5 | 20,000 | AZ-040T00 |
6/30/2025 | 7/4/2025 | 5 | 20,000 | AZ-040T00 |
8/4/2025 | 8/8/2025 | 5 | 20,000 | AZ-040T00 |
9/1/2025 | 9/5/2025 | 5 | 20,000 | AZ-040T00 |
10/6/2025 | 10/10/2025 | 5 | 20,000 | AZ-040T00 |
11/3/2025 | 11/7/2025 | 5 | 20,000 | AZ-040T00 |
12/8/2025 | 12/12/2025 | 5 | 20,000 | AZ-040T00 |
10/15/2024 | 10/18/2024 | 4 | 16,000 | AZ-104T00(T) |
11/5/2024 | 11/8/2024 | 4 | 16,000 | AZ-104T00(T) |
12/10/2024 | 12/13/2024 | 4 | 16,000 | AZ-104T00(T) |
1/6/2025 | 1/9/2025 | 4 | 16,000 | AZ-104T00(T) |
2/4/2025 | 2/7/2025 | 4 | 16,000 | AZ-104T00(T) |
3/11/2025 | 3/14/2025 | 4 | 16,000 | AZ-104T00(T) |
4/22/2025 | 4/25/2025 | 4 | 16,000 | AZ-104T00(T) |
5/27/2025 | 5/30/2025 | 4 | 16,000 | AZ-104T00(T) |
6/2/2025 | 6/6/2025 | 4 | 16,000 | AZ-104T00(T) |
7/15/2025 | 7/18/2025 | 4 | 16,000 | AZ-104T00(T) |
8/11/2025 | 8/15/2025 | 4 | 16,000 | AZ-104T00(T) |
9/9/2025 | 9/12/2025 | 4 | 16,000 | AZ-104T00(T) |
10/14/2025 | 10/17/2025 | 4 | 16,000 | AZ-104T00(T) |
11/4/2025 | 11/7/2025 | 4 | 16,000 | AZ-104T00(T) |
12/1/2025 | 12/4/2025 | 4 | 16,000 | AZ-104T00(T) |
10/15/2024 | 10/17/2024 | 3 | 12,000 | AZ-120T00 |
11/11/2024 | 11/13/2024 | 3 | 12,000 | AZ-120T00 |
12/11/2024 | 12/13/2024 | 3 | 12,000 | AZ-120T00 |
1/13/0205 | 1/15/2025 | 3 | 12,000 | AZ-120T00 |
2/10/2025 | 2/13/2025 | 3 | 12,000 | AZ-120T00 |
3/10/2025 | 3/12/2025 | 3 | 12,000 | AZ-120T00 |
4/2/2025 | 4/4/2025 | 3 | 12,000 | AZ-120T00 |
5/14/2025 | 5/16/2025 | 3 | 12,000 | AZ-120T00 |
6/9/2025 | 6/11/2025 | 3 | 12,000 | AZ-120T00 |
7/7/2025 | 7/9/2025 | 3 | 12,000 | AZ-120T00 |
8/13/2025 | 8/15/2025 | 3 | 12,000 | AZ-120T00 |
9/10/2025 | 9/12/2025 | 3 | 12,000 | AZ-120T00 |
10/15/2025 | 10/17/2025 | 3 | 12,000 | AZ-120T00 |
11/11/2025 | 11/13/2025 | 3 | 12,000 | AZ-120T00 |
12/10/2025 | 12/12/2025 | 3 | 12,000 | AZ-120T00 |
10/28/2024 | 10/31/2024 | 4 | 16,000 | AZ-140T00 |
11/19/2024 | 11/22/2024 | 4 | 16,000 | AZ-140T00 |
12/17/2024 | 12/20/2024 | 4 | 16,000 | AZ-140T00 |
1/21/2025 | 1/24/2025 | 4 | 16,000 | AZ-140T00 |
2/18/2025 | 2/21/2025 | 4 | 16,000 | AZ-140T00 |
3/18/2025 | 3/21/2025 | 4 | 16,000 | AZ-140T00 |
4/22/2025 | 4/25/2025 | 4 | 16,000 | AZ-140T00 |
5/27/2025 | 5/30/2025 | 4 | 16,000 | AZ-140T00 |
6/24/2025 | 6/27/2025 | 4 | 16,000 | AZ-140T00 |
7/22/2025 | 7/25/2025 | 4 | 16,000 | AZ-140T00 |
8/18/2025 | 8/21/2025 | 4 | 16,000 | AZ-140T00 |
9/16/2025 | 9/19/2025 | 4 | 16,000 | AZ-140T00 |
10/27/2025 | 10/30/2025 | 4 | 16,000 | AZ-140T00 |
11/18/2025 | 11/21/2025 | 4 | 16,000 | AZ-140T00 |
12/16/2025 | 12/19/2025 | 4 | 16,000 | AZ-140T00 |
10/8/2024 | 10/11/2024 | 4 | 16,000 | AZ-305T00 |
11/12/2024 | 11/15/2024 | 4 | 16,000 | AZ-305T00 |
12/10/2024 | 12/13/2024 | 4 | 16,000 | AZ-305T00 |
1/14/2025 | 1/17/2025 | 4 | 16,000 | AZ-305T00 |
2/10/2025 | 2/13/2024 | 4 | 16,000 | AZ-305T00 |
3/11/2025 | 3/15/2025 | 4 | 16,000 | AZ-305T00 |
4/22/2025 | 4/25/2025 | 4 | 16,000 | AZ-305T00 |
5/13/2025 | 5/16/2025 | 4 | 16,000 | AZ-305T00 |
6/2/2025 | 6/6/2025 | 4 | 16,000 | AZ-305T00 |
7/15/2025 | 7/18/2025 | 4 | 16,000 | AZ-305T00 |
8/5/2025 | 8/8/2025 | 4 | 16,000 | AZ-305T00 |
9/9/2025 | 9/12/2025 | 4 | 16,000 | AZ-305T00 |
10/7/2025 | 10/10/2025 | 4 | 16,000 | AZ-305T00 |
11/11/2025 | 11/14/2025 | 4 | 16,000 | AZ-305T00 |
12/9/2025 | 12/12/2025 | 4 | 16,000 | AZ-305T00 |
6/6/2023 | 6/9/2023 | 4 | 16,000 | AZ-600T00 |
7/24/2023 | 7/27/2023 | 4 | 16,000 | AZ-600T00 |
8/15/2023 | 8/18/2023 | 4 | 16,000 | AZ-600T00 |
9/5/2023 | 9/8/2023 | 4 | 16,000 | AZ-600T00 |
10/9/2023 | 10/12/2023 | 4 | 16,000 | AZ-600T00 |
11/7/2023 | 11/10/2023 | 4 | 16,000 | AZ-600T00 |
12/12/2023 | 12/15/2023 | 4 | 16,000 | AZ-600T00 |
10/2/2024 | 10/4/2024 | 3 | 12,000 | AZ-700T00 |
11/6/2024 | 11/8/2024 | 3 | 12,000 | AZ-700T00 |
12/11/2024 | 12/13/2024 | 3 | 12,000 | AZ-700T00 |
1/8/2025 | 1/10/2025 | 3 | 12,000 | AZ-700T00 |
2/5/2025 | 2/7/2025 | 3 | 12,000 | AZ-700T00 |
3/5/2025 | 3/7/2025 | 3 | 12,000 | AZ-700T00 |
4/2/2025 | 4/4/2025 | 3 | 12,000 | AZ-700T00 |
5/7/2025 | 5/9/2025 | 3 | 12,000 | AZ-700T00 |
6/4/2025 | 6/6/2025 | 3 | 12,000 | AZ-700T00 |
7/7/2025 | 7/9/2025 | 3 | 12,000 | AZ-700T00 |
8/13/2025 | 8/15/2025 | 3 | 12,000 | AZ-700T00 |
9/3/2025 | 9/5/2025 | 3 | 12,000 | AZ-700T00 |
10/1/2025 | 10/3/2025 | 3 | 12,000 | AZ-700T00 |
11/5/2025 | 11/7/2025 | 3 | 12,000 | AZ-700T00 |
12/10/2025 | 12/12/2025 | 3 | 12,000 | AZ-700T00 |
6/7/2023 | 6/9/2023 | 3 | 12,000 | AZ-720T00 |
7/12/2023 | 7/14/2023 | 3 | 12,000 | AZ-720T00 |
8/2/2023 | 8/4/2023 | 3 | 12,000 | AZ-720T00 |
9/6/2023 | 9/8/2023 | 3 | 12,000 | AZ-720T00 |
10/4/2023 | 10/6/2023 | 3 | 12,000 | AZ-720T00 |
11/1/2023 | 11/3/2023 | 3 | 12,000 | AZ-720T00 |
12/6/2023 | 12/8/2023 | 3 | 12,000 | AZ-720T00 |
10/8/2024 | 10/11/2024 | 4 | 16,000 | AZ-800T00(T) |
11/12/2024 | 11/15/2024 | 4 | 16,000 | AZ-800T00(T) |
12/10/2024 | 12/13/2024 | 4 | 16,000 | AZ-800T00(T) |
1/14/2025 | 1/17/2025 | 4 | 16,000 | AZ-800T00(T) |
2/10/2025 | 2/14/2025 | 4 | 16,000 | AZ-800T00(T) |
3/11/2025 | 3/14/2025 | 4 | 16,000 | AZ-800T00(T) |
4/22/2025 | 4/25/2025 | 4 | 16,000 | AZ-800T00(T) |
5/13/2025 | 5/16/2025 | 4 | 16,000 | AZ-800T00(T) |
6/2/2025 | 6/6/2025 | 4 | 16,000 | AZ-800T00(T) |
7/15/2025 | 7/18/2025 | 4 | 16,000 | AZ-800T00(T) |
8/11/2025 | 8/15/2025 | 4 | 16,000 | AZ-800T00(T) |
9/9/2025 | 9/12/2025 | 4 | 16,000 | AZ-800T00(T) |
10/7/2025 | 10/10/2025 | 4 | 16,000 | AZ-800T00(T) |
11/11/2025 | 11/14/2025 | 4 | 16,000 | AZ-800T00(T) |
12/9/2025 | 12/12/2025 | 4 | 16,000 | AZ-800T00(T) |
10/28/2024 | 10/31/2024 | 4 | 16,000 | AZ-801T00 |
11/19/2024 | 11/22/2024 | 4 | 16,000 | AZ-801T00 |
12/17/2024 | 12/20/2024 | 4 | 16,000 | AZ-801T00 |
1/21/2025 | 1/24/2025 | 4 | 16,000 | AZ-801T00 |
2/18/2025 | 2/21/2025 | 4 | 16,000 | AZ-801T00 |
3/25/2025 | 3/28/2025 | 4 | 16,000 | AZ-801T00 |
4/22/2025 | 4/25/2025 | 4 | 16,000 | AZ-801T00 |
5/27/2025 | 5/30/2025 | 4 | 16,000 | AZ-801T00 |
6/17/2025 | 6/20/2025 | 4 | 16,000 | AZ-801T00 |
7/22/2025 | 7/25/2025 | 4 | 16,000 | AZ-801T00 |
8/19/2025 | 8/22/2025 | 4 | 16,000 | AZ-801T00 |
9/16/2025 | 9/19/2025 | 4 | 16,000 | AZ-801T00 |
10/27/2025 | 10/30/2025 | 4 | 16,000 | AZ-801T00 |
11/18/2025 | 11/21/2025 | 4 | 16,000 | AZ-801T00 |
12/16/2025 | 12/19/2025 | 4 | 16,000 | AZ-801T00 |
10/7/2024 | 10/7/2024 | 1 | 4,000 | AZ-900T00(T) |
11/11/2024 | 11/11/2024 | 1 | 4,000 | AZ-900T00(T) |
12/9/2024 | 12/9/2024 | 1 | 4,000 | AZ-900T00(T) |
1/6/2025 | 1/6/2025 | 1 | 4,000 | AZ-900T00(T) |
2/3/2025 | 2/3/2025 | 1 | 4,000 | AZ-900T00(T) |
3/7/2025 | 3/7/2025 | 1 | 4,000 | AZ-900T00(T) |
4/4/2025 | 4/4/2025 | 1 | 4,000 | AZ-900T00(T) |
5/5/2025 | 5/5/2025 | 1 | 4,000 | AZ-900T00(T) |
6/13/2025 | 6/13/2025 | 1 | 4,000 | AZ-900T00(T) |
7/9/2025 | 7/9/2025 | 1 | 4,000 | AZ-900T00(T) |
8/11/2025 | 8/11/2025 | 1 | 4,000 | AZ-900T00(T) |
9/8/2025 | 9/8/2025 | 1 | 4,000 | AZ-900T00(T) |
10/6/2025 | 10/6/2025 | 1 | 4,000 | AZ-900T00(T) |
11/7/2025 | 11/7/2025 | 1 | 4,000 | AZ-900T00(T) |
12/8/2025 | 12/8/2025 | 1 | 4,000 | AZ-900T00(T) |
1/6/2025 | 1/6/2025 | 1 | 4,000 | AI-3016 |
2/14/2025 | 2/14/2025 | 1 | 4,000 | AI-3016 |
3/7/2025 | 3/7/2025 | 1 | 4,000 | AI-3016 |
4/18/2025 | 4/18/2025 | 1 | 4,000 | AI-3016 |
5/2/2025 | 5/2/2025 | 1 | 4,000 | AI-3016 |
6/6/2025 | 6/6/2025 | 1 | 4,000 | AI-3016 |
7/14/2025 | 7/14/2025 | 1 | 4,000 | AI-3016 |
8/15/2025 | 8/15/2025 | 1 | 4,000 | AI-3016 |
9/12/2025 | 9/12/2025 | 1 | 4,000 | AI-3016 |
10/10/2025 | 10/10/2025 | 1 | 4,000 | AI-3016 |
11/7/2025 | 11/7/2025 | 1 | 4,000 | AI-3016 |
12/12/2025 | 12/12/2025 | 1 | 4,000 | AI-3016 |
10/8/2024 | 10/11/2024 | 4 | 16,000 | AI-102T00 |
11/12/2024 | 11/15/2024 | 4 | 16,000 | AI-102T00 |
12/10/2024 | 12/13/2024 | 4 | 16,000 | AI-102T00 |
1/14/2025 | 1/17/2025 | 4 | 16,000 | AI-102T00 |
2/10/2025 | 2/14/2025 | 4 | 16,000 | AI-102T00 |
3/18/2025 | 3/21/2025 | 4 | 16,000 | AI-102T00 |
4/8/2025 | 4/11/2025 | 4 | 16,000 | AI-102T00 |
5/13/2025 | 5/16/2025 | 4 | 16,000 | AI-102T00 |
6/2/2025 | 6/6/2025 | 4 | 16,000 | AI-102T00 |
7/15/2025 | 7/18/2025 | 4 | 16,000 | AI-102T00 |
8/11/2025 | 8/15/2025 | 4 | 16,000 | AI-102T00 |
9/9/2025 | 9/12/2025 | 4 | 16,000 | AI-102T00 |
10/7/2025 | 10/10/2025 | 4 | 16,000 | AI-102T00 |
11/11/2025 | 11/14/2025 | 4 | 16,000 | AI-102T00 |
12/9/2025 | 12/12/2025 | 4 | 16,000 | AI-102T00 |
10/7/2024 | 10/7/2024 | 1 | 4,000 | AI-900T00 |
11/4/2024 | 11/4/2024 | 1 | 4,000 | AI-900T00 |
12/9/2024 | 12/9/2024 | 1 | 4,000 | AI-900T00 |
1/6/2025 | 1/6/2025 | 1 | 4,000 | AI-900T00 |
2/3/2025 | 2/3/2025 | 1 | 4,000 | AI-900T00 |
3/7/2025 | 3/7/2025 | 1 | 4,000 | AI-900T00 |
4/11/2025 | 4/11/2025 | 1 | 4,000 | AI-900T00 |
5/2/2025 | 5/2/2025 | 1 | 4,000 | AI-900T00 |
6/13/2025 | 6/13/2025 | 1 | 4,000 | AI-900T00 |
7/9/2025 | 7/9/2025 | 1 | 4,000 | AI-900T00 |
8/11/2025 | 8/11/2025 | 1 | 4,000 | AI-900T00 |
9/5/2025 | 9/5/2025 | 1 | 4,000 | AI-900T00 |
10/6/2025 | 10/6/2025 | 1 | 4,000 | AI-900T00 |
11/3/2025 | 11/3/2025 | 1 | 4,000 | AI-900T00 |
12/8/2025 | 12/8/2025 | 1 | 4,000 | AI-900T00 |
10/7/2024 | 10/8/2024 | 2 | 8,000 | DP-080T00 |
11/14/2024 | 11/15/2024 | 2 | 8,000 | DP-080T00 |
12/9/2024 | 12/10/2024 | 2 | 8,000 | DP-080T00 |
1/9/2025 | 1/10/2025 | 2 | 8,000 | DP-080T00 |
2/13/2025 | 2/14/2025 | 2 | 8,000 | DP-080T00 |
3/3/2025 | 3/4/2025 | 2 | 8,000 | DP-080T00 |
4/17/2025 | 4/18/2025 | 2 | 8,000 | DP-080T00 |
5/8/2025 | 5/9/2025 | 2 | 8,000 | DP-080T00 |
6/5/2025 | 6/6/2025 | 2 | 8,000 | DP-080T00 |
7/8/2025 | 7/9/2025 | 2 | 8,000 | DP-080T00 |
8/7/2025 | 8/8/2025 | 2 | 8,000 | DP-080T00 |
9/8/2025 | 9/9/2025 | 2 | 8,000 | DP-080T00 |
10/6/2025 | 10/7/2025 | 2 | 8,000 | DP-080T00 |
11/6/2025 | 11/7/2025 | 2 | 8,000 | DP-080T00 |
12/11/2025 | 12/12/2025 | 2 | 8,000 | DP-080T00 |
10/2/2024 | 10/4/2024 | 3 | 12,000 | DP-100T01 |
11/6/2024 | 11/8/2024 | 3 | 12,000 | DP-100T01 |
12/11/2024 | 12/13/2024 | 3 | 12,000 | DP-100T01 |
1/8/2025 | 1/10/2025 | 3 | 12,000 | DP-100T01 |
2/10/2025 | 2/13/2025 | 3 | 12,000 | DP-100T01 |
3/5/2025 | 3/7/2025 | 3 | 12,000 | DP-100T01 |
4/2/2025 | 4/4/2025 | 3 | 12,000 | DP-100T01 |
5/7/2025 | 5/9/2025 | 3 | 12,000 | DP-100T01 |
6/4/2025 | 6/6/2025 | 3 | 12,000 | DP-100T01 |
7/7/2025 | 7/9/2025 | 3 | 12,000 | DP-100T01 |
8/6/2025 | 8/8/2025 | 3 | 12,000 | DP-100T01 |
9/3/2025 | 9/5/2025 | 3 | 12,000 | DP-100T01 |
10/1/2025 | 10/3/2025 | 3 | 12,000 | DP-100T01 |
11/5/2025 | 11/7/2025 | 3 | 12,000 | DP-100T01 |
12/10/2025 | 12/12/2025 | 3 | 12,000 | DP-100T01 |
10/28/2024 | 10/31/2024 | 4 | 16,000 | DP-300T00(T) |
11/19/2024 | 11/22/2024 | 4 | 16,000 | DP-300T00(T) |
12/17/2024 | 12/20/2024 | 4 | 16,000 | DP-300T00(T) |
1/21/2025 | 1/24/2025 | 4 | 16,000 | DP-300T00(T) |
2/10/2025 | 2/14/2025 | 4 | 16,000 | DP-300T00(T) |
3/25/2025 | 3/28/2025 | 4 | 16,000 | DP-300T00(T) |
4/22/2025 | 4/25/2025 | 4 | 16,000 | DP-300T00(T) |
5/20/2025 | 5/23/2025 | 4 | 16,000 | DP-300T00(T) |
6/17/2025 | 6/20/2025 | 4 | 16,000 | DP-300T00(T) |
7/22/2025 | 7/25/2025 | 4 | 16,000 | DP-300T00(T) |
8/19/2025 | 8/22/2025 | 4 | 16,000 | DP-300T00(T) |
9/16/2025 | 9/19/2025 | 4 | 16,000 | DP-300T00(T) |
10/27/2025 | 10/30/2025 | 4 | 16,000 | DP-300T00(T) |
11/25/2025 | 11/28/2025 | 4 | 16,000 | DP-300T00(T) |
12/16/2025 | 12/19/2025 | 4 | 16,000 | DP-300T00(T) |
10/15/2024 | 10/18/2024 | 4 | 16,000 | DP-420T00 |
11/12/2024 | 11/15/2024 | 4 | 16,000 | DP-420T00 |
12/17/2024 | 12/20/2024 | 4 | 16,000 | DP-420T00 |
1/21/2025 | 1/24/2025 | 4 | 16,000 | DP-420T00 |
2/10/2025 | 2/14/2025 | 4 | 16,000 | DP-420T00 |
3/25/2025 | 3/28/2025 | 4 | 16,000 | DP-420T00 |
4/22/2025 | 4/25/2025 | 4 | 16,000 | DP-420T00 |
5/20/2025 | 5/23/2025 | 4 | 16,000 | DP-420T00 |
6/17/2025 | 6/20/2025 | 4 | 16,000 | DP-420T00 |
7/15/2025 | 7/18/2025 | 4 | 16,000 | DP-420T00 |
8/19/2025 | 8/22/2025 | 4 | 16,000 | DP-420T00 |
9/16/2025 | 9/19/2025 | 4 | 16,000 | DP-420T00 |
10/14/2025 | 10/17/2025 | 4 | 16,000 | DP-420T00 |
11/11/2025 | 11/14/2025 | 4 | 16,000 | DP-420T00 |
12/16/2025 | 12/19/2025 | 4 | 16,000 | DP-420T00 |
5 | 20,000 | MD-100T00 | ||
5 | 20,000 | MD-101T00 | ||
10/7/2024 | 10/11/2024 | 5 | 20,000 | MD-102T00(T) |
11/25/2024 | 11/29/2024 | 5 | 20,000 | MD-102T00(T) |
12/23/2024 | 12/27/2024 | 5 | 20,000 | MD-102T00(T) |
1/20/2025 | 1/24/2025 | 5 | 20,000 | MD-102T00(T) |
2/17/2025 | 2/21/2025 | 5 | 20,000 | MD-102T00(T) |
3/24/2025 | 3/28/2025 | 5 | 20,000 | MD-102T00(T) |
4/21/2025 | 4/25/2025 | 5 | 20,000 | MD-102T00(T) |
5/26/2025 | 5/30/2025 | 5 | 20,000 | MD-102T00(T) |
6/23/2025 | 6/27/2025 | 5 | 20,000 | MD-102T00(T) |
7/14/2025 | 7/18/2025 | 5 | 20,000 | MD-102T00(T) |
8/25/2025 | 8/29/2025 | 5 | 20,000 | MD-102T00(T) |
9/22/2025 | 9/26/2025 | 5 | 20,000 | MD-102T00(T) |
10/6/2025 | 10/10/2025 | 5 | 20,000 | MD-102T00(T) |
11/24/2025 | 11/28/2025 | 5 | 20,000 | MD-102T00(T) |
12/22/2025 | 12/26/2025 | 5 | 20,000 | MD-102T00(T) |
5 | 20,000 | MS-100T00 | ||
5 | 20,000 | MS-101T00 | ||
10/7/2024 | 10/11/2024 | 5 | 20,000 | MS-102T00(T) |
11/25/2024 | 11/29/2024 | 5 | 20,000 | MS-102T00(T) |
12/23/2024 | 12/27/2024 | 5 | 20,000 | MS-102T00(T) |
1/20/2025 | 1/24/2025 | 5 | 20,000 | MS-102T00(T) |
2/17/2025 | 2/21/2025 | 5 | 20,000 | MS-102T00(T) |
3/24/2025 | 3/28/2025 | 5 | 20,000 | MS-102T00(T) |
4/21/2025 | 4/25/2025 | 5 | 20,000 | MS-102T00(T) |
5/26/2025 | 5/30/2025 | 5 | 20,000 | MS-102T00(T) |
6/23/2025 | 6/27/2025 | 5 | 20,000 | MS-102T00(T) |
7/14/2025 | 7/18/2025 | 5 | 20,000 | MS-102T00(T) |
8/25/2025 | 8/29/2025 | 5 | 20,000 | MS-102T00(T) |
9/22/2025 | 9/26/2025 | 5 | 20,000 | MS-102T00(T) |
10/6/2025 | 10/10/2025 | 5 | 20,000 | MS-102T00(T) |
11/24/2025 | 11/28/2025 | 5 | 20,000 | MS-102T00(T) |
12/22/2025 | 12/26/2025 | 5 | 20,000 | MS-102T00(T) |
6/10/2024 | 6/14/2024 | 5 | 20,000 | MS-203T00 |
3 | 20,000 | MS-220T00 | ||
6/11/2024 | 6/14/2024 | 4 | 16,000 | MS-600T00 |
10/15/2024 | 10/18/2024 | 4 | 16,000 | MS-700T00(T) |
11/12/2024 | 11/15/2024 | 4 | 16,000 | MS-700T00(T) |
12/10/2024 | 12/13/2024 | 4 | 16,000 | MS-700T00(T) |
1/14/2025 | 1/17/2025 | 4 | 16,000 | MS-700T00(T) |
2/10/2025 | 2/14/2025 | 4 | 16,000 | MS-700T00(T) |
3/11/2025 | 3/14/2025 | 4 | 16,000 | MS-700T00(T) |
4/22/2025 | 4/25/2025 | 4 | 16,000 | MS-700T00(T) |
5/6/2025 | 5/9/2025 | 4 | 16,000 | MS-700T00(T) |
6/2/2025 | 6/6/2025 | 4 | 16,000 | MS-700T00(T) |
7/22/2025 | 7/25/2025 | 4 | 16,000 | MS-700T00(T) |
8/5/2025 | 8/8/2025 | 4 | 16,000 | MS-700T00(T) |
9/9/2025 | 9/12/2025 | 4 | 16,000 | MS-700T00(T) |
10/14/2025 | 10/17/2025 | 4 | 16,000 | MS-700T00(T) |
11/11/2025 | 11/14/2025 | 4 | 16,000 | MS-700T00(T) |
12/9/2025 | 12/12/2025 | 4 | 16,000 | MS-700T00(T) |
3 | 12,000 | MS-720T00 | ||
10/8/2024 | 10/11/2024 | 4 | 16,000 | MS-721T00 |
11/12/2024 | 11/15/2024 | 4 | 16,000 | MS-721T00 |
12/10/2024 | 12/13/2024 | 4 | 16,000 | MS-721T00 |
1/14/2025 | 1/17/2025 | 4 | 16,000 | MS-721T00 |
2/10/2025 | 2/14/2025 | 4 | 16,000 | MS-721T00 |
3/11/2025 | 3/14/2025 | 4 | 16,000 | MS-721T00 |
4/1/2025 | 4/4/2025 | 4 | 16,000 | MS-721T00 |
5/6/2025 | 5/9/2025 | 4 | 16,000 | MS-721T00 |
6/17/2025 | 6/20/2025 | 4 | 16,000 | MS-721T00 |
7/22/2025 | 7/25/2025 | 4 | 16,000 | MS-721T00 |
8/5/2025 | 8/8/2025 | 4 | 16,000 | MS-721T00 |
9/9/2025 | 9/12/2025 | 4 | 16,000 | MS-721T00 |
10/7/2025 | 10/10/2025 | 4 | 16,000 | MS-721T00 |
11/11/2025 | 11/14/2025 | 4 | 16,000 | MS-721T00 |
12/9/2025 | 12/12/2025 | 4 | 16,000 | MS-721T00 |
3 | 12,000 | MS-740T00 | ||
10/21/2024 | 10/21/2024 | 1 | 4,000 | MS-080T00 |
11/18/2024 | 11/18/2024 | 1 | 4,000 | MS-080T00 |
12/6/2024 | 12/6/2024 | 1 | 4,000 | MS-080T00 |
1/27/2025 | 1/27/2025 | 1 | 4,000 | MS-080T00 |
2/10/2025 | 2/10/2025 | 1 | 4,000 | MS-080T00 |
3/21/2025 | 3/21/2025 | 1 | 4,000 | MS-080T00 |
4/11/2025 | 4/11/2025 | 1 | 4,000 | MS-080T00 |
5/19/2025 | 5/19/2025 | 1 | 4,000 | MS-080T00 |
6/13/2025 | 6/13/2025 | 1 | 4,000 | MS-080T00 |
7/14/2025 | 7/14/2025 | 1 | 4,000 | MS-080T00 |
8/15/2025 | 8/15/2025 | 1 | 4,000 | MS-080T00 |
9/15/2025 | 9/15/2025 | 1 | 4,000 | MS-080T00 |
10/20/2025 | 10/20/2025 | 1 | 4,000 | MS-080T00 |
11/17/2025 | 11/17/2025 | 1 | 4,000 | MS-080T00 |
12/4/2025 | 12/4/2025 | 1 | 4,000 | MS-080T00 |
10/21/2024 | 10/21/2024 | 1 | 4,000 | MS-900T01 |
11/18/2024 | 11/18/2024 | 1 | 4,000 | MS-900T01 |
12/6/2024 | 12/6/2024 | 1 | 4,000 | MS-900T01 |
1/27/2025 | 1/27/2025 | 1 | 4,000 | MS-900T01 |
2/10/2025 | 2/10/2025 | 1 | 4,000 | MS-900T01 |
3/21/2025 | 3/21/2025 | 1 | 4,000 | MS-900T01 |
4/11/2025 | 4/11/2025 | 1 | 4,000 | MS-900T01 |
5/19/2025 | 5/19/2025 | 1 | 4,000 | MS-900T01 |
6/13/2025 | 6/13/2025 | 1 | 4,000 | MS-900T01 |
7/14/2025 | 7/14/2025 | 1 | 4,000 | MS-900T01 |
8/15/2025 | 8/15/2025 | 1 | 4,000 | MS-900T01 |
9/15/2025 | 9/15/2025 | 1 | 4,000 | MS-900T01 |
10/20/2025 | 10/20/2025 | 1 | 4,000 | MS-900T01 |
11/17/2025 | 11/17/2025 | 1 | 4,000 | MS-900T01 |
12/4/2025 | 12/4/2025 | 1 | 4,000 | MS-900T01 |
10/7/2024 | 10/11/2024 | 5 | 20,000 | 55354A(T) |
11/18/2024 | 11/22/2024 | 5 | 20,000 | 55354A(T) |
12/16/2024 | 12/20/2024 | 5 | 20,000 | 55354A(T) |
1/13/2025 | 1/17/2025 | 5 | 20,000 | 55354A(T) |
2/3/2025 | 2/7/2025 | 5 | 20,000 | 55354A(T) |
3/17/2025 | 3/21/2025 | 5 | 20,000 | 55354A(T) |
4/21/2025 | 4/25/2025 | 5 | 20,000 | 55354A(T) |
5/26/2025 | 5/30/2025 | 5 | 20,000 | 55354A(T) |
6/9/2025 | 6/13/2025 | 5 | 20,000 | 55354A(T) |
7/14/2025 | 7/18/2025 | 5 | 20,000 | 55354A(T) |
8/18/2025 | 8/22/2025 | 5 | 20,000 | 55354A(T) |
9/15/2025 | 9/19/2025 | 5 | 20,000 | 55354A(T) |
10/6/2025 | 10/10/2025 | 5 | 20,000 | 55354A(T) |
11/17/2025 | 11/21/2025 | 5 | 20,000 | 55354A(T) |
12/15/2025 | 12/19/2025 | 5 | 20,000 | 55354A(T) |
11/12/2024 | 11/15/2024 | 4 | 16,000 | AZ-500T00(T) |
12/10/2024 | 12/13/2024 | 4 | 16,000 | AZ-500T00(T) |
1/14/2025 | 1/17/2025 | 4 | 16,000 | AZ-500T00(T) |
2/10/2025 | 2/13/2025 | 4 | 16,000 | AZ-500T00(T) |
3/11/2025 | 3/14/2025 | 4 | 16,000 | AZ-500T00(T) |
4/29/2025 | 5/1/2025 | 4 | 16,000 | AZ-500T00(T) |
5/13/2025 | 5/16/2025 | 4 | 16,000 | AZ-500T00(T) |
6/2/2025 | 6/6/2025 | 4 | 16,000 | AZ-500T00(T) |
7/15/2025 | 7/18/2025 | 4 | 16,000 | AZ-500T00(T) |
8/5/2025 | 8/8/2025 | 4 | 16,000 | AZ-500T00(T) |
9/9/2025 | 9/12/2025 | 4 | 16,000 | AZ-500T00(T) |
10/7/2025 | 10/10/2025 | 4 | 16,000 | AZ-500T00(T) |
11/11/2025 | 11/14/2025 | 4 | 16,000 | AZ-500T00(T) |
12/9/2025 | 12/12/2025 | 4 | 16,000 | AZ-500T00(T) |
4 | 16,000 | MS-500T00 | ||
10/8/2024 | 10/11/2024 | 4 | 16,000 | SC-100T00(T) |
11/12/2024 | 11/15/2024 | 4 | 16,000 | SC-100T00(T) |
12/10/2024 | 12/13/2024 | 4 | 16,000 | SC-100T00(T) |
1/14/2025 | 1/17/2025 | 4 | 16,000 | SC-100T00(T) |
2/10/2025 | 2/13/2025 | 4 | 16,000 | SC-100T00(T) |
3/11/2025 | 3/14/2025 | 4 | 16,000 | SC-100T00(T) |
4/29/2025 | 5/1/2025 | 4 | 16,000 | SC-100T00(T) |
5/13/2025 | 5/16/2025 | 4 | 16,000 | SC-100T00(T) |
6/2/2025 | 6/6/2025 | 4 | 16,000 | SC-100T00(T) |
7/15/2025 | 7/18/2025 | 4 | 16,000 | SC-100T00(T) |
8/5/2025 | 8/8/2025 | 4 | 16,000 | SC-100T00(T) |
9/9/2025 | 9/12/2025 | 4 | 16,000 | SC-100T00(T) |
10/7/2025 | 10/10/2025 | 4 | 16,000 | SC-100T00(T) |
11/11/2025 | 11/14/2025 | 4 | 16,000 | SC-100T00(T) |
12/9/2025 | 12/12/2025 | 4 | 16,000 | SC-100T00(T) |
6/4/2024 | 6/7/2024 | 4 | 16,000 | SC-200T00(T) |
7/16/2024 | 7/19/2024 | 4 | 16,000 | SC-200T00(T) |
8/13/2024 | 8/16/2024 | 4 | 16,000 | SC-200T00(T) |
9/10/2024 | 9/13/2024 | 4 | 16,000 | SC-200T00(T) |
10/8/2024 | 10/11/2024 | 4 | 16,000 | SC-200T00(T) |
11/12/2024 | 11/15/2024 | 4 | 16,000 | SC-200T00(T) |
12/10/2024 | 12/13/2024 | 4 | 16,000 | SC-200T00(T) |
1/14/2025 | 1/17/2025 | 4 | 16,000 | SC-200T00(T) |
2/10/2025 | 2/13/2025 | 4 | 16,000 | SC-200T00(T) |
3/11/2025 | 3/14/2025 | 4 | 16,000 | SC-200T00(T) |
4/29/2025 | 5/1/2025 | 4 | 16,000 | SC-200T00(T) |
5/13/2025 | 5/16/2025 | 4 | 16,000 | SC-200T00(T) |
6/2/2025 | 6/6/2025 | 4 | 16,000 | SC-200T00(T) |
7/15/2025 | 7/18/2025 | 4 | 16,000 | SC-200T00(T) |
8/5/2025 | 8/8/2025 | 4 | 16,000 | SC-200T00(T) |
9/9/2025 | 9/12/2025 | 4 | 16,000 | SC-200T00(T) |
10/7/2025 | 10/10/2025 | 4 | 16,000 | SC-200T00(T) |
11/11/2025 | 11/14/2025 | 4 | 16,000 | SC-200T00(T) |
12/9/2025 | 12/12/2025 | 4 | 16,000 | SC-200T00(T) |
10/28/2024 | 10/31/2024 | 4 | 16,000 | SC-300T00(T) |
11/19/2024 | 11/22/2024 | 4 | 16,000 | SC-300T00(T) |
12/17/2024 | 12/20/2024 | 4 | 16,000 | SC-300T00(T) |
1/21/2025 | 1/24/2025 | 4 | 16,000 | SC-300T00(T) |
2/18/2025 | 2/21/2025 | 4 | 16,000 | SC-300T00(T) |
3/18/2025 | 3/21/2025 | 4 | 16,000 | SC-300T00(T) |
4/29/2025 | 5/1/2025 | 4 | 16,000 | SC-300T00(T) |
5/20/2025 | 5/23/2025 | 4 | 16,000 | SC-300T00(T) |
6/17/2025 | 6/20/2025 | 4 | 16,000 | SC-300T00(T) |
7/22/2025 | 7/25/2025 | 4 | 16,000 | SC-300T00(T) |
8/19/2025 | 8/22/2025 | 4 | 16,000 | SC-300T00(T) |
9/16/2025 | 9/19/2025 | 4 | 16,000 | SC-300T00(T) |
10/27/2025 | 10/30/2025 | 4 | 16,000 | SC-300T00(T) |
11/18/2025 | 11/21/2025 | 4 | 16,000 | SC-300T00(T) |
12/16/2025 | 12/19/2025 | 4 | 16,000 | SC-300T00(T) |
10/28/2024 | 10/31/2024 | 4 | 16,000 | SC-400T00(T) |
11/19/2024 | 11/22/2024 | 4 | 16,000 | SC-400T00(T) |
12/10/2024 | 12/13/2024 | 4 | 16,000 | SC-400T00(T) |
1/14/2025 | 1/17/2025 | 4 | 16,000 | SC-400T00(T) |
2/10/2025 | 2/14/2025 | 4 | 16,000 | SC-400T00(T) |
3/18/2025 | 3/21/2025 | 4 | 16,000 | SC-400T00(T) |
4/8/2025 | 4/11/2025 | 4 | 16,000 | SC-400T00(T) |
5/14/2025 | 5/17/2025 | 4 | 16,000 | SC-400T00(T) |
6/17/2025 | 6/20/2025 | 4 | 16,000 | SC-400T00(T) |
7/15/2025 | 7/18/2025 | 4 | 16,000 | SC-400T00(T) |
8/26/2025 | 8/29/2025 | 4 | 16,000 | SC-400T00(T) |
9/23/2025 | 9/26/2025 | 4 | 16,000 | SC-400T00(T) |
10/27/2025 | 10/30/2025 | 4 | 16,000 | SC-400T00(T) |
11/18/2025 | 11/21/2025 | 4 | 16,000 | SC-400T00(T) |
12/9/2025 | 12/12/2025 | 4 | 16,000 | SC-400T00(T) |
10/7/2024 | 10/7/2024 | 1 | 4,000 | SC-900T00(T) |
11/11/2024 | 11/11/2024 | 1 | 4,000 | SC-900T00(T) |
12/4/2024 | 12/4/2024 | 1 | 4,000 | SC-900T00(T) |
1/6/2025 | 1/6/2025 | 1 | 4,000 | SC-900T00(T) |
2/14/2025 | 2/14/2025 | 1 | 4,000 | SC-900T00(T) |
3/14/2025 | 3/14/2025 | 1 | 4,000 | SC-900T00(T) |
4/18/2025 | 4/18/2025 | 1 | 4,000 | SC-900T00(T) |
5/2/2025 | 5/2/2025 | 1 | 4,000 | SC-900T00(T) |
6/30/2025 | 6/30/2025 | 1 | 4,000 | SC-900T00(T) |
7/29/2025 | 7/29/2025 | 1 | 4,000 | SC-900T00(T) |
8/11/2025 | 8/11/2025 | 1 | 4,000 | SC-900T00(T) |
9/8/2025 | 9/8/2025 | 1 | 4,000 | SC-900T00(T) |
10/6/2025 | 10/6/2025 | 1 | 4,000 | SC-900T00(T) |
11/10/2025 | 11/10/2025 | 1 | 4,000 | SC-900T00(T) |
12/4/2025 | 12/4/2025 | 1 | 4,000 | SC-900T00(T) |
16,000 | PL-100T00(T) | |||
10/15/2024 | 10/18/2024 | 4 | 16,000 | PL-200T00(T) |
11/12/2024 | 11/15/2024 | 4 | 16,000 | PL-200T00(T) |
12/17/2024 | 12/20/2024 | 4 | 16,000 | PL-200T00(T) |
1/14/2025 | 1/17/2025 | 4 | 16,000 | PL-200T00(T) |
2/10/2025 | 2/14/2025 | 4 | 16,000 | PL-200T00(T) |
3/11/2025 | 3/14/2025 | 4 | 16,000 | PL-200T00(T) |
4/22/2025 | 4/25/2025 | 4 | 16,000 | PL-200T00(T) |
5/13/2025 | 5/16/2025 | 4 | 16,000 | PL-200T00(T) |
6/17/2025 | 6/20/2025 | 4 | 16,000 | PL-200T00(T) |
7/15/2025 | 7/18/2025 | 4 | 16,000 | PL-200T00(T) |
8/11/2025 | 8/15/2025 | 4 | 16,000 | PL-200T00(T) |
9/9/2025 | 9/12/2025 | 4 | 16,000 | PL-200T00(T) |
10/14/2025 | 10/17/2025 | 4 | 16,000 | PL-200T00(T) |
11/11/2025 | 11/14/2025 | 4 | 16,000 | PL-200T00(T) |
12/23/2025 | 12/26/2025 | 4 | 16,000 | PL-200T00(T) |
10/15/2024 | 10/17/2024 | 3 | 12,000 | PL-300T00(T) |
11/4/2024 | 11/6/2024 | 3 | 12,000 | PL-300T00(T) |
12/11/2024 | 12/13/2024 | 3 | 12,000 | PL-300T00(T) |
1/15/2025 | 1/17/2025 | 3 | 12,000 | PL-300T00(T) |
2/10/2025 | 2/13/2025 | 3 | 12,000 | PL-300T00(T) |
3/10/2025 | 3/12/2025 | 3 | 12,000 | PL-300T00(T) |
4/28/2025 | 4/30/2025 | 3 | 12,000 | PL-300T00(T) |
5/7/2025 | 5/9/2025 | 3 | 12,000 | PL-300T00(T) |
6/9/2025 | 6/11/2025 | 3 | 12,000 | PL-300T00(T) |
7/14/2025 | 7/16/2025 | 3 | 12,000 | PL-300T00(T) |
8/13/2025 | 8/15/2025 | 3 | 12,000 | PL-300T00(T) |
9/10/2025 | 9/12/2025 | 3 | 12,000 | PL-300T00(T) |
10/14/2025 | 10/16/2025 | 3 | 12,000 | PL-300T00(T) |
11/12/2025 | 11/14/2025 | 3 | 12,000 | PL-300T00(T) |
12/10/2025 | 12/12/2025 | 3 | 12,000 | PL-300T00(T) |
10/7/2024 | 10/11/2024 | 5 | 20,000 | PL-400T00(T) |
11/18/2024 | 11/22/2024 | 5 | 20,000 | PL-400T00(T) |
12/16/2024 | 12/20/2024 | 5 | 20,000 | PL-400T00(T) |
1/14/2025 | 1/17/2025 | 5 | 20,000 | PL-400T00(T) |
2/17/2025 | 2/21/2025 | 5 | 20,000 | PL-400T00(T) |
3/17/2025 | 3/21/2025 | 5 | 20,000 | PL-400T00(T) |
4/21/2025 | 4/25/2025 | 5 | 20,000 | PL-400T00(T) |
5/19/2025 | 5/23/2025 | 5 | 20,000 | PL-400T00(T) |
6/23/2025 | 6/27/2025 | 5 | 20,000 | PL-400T00(T) |
7/14/2025 | 7/18/2025 | 5 | 20,000 | PL-400T00(T) |
8/18/2025 | 8/22/2025 | 5 | 20,000 | PL-400T00(T) |
9/15/2025 | 9/19/2025 | 5 | 20,000 | PL-400T00(T) |
10/6/2025 | 10/10/2025 | 5 | 20,000 | PL-400T00(T) |
11/17/2025 | 11/21/2025 | 5 | 20,000 | PL-400T00(T) |
12/15/2025 | 12/19/2025 | 5 | 20,000 | PL-400T00(T) |
10/15/2024 | 10/18/2024 | 4 | 16,000 | PL-500T00(T) |
11/19/2024 | 11/22/2024 | 4 | 16,000 | PL-500T00(T) |
12/17/2024 | 12/20/2024 | 4 | 16,000 | PL-500T00(T) |
1/21/2025 | 1/24/2025 | 4 | 16,000 | PL-500T00(T) |
2/10/2025 | 2/14/2025 | 4 | 16,000 | PL-500T00(T) |
3/18/2025 | 3/21/2025 | 4 | 16,000 | PL-500T00(T) |
4/22/2025 | 4/25/2025 | 4 | 16,000 | PL-500T00(T) |
5/27/2025 | 5/30/2025 | 4 | 16,000 | PL-500T00(T) |
6/16/2025 | 6/20/2025 | 4 | 16,000 | PL-500T00(T) |
7/29/2025 | 8/1/2025 | 4 | 16,000 | PL-500T00(T) |
8/19/2025 | 8/22/2025 | 4 | 16,000 | PL-500T00(T) |
9/9/2025 | 9/12/2025 | 4 | 16,000 | PL-500T00(T) |
10/14/2025 | 10/17/2025 | 4 | 16,000 | PL-500T00(T) |
11/18/2025 | 11/21/2025 | 4 | 16,000 | PL-500T00(T) |
12/16/2025 | 12/19/2025 | 4 | 16,000 | PL-500T00(T) |
10/28/2024 | 10/30/2024 | 3 | 12,000 | PL-600T00(T) |
11/20/2024 | 11/22/2024 | 3 | 12,000 | PL-600T00(T) |
12/23/2024 | 12/25/2024 | 3 | 12,000 | PL-600T00(T) |
1/20/2025 | 1/22/2025 | 3 | 12,000 | PL-600T00(T) |
2/11/2025 | 2/14/2025 | 3 | 12,000 | PL-600T00(T) |
3/10/2025 | 3/12/2025 | 3 | 12,000 | PL-600T00(T) |
4/9/2025 | 4/11/2025 | 3 | 12,000 | PL-600T00(T) |
5/14/2025 | 5/16/2025 | 3 | 12,000 | PL-600T00(T) |
6/16/2025 | 6/18/2025 | 3 | 12,000 | PL-600T00(T) |
7/29/2025 | 7/31/2025 | 3 | 12,000 | PL-600T00(T) |
8/27/2025 | 8/29/2025 | 3 | 12,000 | PL-600T00(T) |
9/22/2025 | 9/24/2025 | 3 | 12,000 | PL-600T00(T) |
10/20/2025 | 10/22/2025 | 3 | 12,000 | PL-600T00(T) |
11/19/2025 | 11/21/2025 | 3 | 12,000 | PL-600T00(T) |
12/24/2025 | 12/26/2025 | 3 | 12,000 | PL-600T00(T) |
10/7/2024 | 10/7/2024 | 1 | 4,000 | PL-900T00(T) |
11/4/2024 | 11/4/2024 | 1 | 4,000 | PL-900T00(T) |
12/9/2024 | 12/9/2024 | 1 | 4,000 | PL-900T00(T) |
1/6/2025 | 1/6/2025 | 1 | 4,000 | PL-900T00(T) |
2/10/2025 | 2/10/2025 | 1 | 4,000 | PL-900T00(T) |
3/3/2025 | 3/3/2025 | 1 | 4,000 | PL-900T00(T) |
4/18/2025 | 4/18/2025 | 1 | 4,000 | PL-900T00(T) |
5/9/2025 | 5/9/2025 | 1 | 4,000 | PL-900T00(T) |
6/9/2025 | 6/9/2025 | 1 | 4,000 | PL-900T00(T) |
7/9/2025 | 7/9/2025 | 1 | 4,000 | PL-900T00(T) |
8/11/2025 | 8/11/2025 | 1 | 4,000 | PL-900T00(T) |
9/5/2025 | 9/5/2025 | 1 | 4,000 | PL-900T00(T) |
10/6/2025 | 10/6/2025 | 1 | 4,000 | PL-900T00(T) |
11/3/2025 | 11/3/2025 | 1 | 4,000 | PL-900T00(T) |
12/8/2025 | 12/8/2025 | 1 | 4,000 | PL-900T00(T) |
10/28/2024 | 10/31/2024 | 4 | 10,000 | PWA-201(T) |
11/25/2024 | 11/28/2024 | 4 | 10,000 | PWA-201(T) |
12/23/2024 | 12/26/2024 | 4 | 10,000 | PWA-201(T) |
1/20/2025 | 1/23/2025 | 4 | 10,000 | PWA-201(T) |
2/18/2025 | 2/21/2025 | 4 | 10,000 | PWA-201(T) |
3/25/2025 | 3/28/2025 | 4 | 10,000 | PWA-201(T) |
4/22/2025 | 4/25/2025 | 4 | 10,000 | PWA-201(T) |
5/6/2025 | 5/9/2025 | 4 | 10,000 | PWA-201(T) |
6/23/2025 | 6/26/2025 | 4 | 10,000 | PWA-201(T) |
7/1/2025 | 7/4/2025 | 4 | 10,000 | PWA-201(T) |
8/25/2025 | 8/28/2025 | 4 | 10,000 | PWA-201(T) |
9/22/2025 | 9/25/2025 | 4 | 10,000 | PWA-201(T) |
10/20/2025 | 10/24/2025 | 4 | 10,000 | PWA-201(T) |
11/25/2025 | 11/28/2025 | 4 | 10,000 | PWA-201(T) |
12/22/2025 | 12/25/2025 | 4 | 10,000 | PWA-201(T) |
11/6/2024 | 11/7/2024 | 2 | 5,000 | M365-End-User(T) |
12/2/2024 | 12/3/2024 | 2 | 5,000 | M365-End-User(T) |
1/6/2025 | 1/7/2025 | 2 | 5,000 | M365-End-User(T) |
2/3/2025 | 2/4/2025 | 2 | 5,000 | M365-End-User(T) |
3/3/2025 | 3/4/2025 | 2 | 5,000 | M365-End-User(T) |
4/17/2025 | 4/18/2025 | 2 | 5,000 | M365-End-User(T) |
5/6/2025 | 5/7/2025 | 2 | 5,000 | M365-End-User(T) |
6/12/2025 | 6/13/2025 | 2 | 5,000 | M365-End-User(T) |
7/8/2025 | 7/9/2025 | 2 | 5,000 | M365-End-User(T) |
8/7/2025 | 8/8/2025 | 2 | 5,000 | M365-End-User(T) |
9/1/2025 | 9/2/2025 | 2 | 5,000 | M365-End-User(T) |
9/30/2025 | 10/1/2025 | 2 | 5,000 | M365-End-User(T) |
11/6/2025 | 11/7/2025 | 2 | 5,000 | M365-End-User(T) |
12/1/2025 | 12/2/2025 | 2 | 5,000 | M365-End-User(T) |
1 | 2,500 | O365-Admin-ENH | ||
10/18/2024 | 10/18/2024 | 1 | 4,000 | 55154B(T) |
11/11/2024 | 11/11/2024 | 1 | 4,000 | 55154B(T) |
12/9/2024 | 12/9/2024 | 1 | 4,000 | 55154B(T) |
1/20/2025 | 1/20/2025 | 1 | 4,000 | 55154B(T) |
2/14/2025 | 2/14/2025 | 1 | 4,000 | 55154B(T) |
3/14/2025 | 3/14/2025 | 1 | 4,000 | 55154B(T) |
4/18/2025 | 4/18/2025 | 1 | 4,000 | 55154B(T) |
5/9/2025 | 5/9/2025 | 1 | 4,000 | 55154B(T) |
6/9/2025 | 6/9/2025 | 1 | 4,000 | 55154B(T) |
7/14/2025 | 7/14/2025 | 1 | 4,000 | 55154B(T) |
8/15/2025 | 8/15/2025 | 1 | 4,000 | 55154B(T) |
9/15/2025 | 9/15/2025 | 1 | 4,000 | 55154B(T) |
10/17/2025 | 10/17/2025 | 1 | 4,000 | 55154B(T) |
11/10/2025 | 11/10/2025 | 1 | 4,000 | 55154B(T) |
12/8/2025 | 12/8/2025 | 1 | 4,000 | 55154B(T) |
6/10/2024 | 6/10/2024 | 1 | 2,500 | OF-19-NF |
6/13/2024 | 6/14/2024 | 2 | 5,000 | WD-19-01 |
6/20/2024 | 6/21/2024 | 2 | 5,000 | WD-19-02 |
6/13/2024 | 6/14/2024 | 2 | 5,000 | EX-19-01 |
6/20/2024 | 6/21/2024 | 2 | 5,000 | EX-19-02 |
6/24/2024 | 6/24/2024 | 1 | 2,500 | EX-19-03 |
6/11/2024 | 6/12/2024 | 2 | 5,000 | PP-19-01 |
6/13/2024 | 6/14/2024 | 2 | 5,000 | PP-19-02 |
6/5/2024 | 6/7/2024 | 3 | 7,500 | PJ-19-US |
6/24/2024 | 6/24/2024 | 1 | 2,500 | OL-19-01 |
10/7/2024 | 10/11/2024 | 5 | 12,500 | ASP-MVC5 |
11/25/2024 | 11/29/2024 | 5 | 12,500 | ASP-MVC5 |
12/23/2024 | 12/27/2024 | 5 | 12,500 | ASP-MVC5 |
1/20/2025 | 1/24/2025 | 5 | 12,500 | ASP-MVC5 |
2/17/2025 | 2/21/2025 | 5 | 12,500 | ASP-MVC5 |
3/17/2025 | 3/21/2025 | 5 | 12,500 | ASP-MVC5 |
4/21/2025 | 4/25/2025 | 5 | 12,500 | ASP-MVC5 |
5/26/2025 | 5/30/2025 | 5 | 12,500 | ASP-MVC5 |
6/23/2025 | 6/27/2025 | 5 | 12,500 | ASP-MVC5 |
7/14/2025 | 7/18/2025 | 5 | 12,500 | ASP-MVC5 |
8/25/2025 | 8/29/2025 | 5 | 12,500 | ASP-MVC5 |
9/22/2025 | 9/26/2025 | 5 | 12,500 | ASP-MVC5 |
10/6/2025 | 10/10/2025 | 5 | 12,500 | ASP-MVC5 |
11/24/2025 | 11/28/2025 | 5 | 12,500 | ASP-MVC5 |
12/22/2025 | 12/26/2025 | 5 | 12,500 | ASP-MVC5 |
10/24/2024 | 10/25/2024 | 2 | 8,000 | 55267-A |
11/18/2024 | 11/19/2024 | 2 | 8,000 | 55267-A |
12/19/2024 | 12/20/2024 | 2 | 8,000 | 55267-A |
1/27/2025 | 1/28/2025 | 2 | 8,000 | 55267-A |
2/27/2025 | 2/28/2025 | 2 | 8,000 | 55267-A |
3/27/2025 | 3/28/2025 | 2 | 8,000 | 55267-A |
4/17/2025 | 4/18/2025 | 2 | 8,000 | 55267-A |
5/26/2025 | 5/27/2025 | 2 | 8,000 | 55267-A |
6/26/2025 | 6/27/2025 | 2 | 8,000 | 55267-A |
7/30/2025 | 7/31/2025 | 2 | 8,000 | 55267-A |
8/18/2025 | 8/19/2025 | 2 | 8,000 | 55267-A |
9/15/2025 | 9/16/2025 | 2 | 8,000 | 55267-A |
10/21/2025 | 10/22/2025 | 2 | 8,000 | 55267-A |
11/3/2025 | 11/4/2025 | 2 | 8,000 | 55267-A |
12/18/2025 | 12/19/2025 | 2 | 8,000 | 55267-A |
11/7/2024 | 11/15/2024 | 4 | 16,000 | 55284-A |
12/10/2024 | 12/13/2024 | 4 | 16,000 | 55284-A |
1/14/2025 | 1/17/2025 | 4 | 16,000 | 55284-A |
2/10/2025 | 2/14/2025 | 4 | 16,000 | 55284-A |
3/11/2024 | 3/14/2024 | 4 | 16,000 | 55284-A |
4/1/2025 | 4/4/2025 | 4 | 16,000 | 55284-A |
5/13/2025 | 5/16/2025 | 4 | 16,000 | 55284-A |
6/2/2025 | 6/6/2025 | 4 | 16,000 | 55284-A |
7/15/2025 | 7/18/2025 | 4 | 16,000 | 55284-A |
8/19/2025 | 8/22/2025 | 4 | 16,000 | 55284-A |
9/9/2025 | 9/12/2025 | 4 | 16,000 | 55284-A |
10/14/2025 | 10/17/2025 | 4 | 16,000 | 55284-A |
11/10/2025 | 11/13/2025 | 4 | 16,000 | 55284-A |
12/9/2025 | 12/12/2025 | 4 | 16,000 | 55284-A |
10/21/2024 | 10/22/2024 | 2 | 8,000 | 55285-A |
11/21/2024 | 11/22/2024 | 2 | 8,000 | 55285-A |
12/19/2024 | 12/20/2024 | 2 | 8,000 | 55285-A |
1/23/2025 | 1/24/2025 | 2 | 8,000 | 55285-A |
2/24/2025 | 2/25/2025 | 2 | 8,000 | 55285-A |
3/24/2025 | 3/25/2025 | 2 | 8,000 | 55285-A |
4/17/2025 | 4/18/2025 | 2 | 8,000 | 55285-A |
5/23/2025 | 5/24/2025 | 2 | 8,000 | 55285-A |
6/12/2025 | 6/13/2025 | 2 | 8,000 | 55285-A |
7/30/2025 | 7/31/2025 | 2 | 8,000 | 55285-A |
8/18/2025 | 8/19/2025 | 2 | 8,000 | 55285-A |
9/15/2025 | 9/16/2025 | 2 | 8,000 | 55285-A |
10/20/2025 | 10/21/2025 | 2 | 8,000 | 55285-A |
11/6/2025 | 11/7/2025 | 2 | 8,000 | 55285-A |
12/18/2025 | 12/19/2025 | 2 | 8,000 | 55285-A |
10/7/2024 | 10/11/2024 | 5 | 20,000 | 55039-B |
11/25/2024 | 11/29/2024 | 5 | 20,000 | 55039-B |
12/23/2024 | 12/27/2024 | 5 | 20,000 | 55039-B |
1/13/2025 | 1/17/2025 | 5 | 20,000 | 55039-B |
2/24/2025 | 2/28/2025 | 5 | 20,000 | 55039-B |
3/24/2025 | 3/28/2025 | 5 | 20,000 | 55039-B |
4/21/2025 | 4/25/2025 | 5 | 20,000 | 55039-B |
5/26/2025 | 5/30/2025 | 5 | 20,000 | 55039-B |
6/23/2025 | 6/27/2025 | 5 | 20,000 | 55039-B |
7/14/2025 | 7/18/2025 | 5 | 20,000 | 55039-B |
8/25/2025 | 8/29/2025 | 5 | 20,000 | 55039-B |
9/22/2025 | 9/26/2025 | 5 | 20,000 | 55039-B |
10/6/2025 | 10/10/2025 | 5 | 20,000 | 55039-B |
11/24/2025 | 11/28/2025 | 5 | 20,000 | 55039-B |
12/22/2025 | 12/26/2025 | 5 | 20,000 | 55039-B |
10/21/2024 | 10/25/2024 | 4 | 16,000 | 55215-B(T) |
11/12/2024 | 11/15/2024 | 4 | 16,000 | 55215-B(T) |
12/10/2024 | 12/13/2024 | 4 | 16,000 | 55215-B(T) |
1/14/2025 | 1/17/2025 | 4 | 16,000 | 55215-B(T) |
2/10/2025 | 2/14/2025 | 4 | 16,000 | 55215-B(T) |
3/10/2025 | 3/13/2025 | 4 | 16,000 | 55215-B(T) |
4/1/2025 | 4/4/2025 | 4 | 16,000 | 55215-B(T) |
5/13/2025 | 5/16/2025 | 4 | 16,000 | 55215-B(T) |
6/17/2025 | 6/20/2025 | 4 | 16,000 | 55215-B(T) |
7/15/2025 | 7/18/2025 | 4 | 16,000 | 55215-B(T) |
8/11/2025 | 8/15/2025 | 4 | 16,000 | 55215-B(T) |
9/9/2025 | 9/12/2025 | 4 | 16,000 | 55215-B(T) |
10/7/2025 | 10/10/2025 | 4 | 16,000 | 55215-B(T) |
11/11/2025 | 11/14/2025 | 4 | 16,000 | 55215-B(T) |
12/9/2025 | 12/12/2025 | 4 | 16,000 | 55215-B(T) |
10/2/2024 | 10/4/2024 | 3 | 12,000 | 55199-A(T) |
11/6/2024 | 11/8/2024 | 3 | 12,000 | 55199-A(T) |
12/2/2024 | 12/4/2024 | 3 | 12,000 | 55199-A(T) |
1/15/2025 | 1/17/2025 | 3 | 12,000 | 55199-A(T) |
2/5/2025 | 2/7/2025 | 3 | 12,000 | 55199-A(T) |
3/5/2025 | 3/7/2025 | 3 | 12,000 | 55199-A(T) |
4/28/2025 | 4/30/2025 | 3 | 12,000 | 55199-A(T) |
5/7/2025 | 5/9/2025 | 3 | 12,000 | 55199-A(T) |
6/4/2025 | 6/6/2025 | 3 | 12,000 | 55199-A(T) |
7/29/2025 | 7/31/2025 | 3 | 12,000 | 55199-A(T) |
8/6/2025 | 8/8/2025 | 3 | 12,000 | 55199-A(T) |
9/3/2025 | 9/5/2025 | 3 | 12,000 | 55199-A(T) |
10/1/2025 | 10/3/2025 | 3 | 12,000 | 55199-A(T) |
11/4/2025 | 11/7/2025 | 3 | 12,000 | 55199-A(T) |
12/1/2025 | 12/3/2025 | 3 | 12,000 | 55199-A(T) |
10/7/2024 | 10/8/2024 | 2 | 8,000 | 55200-A(T) |
11/14/2024 | 11/15/2024 | 2 | 8,000 | 55200-A(T) |
12/2/2024 | 12/3/2024 | 2 | 8,000 | 55200-A(T) |
1/16/2025 | 1/17/2025 | 2 | 8,000 | 55200-A(T) |
2/6/2025 | 2/7/2025 | 2 | 8,000 | 55200-A(T) |
3/3/2025 | 3/4/2025 | 2 | 8,000 | 55200-A(T) |
4/17/2025 | 4/18/2025 | 2 | 8,000 | 55200-A(T) |
5/13/2025 | 5/14/2025 | 2 | 8,000 | 55200-A(T) |
6/9/2025 | 6/10/2025 | 2 | 8,000 | 55200-A(T) |
7/8/2025 | 7/9/2025 | 2 | 8,000 | 55200-A(T) |
8/4/2025 | 8/5/2025 | 2 | 8,000 | 55200-A(T) |
9/8/2025 | 9/9/2025 | 2 | 8,000 | 55200-A(T) |
10/6/2025 | 10/7/2025 | 2 | 8,000 | 55200-A(T) |
11/13/2025 | 11/14/2025 | 2 | 8,000 | 55200-A(T) |
12/1/2025 | 12/2/2025 | 2 | 8,000 | 55200-A(T) |
4 | 16,000 | 55217-A | ||
10/7/2024 | 10/11/2024 | 5 | 20,000 | 55234-A(T) |
11/25/2024 | 11/29/2024 | 5 | 20,000 | 55234-A(T) |
12/23/2024 | 12/27/2024 | 5 | 20,000 | 55234-A(T) |
1/20/2025 | 1/24/2025 | 5 | 20,000 | 55234-A(T) |
2/24/2025 | 2/28/2025 | 5 | 20,000 | 55234-A(T) |
3/24/2025 | 3/28/2025 | 5 | 20,000 | 55234-A(T) |
4/21/2025 | 4/25/2025 | 5 | 20,000 | 55234-A(T) |
5/26/2025 | 5/30/2025 | 5 | 20,000 | 55234-A(T) |
6/23/2025 | 6/27/2025 | 5 | 20,000 | 55234-A(T) |
7/14/2025 | 7/18/2025 | 5 | 20,000 | 55234-A(T) |
8/25/2025 | 8/29/2025 | 5 | 20,000 | 55234-A(T) |
9/22/2025 | 9/26/2025 | 5 | 20,000 | 55234-A(T) |
10/6/2025 | 10/10/2025 | 5 | 20,000 | 55234-A(T) |
11/24/2025 | 11/28/2025 | 5 | 20,000 | 55234-A(T) |
12/22/2025 | 12/26/2025 | 5 | 20,000 | 55234-A(T) |
10/28/2024 | 10/30/2024 | 3 | 12,000 | 55238-B(T) |
11/11/2024 | 11/13/2024 | 3 | 12,000 | 55238-B(T) |
12/11/2024 | 12/13/2024 | 3 | 12,000 | 55238-B(T) |
1/15/2025 | 1/17/2025 | 3 | 12,000 | 55238-B(T) |
2/17/2025 | 2/19/2025 | 3 | 12,000 | 55238-B(T) |
3/10/2025 | 3/12/2025 | 3 | 12,000 | 55238-B(T) |
4/28/2025 | 4/30/2025 | 3 | 12,000 | 55238-B(T) |
5/14/2025 | 5/16/2025 | 3 | 12,000 | 55238-B(T) |
6/17/2025 | 6/19/2025 | 3 | 12,000 | 55238-B(T) |
7/29/2025 | 7/31/2025 | 3 | 12,000 | 55238-B(T) |
8/13/2025 | 8/15/2025 | 3 | 12,000 | 55238-B(T) |
9/3/2025 | 9/5/2025 | 3 | 12,000 | 55238-B(T) |
10/20/2025 | 10/22/2025 | 3 | 12,000 | 55238-B(T) |
11/10/2025 | 11/12/2025 | 3 | 12,000 | 55238-B(T) |
12/10/2025 | 12/12/2025 | 3 | 12,000 | 55238-B(T) |
10/7/2024 | 10/8/2024 | 2 | 8,000 | 55251-A(T) |
11/4/2024 | 11/5/2024 | 2 | 8,000 | 55251-A(T) |
12/2/2024 | 12/3/2024 | 2 | 8,000 | 55251-A(T) |
1/6/2025 | 1/7/2025 | 2 | 8,000 | 55251-A(T) |
2/3/2025 | 2/4/2025 | 2 | 8,000 | 55251-A(T) |
3/6/2025 | 3/7/2025 | 2 | 8,000 | 55251-A(T) |
4/17/2025 | 4/18/2025 | 2 | 8,000 | 55251-A(T) |
5/8/2025 | 5/9/2025 | 2 | 8,000 | 55251-A(T) |
6/5/2025 | 6/6/2025 | 2 | 8,000 | 55251-A(T) |
7/1/2025 | 7/2/2025 | 2 | 8,000 | 55251-A(T) |
8/4/2025 | 8/5/2025 | 2 | 8,000 | 55251-A(T) |
9/1/2025 | 9/2/2025 | 2 | 8,000 | 55251-A(T) |
10/6/2025 | 10/7/2025 | 2 | 8,000 | 55251-A(T) |
11/3/2025 | 11/4/2025 | 2 | 8,000 | 55251-A(T) |
12/1/2025 | 12/2/2025 | 2 | 8,000 | 55251-A(T) |
10/7/2024 | 10/11/2024 | 5 | 12,000 | 10972(T) |
11/4/2024 | 11/8/2024 | 5 | 12,000 | 10972(T) |
12/9/2024 | 12/13/2024 | 5 | 12,000 | 10972(T) |
1/6/2025 | 1/10/2025 | 5 | 12,000 | 10972(T) |
2/3/2025 | 2/7/2025 | 5 | 12,000 | 10972(T) |
3/3/2025 | 3/7/2025 | 5 | 12,000 | 10972(T) |
4/21/2025 | 4/25/2025 | 5 | 12,000 | 10972(T) |
5/19/2025 | 5/23/2025 | 5 | 12,000 | 10972(T) |
6/9/2025 | 6/13/2025 | 5 | 12,000 | 10972(T) |
7/14/2025 | 7/18/2025 | 5 | 12,000 | 10972(T) |
8/4/2025 | 8/8/2025 | 5 | 12,000 | 10972(T) |
9/1/2025 | 9/5/2025 | 5 | 12,000 | 10972(T) |
10/6/2025 | 10/10/2025 | 5 | 12,000 | 10972(T) |
11/3/2025 | 11/7/2025 | 5 | 12,000 | 10972(T) |
12/8/2025 | 12/12/2025 | 5 | 12,000 | 10972(T) |
10/15/2024 | 10/18/2024 | 4 | 16,000 | 10987(T) |
11/12/2024 | 11/15/2024 | 4 | 16,000 | 10987(T) |
12/17/2024 | 12/20/2024 | 4 | 16,000 | 10987(T) |
1/14/2025 | 1/17/2025 | 4 | 16,000 | 10987(T) |
2/10/2025 | 2/14/2025 | 4 | 16,000 | 10987(T) |
3/18/2025 | 3/21/2025 | 4 | 16,000 | 10987(T) |
4/1/2025 | 4/4/2025 | 4 | 16,000 | 10987(T) |
5/27/2025 | 5/30/2025 | 4 | 16,000 | 10987(T) |
6/17/2025 | 6/20/2025 | 4 | 16,000 | 10987(T) |
7/22/2025 | 7/25/2025 | 4 | 16,000 | 10987(T) |
8/5/2025 | 8/8/2025 | 4 | 16,000 | 10987(T) |
9/16/2025 | 9/19/2025 | 4 | 16,000 | 10987(T) |
10/14/2025 | 10/17/2025 | 4 | 16,000 | 10987(T) |
11/11/2025 | 11/14/2025 | 4 | 16,000 | 10987(T) |
12/16/2025 | 12/19/2025 | 4 | 16,000 | 10987(T) |
10/28/2024 | 10/30/2024 | 3 | 12,000 | 10997 |
11/25/2024 | 11/27/2024 | 3 | 12,000 | 10997 |
12/23/2024 | 12/25/2024 | 3 | 12,000 | 10997 |
1/27/2025 | 1/29/2025 | 3 | 12,000 | 10997 |
2/24/2025 | 2/26/2025 | 3 | 12,000 | 10997 |
3/26/2025 | 3/28/2025 | 3 | 12,000 | 10997 |
4/28/2025 | 4/30/2025 | 3 | 12,000 | 10997 |
5/28/2025 | 5/30/2025 | 3 | 12,000 | 10997 |
6/23/2025 | 6/25/2025 | 3 | 12,000 | 10997 |
7/29/2025 | 7/31/2025 | 3 | 12,000 | 10997 |
8/13/2025 | 8/15/2025 | 3 | 12,000 | 10997 |
9/24/2025 | 9/26/2025 | 3 | 12,000 | 10997 |
10/27/2025 | 10/29/2025 | 3 | 12,000 | 10997 |
11/24/2025 | 11/26/2025 | 3 | 12,000 | 10997 |
12/24/2025 | 12/26/2025 | 3 | 12,000 | 10997 |
10/28/2024 | 11/1/2024 | 5 | 20,000 | 20761 |
11/25/2024 | 11/29/2024 | 5 | 20,000 | 20761 |
12/16/2024 | 12/20/2024 | 5 | 20,000 | 20761 |
1/20/2025 | 1/24/2025 | 5 | 20,000 | 20761 |
2/17/2025 | 2/21/2025 | 5 | 20,000 | 20761 |
3/3/2025 | 3/7/2025 | 5 | 20,000 | 20761 |
4/21/2025 | 4/25/2025 | 5 | 20,000 | 20761 |
5/26/2025 | 5/30/2025 | 5 | 20,000 | 20761 |
6/23/2025 | 6/27/2025 | 5 | 20,000 | 20761 |
7/21/2025 | 7/25/2025 | 5 | 20,000 | 20761 |
8/25/2025 | 8/29/2025 | 5 | 20,000 | 20761 |
9/22/2025 | 9/26/2025 | 5 | 20,000 | 20761 |
10/27/2025 | 10/31/2025 | 5 | 20,000 | 20761 |
11/24/2025 | 11/28/2025 | 5 | 20,000 | 20761 |
12/15/2025 | 12/19/2025 | 5 | 20,000 | 20761 |
11/11/2024 | 11/15/2024 | 5 | 20,000 | 20764(T) |
12/9/2024 | 12/13/2024 | 5 | 20,000 | 20764(T) |
1/6/2025 | 1/10/2025 | 5 | 20,000 | 20764(T) |
2/3/2025 | 2/7/2025 | 5 | 20,000 | 20764(T) |
3/3/2025 | 3/7/2025 | 5 | 20,000 | 20764(T) |
4/21/2025 | 4/25/2025 | 5 | 20,000 | 20764(T) |
5/26/2025 | 5/30/2025 | 5 | 20,000 | 20764(T) |
6/9/2025 | 6/13/2025 | 5 | 20,000 | 20764(T) |
7/21/2025 | 7/25/2025 | 5 | 20,000 | 20764(T) |
8/18/2025 | 8/22/2025 | 5 | 20,000 | 20764(T) |
9/8/2025 | 9/12/2025 | 5 | 20,000 | 20764(T) |
10/27/2025 | 10/31/2025 | 5 | 20,000 | 20764(T) |
11/10/2025 | 11/14/2025 | 5 | 20,000 | 20764(T) |
12/8/2025 | 12/12/2025 | 5 | 20,000 | 20764(T) |
10/7/2024 | 10/8/2024 | 2 | 8,000 | 20778(T) |
11/14/2024 | 11/15/2024 | 2 | 8,000 | 20778(T) |
12/2/2024 | 12/3/2024 | 2 | 8,000 | 20778(T) |
1/16/2025 | 1/17/2025 | 2 | 8,000 | 20778(T) |
2/10/2025 | 2/11/2025 | 2 | 8,000 | 20778(T) |
3/27/2025 | 3/28/2025 | 2 | 8,000 | 20778(T) |
4/17/2025 | 4/18/2025 | 2 | 8,000 | 20778(T) |
5/22/2025 | 5/23/2025 | 2 | 8,000 | 20778(T) |
6/12/2025 | 6/13/2025 | 2 | 8,000 | 20778(T) |
7/8/2025 | 7/9/2025 | 2 | 8,000 | 20778(T) |
8/7/2025 | 8/8/2025 | 2 | 8,000 | 20778(T) |
9/1/2025 | 9/2/2025 | 2 | 8,000 | 20778(T) |
10/6/2025 | 10/7/2025 | 2 | 8,000 | 20778(T) |
11/13/2025 | 11/14/2025 | 2 | 8,000 | 20778(T) |
12/1/2025 | 12/2/2025 | 2 | 8,000 | 20778(T) |
10/2/2024 | 10/4/2024 | 3 | 12,000 | 20779 |
11/6/2024 | 11/8/2024 | 3 | 12,000 | 20779 |
12/11/2024 | 12/13/2024 | 3 | 12,000 | 20779 |
1/8/2025 | 1/10/2025 | 3 | 12,000 | 20779 |
2/19/2025 | 2/21/2025 | 3 | 12,000 | 20779 |
3/5/2025 | 3/7/2025 | 3 | 12,000 | 20779 |
4/28/2025 | 4/30/2025 | 3 | 12,000 | 20779 |
5/7/2025 | 5/9/2025 | 3 | 12,000 | 20779 |
6/4/2025 | 6/6/2025 | 3 | 12,000 | 20779 |
7/29/2025 | 7/31/2025 | 3 | 12,000 | 20779 |
8/6/2025 | 8/8/2025 | 3 | 12,000 | 20779 |
9/3/2025 | 9/5/2025 | 3 | 12,000 | 20779 |
10/1/2025 | 10/3/2025 | 3 | 12,000 | 20779 |
11/5/2025 | 11/7/2025 | 3 | 12,000 | 20779 |
12/10/2025 | 12/12/2025 | 3 | 12,000 | 20779 |
11/4/2024 | 11/8/2024 | 5 | 20,000 | WS-011T00(T) |
12/9/2024 | 12/13/2024 | 5 | 20,000 | WS-011T00(T) |
1/13/2025 | 1/17/2025 | 5 | 20,000 | WS-011T00(T) |
2/3/2025 | 2/7/2025 | 5 | 20,000 | WS-011T00(T) |
3/10/2025 | 3/14/2025 | 5 | 20,000 | WS-011T00(T) |
4/21/2025 | 4/25/2025 | 5 | 20,000 | WS-011T00(T) |
5/19/2025 | 5/23/2025 | 5 | 20,000 | WS-011T00(T) |
6/9/2025 | 6/13/2025 | 5 | 20,000 | WS-011T00(T) |
7/14/2025 | 7/18/2025 | 5 | 20,000 | WS-011T00(T) |
8/4/2025 | 8/8/2025 | 5 | 20,000 | WS-011T00(T) |
9/1/2025 | 9/5/2025 | 5 | 20,000 | WS-011T00(T) |
9/29/2025 | 10/3/2025 | 5 | 20,000 | WS-011T00(T) |
11/3/2025 | 11/7/2025 | 5 | 20,000 | WS-011T00(T) |
12/8/2025 | 12/12/2025 | 5 | 20,000 | WS-011T00(T) |
10/8/2024 | 10/11/2024 | 4 | 16,000 | DP-600T00 |
1/14/2025 | 1/17/2025 | 4 | 16,000 | DP-600T00 |
2/10/2025 | 2/14/2025 | 4 | 16,000 | DP-600T00 |
3/17/2025 | 3/20/2025 | 4 | 16,000 | DP-600T00 |
4/8/2025 | 4/11/2025 | 4 | 16,000 | DP-600T00 |
5/6/2025 | 5/9/2025 | 4 | 16,000 | DP-600T00 |
6/9/2025 | 6/12/2025 | 4 | 16,000 | DP-600T00 |
7/15/2025 | 7/18/2025 | 4 | 16,000 | DP-600T00 |
8/5/2025 | 8/8/2025 | 4 | 16,000 | DP-600T00 |
9/2/2025 | 9/5/2025 | 4 | 16,000 | DP-600T00 |
10/7/2025 | 10/10/2025 | 4 | 16,000 | DP-600T00 |
11/4/2025 | 11/7/2025 | 4 | 16,000 | DP-600T00 |
12/22/2025 | 12/25/2025 | 4 | 16,000 | DP-600T00 |
1/17/2025 | 1/17/2025 | 1 | 4,000 | DP-3011 |
2/7/2025 | 2/7/2025 | 1 | 4,000 | DP-3011 |
3/3/2025 | 3/3/2025 | 1 | 4,000 | DP-3011 |
4/4/2025 | 4/4/2025 | 1 | 4,000 | DP-3011 |
5/2/2025 | 5/2/2025 | 1 | 4,000 | DP-3011 |
6/9/2025 | 6/9/2025 | 1 | 4,000 | DP-3011 |
7/18/2025 | 7/18/2025 | 1 | 4,000 | DP-3011 |
8/8/2025 | 8/8/2025 | 1 | 4,000 | DP-3011 |
9/12/2025 | 9/12/2025 | 1 | 4,000 | DP-3011 |
10/6/2025 | 10/6/2025 | 1 | 4,000 | DP-3011 |
11/14/2025 | 11/14/2025 | 1 | 4,000 | DP-3011 |
12/22/2025 | 12/22/2025 | 1 | 4,000 | DP-3011 |
10/18/2024 | 10/18/2024 | 1 | 4,000 | DP-3012 |
1/20/2025 | 1/20/2025 | 1 | 4,000 | DP-3012 |
2/21/2025 | 2/21/2025 | 1 | 4,000 | DP-3012 |
3/24/2025 | 3/24/2025 | 1 | 4,000 | DP-3012 |
4/21/2025 | 4/21/2025 | 1 | 4,000 | DP-3012 |
5/19/2025 | 5/19/2025 | 1 | 4,000 | DP-3012 |
6/16/2025 | 6/16/2025 | 1 | 4,000 | DP-3012 |
7/18/2025 | 7/18/2025 | 1 | 4,000 | DP-3012 |
8/15/2025 | 8/15/2025 | 1 | 4,000 | DP-3012 |
9/19/2025 | 9/19/2025 | 1 | 4,000 | DP-3012 |
10/24/2025 | 10/24/2025 | 1 | 4,000 | DP-3012 |
11/17/2025 | 11/17/2025 | 1 | 4,000 | DP-3012 |
12/25/2025 | 12/25/2025 | 1 | 4,000 | DP-3012 |
11/11/2024 | 11/11/2024 | 1 | 4,000 | DP-3014 |
1/10/2025 | 1/10/2025 | 1 | 4,000 | DP-3014 |
2/10/2025 | 2/10/2025 | 1 | 4,000 | DP-3014 |
3/14/2025 | 3/14/2025 | 1 | 4,000 | DP-3014 |
4/11/2025 | 4/11/2025 | 1 | 4,000 | DP-3014 |
5/16/2025 | 5/16/2025 | 1 | 4,000 | DP-3014 |
6/13/2025 | 6/13/2025 | 1 | 4,000 | DP-3014 |
7/21/2025 | 7/21/2025 | 1 | 4,000 | DP-3014 |
8/15/2025 | 8/15/2025 | 1 | 4,000 | DP-3014 |
9/8/2025 | 9/8/2025 | 1 | 4,000 | DP-3014 |
10/10/2025 | 10/10/2025 | 1 | 4,000 | DP-3014 |
11/14/2025 | 11/14/2025 | 1 | 4,000 | DP-3014 |
12/8/2025 | 12/8/2025 | 1 | 4,000 | DP-3014 |
10/25/2024 | 10/25/2024 | 1 | 4,000 | DP-605T00 |
1/13/2025 | 1/13/2025 | 1 | 4,000 | DP-605T00 |
2/21/2025 | 2/21/2025 | 1 | 4,000 | DP-605T00 |
3/28/2025 | 3/28/2025 | 1 | 4,000 | DP-605T00 |
4/21/2025 | 4/21/2025 | 1 | 4,000 | DP-605T00 |
5/2/2025 | 5/2/2025 | 1 | 4,000 | DP-605T00 |
6/2/2025 | 6/2/2025 | 1 | 4,000 | DP-605T00 |
7/21/2025 | 7/21/2025 | 1 | 4,000 | DP-605T00 |
8/8/2025 | 8/8/2025 | 1 | 4,000 | DP-605T00 |
9/19/2025 | 9/19/2025 | 1 | 4,000 | DP-605T00 |
10/20/2025 | 10/20/2025 | 1 | 4,000 | DP-605T00 |
11/21/2025 | 11/21/2025 | 1 | 4,000 | DP-605T00 |
12/19/2025 | 12/19/2025 | 1 | 4,000 | DP-605T00 |
10/18/2024 | 10/18/2024 | 1 | 4,000 | DP-900T00(T) |
11/11/2024 | 11/11/2024 | 1 | 4,000 | DP-900T00(T) |
12/16/2024 | 12/16/2024 | 1 | 4,000 | DP-900T00(T) |
1/17/2025 | 1/17/2025 | 1 | 4,000 | DP-900T00(T) |
2/14/2025 | 2/14/2025 | 1 | 4,000 | DP-900T00(T) |
3/14/2025 | 3/14/2025 | 1 | 4,000 | DP-900T00(T) |
4/18/2025 | 4/18/2025 | 1 | 4,000 | DP-900T00(T) |
5/19/2025 | 5/19/2025 | 1 | 4,000 | DP-900T00(T) |
6/13/2025 | 6/13/2025 | 1 | 4,000 | DP-900T00(T) |
7/14/2025 | 7/14/2025 | 1 | 4,000 | DP-900T00(T) |
8/15/2025 | 8/15/2025 | 1 | 4,000 | DP-900T00(T) |
9/15/2025 | 9/15/2025 | 1 | 4,000 | DP-900T00(T) |
10/17/2025 | 10/17/2025 | 1 | 4,000 | DP-900T00(T) |
11/14/2025 | 11/14/2025 | 1 | 4,000 | DP-900T00(T) |
12/15/2025 | 12/15/2025 | 1 | 4,000 | DP-900T00(T) |
6/4/2024 | 6/7/2024 | 4 | 8,000 | IC-001T00 |
7/2/2024 | 7/5/2024 | 4 | 8,000 | IC-001T00 |
8/6/2024 | 8/9/2024 | 4 | 8,000 | IC-001T00 |
9/10/2024 | 9/13/2024 | 4 | 8,000 | IC-001T00 |
10/1/2024 | 10/4/2024 | 4 | 8,000 | IC-001T00 |
11/5/2024 | 11/8/2024 | 4 | 8,000 | IC-001T00 |
12/10/2024 | 12/13/2024 | 4 | 8,000 | IC-001T00 |
1 | 12,900 | ERT-GAI01 | ||
1/20/2025 | 1/20/2025 | 1 | 4,000 | MS-4017 |
2/21/2025 | 2/21/2025 | 1 | 4,000 | MS-4017 |
3/24/2025 | 3/24/2025 | 1 | 4,000 | MS-4017 |
4/21/2025 | 4/21/2025 | 1 | 4,000 | MS-4017 |
5/19/2025 | 5/19/2025 | 1 | 4,000 | MS-4017 |
6/16/2025 | 6/16/2025 | 1 | 4,000 | MS-4017 |
7/18/2025 | 7/18/2025 | 1 | 4,000 | MS-4017 |
8/15/2025 | 8/15/2025 | 1 | 4,000 | MS-4017 |
9/19/2025 | 9/19/2025 | 1 | 4,000 | MS-4017 |
10/24/2025 | 10/24/2025 | 1 | 4,000 | MS-4017 |
11/17/2025 | 11/17/2025 | 1 | 4,000 | MS-4017 |
12/15/2025 | 12/15/2025 | 1 | 4,000 | MS-4017 |
10/11/2024 | 10/11/2024 | 1 | 4,000 | MS-4004 |
11/18/2024 | 11/18/2024 | 1 | 4,000 | MS-4004 |
12/23/2024 | 12/23/2024 | 1 | 4,000 | MS-4004 |
1/6/2025 | 1/6/2025 | 1 | 4,000 | MS-4004 |
2/10/2025 | 2/10/2025 | 1 | 4,000 | MS-4004 |
3/3/2025 | 3/3/2025 | 1 | 4,000 | MS-4004 |
4/18/2025 | 4/18/2025 | 1 | 4,000 | MS-4004 |
5/9/2025 | 5/9/2025 | 1 | 4,000 | MS-4004 |
6/9/2025 | 6/9/2025 | 1 | 4,000 | MS-4004 |
7/9/2025 | 7/9/2025 | 1 | 4,000 | MS-4004 |
8/11/2025 | 8/11/2025 | 1 | 4,000 | MS-4004 |
9/5/2025 | 9/5/2025 | 1 | 4,000 | MS-4004 |
10/6/2025 | 10/6/2025 | 1 | 4,000 | MS-4004 |
11/3/2025 | 11/3/2025 | 1 | 4,000 | MS-4004 |
12/8/2025 | 12/8/2025 | 1 | 4,000 | MS-4004 |
10/21/2024 | 10/21/2024 | 1 | 4,000 | MS-4005 |
11/22/2024 | 11/22/2024 | 1 | 4,000 | MS-4005 |
12/20/2024 | 12/20/2024 | 1 | 4,000 | MS-4005 |
1/10/2025 | 1/10/2025 | 1 | 4,000 | MS-4005 |
2/14/2025 | 2/14/2025 | 1 | 4,000 | MS-4005 |
3/17/2025 | 3/17/2025 | 1 | 4,000 | MS-4005 |
4/7/2025 | 4/7/2025 | 1 | 4,000 | MS-4005 |
5/16/2025 | 5/16/2025 | 1 | 4,000 | MS-4005 |
6/20/2025 | 6/20/2025 | 1 | 4,000 | MS-4005 |
7/18/2025 | 7/18/2025 | 1 | 4,000 | MS-4005 |
8/8/2025 | 8/8/2025 | 1 | 4,000 | MS-4005 |
9/12/2025 | 9/12/2025 | 1 | 4,000 | MS-4005 |
10/10/2025 | 10/10/2025 | 1 | 4,000 | MS-4005 |
11/14/2025 | 11/14/2025 | 1 | 4,000 | MS-4005 |
12/12/2025 | 12/12/2025 | 1 | 4,000 | MS-4005 |
11/23/2024 | 11/23/2024 | 1 | 4,000 | MS-4006 |
10/18/2024 | 10/18/2024 | 1 | 4,000 | MS-4008 |
11/15/2024 | 11/15/2024 | 1 | 4,000 | MS-4008 |
12/16/2024 | 12/16/2024 | 1 | 4,000 | MS-4008 |
1/20/2025 | 1/20/2025 | 1 | 4,000 | MS-4008 |
2/21/2025 | 2/21/2025 | 1 | 4,000 | MS-4008 |
3/21/2025 | 3/24/2025 | 1 | 4,000 | MS-4008 |
4/25/2025 | 4/21/2025 | 1 | 4,000 | MS-4008 |
5/9/2025 | 5/19/2025 | 1 | 4,000 | MS-4008 |
6/16/2025 | 6/16/2025 | 1 | 4,000 | MS-4008 |
7/7/2025 | 7/18/2025 | 1 | 4,000 | MS-4008 |
8/8/2025 | 8/15/2025 | 1 | 4,000 | MS-4008 |
9/19/2025 | 9/19/2025 | 1 | 4,000 | MS-4008 |
10/17/2025 | 10/24/2025 | 1 | 4,000 | MS-4008 |
11/21/2025 | 11/17/2025 | 1 | 4,000 | MS-4008 |
12/15/2025 | 12/15/2025 | 1 | 4,000 | MS-4008 |
10/7/2024 | 10/7/2024 | 1 | 4,000 | AI-3002 |
11/4/2024 | 11/4/2024 | 1 | 4,000 | AI-3002 |
12/9/2024 | 12/9/2024 | 1 | 4,000 | AI-3002 |
1/10/2025 | 1/10/2025 | 1 | 4,000 | AI-3002 |
2/10/2025 | 2/10/2025 | 1 | 4,000 | AI-3002 |
3/14/2025 | 3/14/2025 | 1 | 4,000 | AI-3002 |
4/11/2025 | 4/11/2025 | 1 | 4,000 | AI-3002 |
5/16/2025 | 5/16/2025 | 1 | 4,000 | AI-3002 |
6/13/2025 | 6/13/2025 | 1 | 4,000 | AI-3002 |
7/21/2025 | 7/21/2025 | 1 | 4,000 | AI-3002 |
8/15/2025 | 8/15/2025 | 1 | 4,000 | AI-3002 |
9/8/2025 | 9/8/2025 | 1 | 4,000 | AI-3002 |
10/10/2025 | 10/10/2025 | 1 | 4,000 | AI-3002 |
11/14/2025 | 11/14/2025 | 1 | 4,000 | AI-3002 |
12/8/2025 | 12/8/2025 | 1 | 4,000 | AI-3002 |
10/21/2024 | 10/21/2024 | 1 | 4,000 | AI-3003 |
11/18/2024 | 11/18/2024 | 1 | 4,000 | AI-3003 |
12/23/2024 | 12/23/2024 | 1 | 4,000 | AI-3003 |
1/17/2025 | 1/17/2025 | 1 | 4,000 | AI-3003 |
2/7/2025 | 2/7/2025 | 1 | 4,000 | AI-3003 |
3/3/2025 | 3/3/2025 | 1 | 4,000 | AI-3003 |
4/4/2025 | 4/4/2025 | 1 | 4,000 | AI-3003 |
5/2/2025 | 5/2/2025 | 1 | 4,000 | AI-3003 |
6/9/2025 | 6/9/2025 | 1 | 4,000 | AI-3003 |
7/18/2025 | 7/18/2025 | 1 | 4,000 | AI-3003 |
8/8/2025 | 8/8/2025 | 1 | 4,000 | AI-3003 |
9/12/2025 | 9/12/2025 | 1 | 4,000 | AI-3003 |
10/6/2025 | 10/6/2025 | 1 | 4,000 | AI-3003 |
11/14/2025 | 11/14/2025 | 1 | 4,000 | AI-3003 |
12/22/2025 | 12/22/2025 | 1 | 4,000 | AI-3003 |
10/28/2024 | 10/28/2024 | 1 | 4,000 | AI-3004 |
11/25/2024 | 11/25/2024 | 1 | 4,000 | AI-3004 |
12/30/2024 | 12/30/2024 | 1 | 4,000 | AI-3004 |
1/13/2025 | 1/13/2025 | 1 | 4,000 | AI-3004 |
2/21/2025 | 2/21/2025 | 1 | 4,000 | AI-3004 |
3/7/2025 | 3/7/2025 | 1 | 4,000 | AI-3004 |
4/7/2025 | 4/7/2025 | 1 | 4,000 | AI-3004 |
5/9/2025 | 5/9/2025 | 1 | 4,000 | AI-3004 |
6/6/2025 | 6/6/2025 | 1 | 4,000 | AI-3004 |
7/9/2025 | 7/9/2025 | 1 | 4,000 | AI-3004 |
8/1/2025 | 8/1/2025 | 1 | 4,000 | AI-3004 |
9/19/2025 | 9/19/2025 | 1 | 4,000 | AI-3004 |
10/17/2025 | 10/17/2025 | 1 | 4,000 | AI-3004 |
11/3/2025 | 11/3/2025 | 1 | 4,000 | AI-3004 |
12/8/2025 | 12/8/2025 | 1 | 4,000 | AI-3004 |
10/4/2024 | 10/4/2024 | 1 | 4,000 | DP-3007 |
11/1/2024 | 11/1/2024 | 1 | 4,000 | DP-3007 |
12/2/2024 | 12/2/2024 | 1 | 4,000 | DP-3007 |
1/6/2025 | 1/6/2025 | 1 | 4,000 | DP-3007 |
2/14/2025 | 2/14/2025 | 1 | 4,000 | DP-3007 |
3/7/2025 | 3/7/2025 | 1 | 4,000 | DP-3007 |
4/18/2025 | 4/18/2025 | 1 | 4,000 | DP-3007 |
5/2/2025 | 5/2/2025 | 1 | 4,000 | DP-3007 |
6/6/2025 | 6/6/2025 | 1 | 4,000 | DP-3007 |
7/14/2025 | 7/14/2025 | 1 | 4,000 | DP-3007 |
8/15/2025 | 8/15/2025 | 1 | 4,000 | DP-3007 |
9/12/2025 | 9/12/2025 | 1 | 4,000 | DP-3007 |
10/10/2025 | 10/10/2025 | 1 | 4,000 | DP-3007 |
11/7/2025 | 11/7/2025 | 1 | 4,000 | DP-3007 |
12/12/2025 | 12/12/2025 | 1 | 4,000 | DP-3007 |
10/25/2024 | 10/25/2024 | 1 | 4,000 | DP-3015 |
11/29/2024 | 11/29/2024 | 1 | 4,000 | DP-3015 |
12/16/2024 | 12/16/2024 | 1 | 4,000 | DP-3015 |
1/3/2025 | 1/3/2025 | 1 | 4,000 | DP-3015 |
2/7/2025 | 2/7/2025 | 1 | 4,000 | DP-3015 |
3/10/2025 | 3/10/2025 | 1 | 4,000 | DP-3015 |
4/11/2025 | 4/11/2025 | 1 | 4,000 | DP-3015 |
5/9/2025 | 5/9/2025 | 1 | 4,000 | DP-3015 |
6/6/2025 | 6/6/2025 | 1 | 4,000 | DP-3015 |
7/7/2025 | 7/7/2025 | 1 | 4,000 | DP-3015 |
8/8/2025 | 8/8/2025 | 1 | 4,000 | DP-3015 |
9/5/2025 | 9/5/2025 | 1 | 4,000 | DP-3015 |
10/10/2025 | 10/10/2025 | 1 | 4,000 | DP-3015 |
11/14/2025 | 11/14/2025 | 1 | 4,000 | DP-3015 |
12/12/2025 | 12/12/2025 | 1 | 4,000 | DP-3015 |
10/28/2024 | 10/28/2024 | 1 | 4,000 | DP-602T00 |
11/18/2024 | 11/18/2024 | 1 | 4,000 | DP-602T00 |
12/20/2024 | 12/20/2024 | 1 | 4,000 | DP-602T00 |
1/3/2025 | 1/3/2025 | 1 | 4,000 | DP-602T00 |
2/7/2025 | 2/7/2025 | 1 | 4,000 | DP-602T00 |
3/10/2025 | 3/10/2025 | 1 | 4,000 | DP-602T00 |
4/11/2025 | 4/11/2025 | 1 | 4,000 | DP-602T00 |
5/9/2025 | 5/9/2025 | 1 | 4,000 | DP-602T00 |
6/6/2025 | 6/6/2025 | 1 | 4,000 | DP-602T00 |
7/7/2025 | 7/7/2025 | 1 | 4,000 | DP-602T00 |
8/8/2025 | 8/8/2025 | 1 | 4,000 | DP-602T00 |
9/5/2025 | 9/5/2025 | 1 | 4,000 | DP-602T00 |
10/10/2025 | 10/10/2025 | 1 | 4,000 | DP-602T00 |
11/14/2025 | 11/14/2025 | 1 | 4,000 | DP-602T00 |
12/12/2025 | 12/12/2025 | 1 | 4,000 | DP-602T00 |
10/7/2024 | 10/7/2024 | 1 | 4,000 | DP-603T00 |
11/25/2024 | 11/25/2024 | 1 | 4,000 | DP-603T00 |
12/27/2024 | 12/27/2024 | 1 | 4,000 | DP-603T00 |
1/17/2025 | 1/17/2025 | 1 | 4,000 | DP-603T00 |
2/14/2025 | 2/14/2025 | 1 | 4,000 | DP-603T00 |
3/14/2025 | 3/14/2025 | 1 | 4,000 | DP-603T00 |
4/18/2025 | 4/18/2025 | 1 | 4,000 | DP-603T00 |
5/19/2025 | 5/19/2025 | 1 | 4,000 | DP-603T00 |
6/13/2025 | 6/13/2025 | 1 | 4,000 | DP-603T00 |
7/14/2025 | 7/14/2025 | 1 | 4,000 | DP-603T00 |
8/15/2025 | 8/15/2025 | 1 | 4,000 | DP-603T00 |
9/15/2025 | 9/15/2025 | 1 | 4,000 | DP-603T00 |
10/17/2025 | 10/17/2025 | 1 | 4,000 | DP-603T00 |
11/14/2025 | 11/14/2025 | 1 | 4,000 | DP-603T00 |
12/15/2025 | 12/15/2025 | 1 | 4,000 | DP-603T00 |
11/8/2024 | 11/8/2024 | 1 | 4,000 | PL-7001 |
12/13/2024 | 12/13/2024 | 1 | 4,000 | PL-7001 |
1/20/2025 | 1/20/2025 | 1 | 4,000 | PL-7001 |
2/21/2025 | 2/21/2025 | 1 | 4,000 | PL-7001 |
3/21/2025 | 3/21/2025 | 1 | 4,000 | PL-7001 |
4/25/2025 | 4/25/2025 | 1 | 4,000 | PL-7001 |
5/9/2025 | 5/9/2025 | 1 | 4,000 | PL-7001 |
6/16/2025 | 6/16/2025 | 1 | 4,000 | PL-7001 |
7/7/2025 | 7/7/2025 | 1 | 4,000 | PL-7001 |
8/8/2025 | 8/8/2025 | 1 | 4,000 | PL-7001 |
9/19/2025 | 9/19/2025 | 1 | 4,000 | PL-7001 |
10/17/2025 | 10/17/2025 | 1 | 4,000 | PL-7001 |
11/21/2025 | 11/21/2025 | 1 | 4,000 | PL-7001 |
12/15/2025 | 12/15/2025 | 1 | 4,000 | PL-7001 |
11/22/2024 | 11/22/2024 | 1 | 4,000 | PL-7002 |
12/20/2024 | 12/20/2024 | 1 | 4,000 | PL-7002 |
1/3/2025 | 1/3/2025 | 1 | 4,000 | PL-7002 |
2/7/2025 | 2/7/2025 | 1 | 4,000 | PL-7002 |
3/10/2025 | 3/10/2025 | 1 | 4,000 | PL-7002 |
4/11/2025 | 4/11/2025 | 1 | 4,000 | PL-7002 |
5/9/2025 | 5/9/2025 | 1 | 4,000 | PL-7002 |
6/6/2025 | 6/6/2025 | 1 | 4,000 | PL-7002 |
7/7/2025 | 7/7/2025 | 1 | 4,000 | PL-7002 |
8/8/2025 | 8/8/2025 | 1 | 4,000 | PL-7002 |
9/5/2025 | 9/5/2025 | 1 | 4,000 | PL-7002 |
10/10/2025 | 10/10/2025 | 1 | 4,000 | PL-7002 |
11/14/2025 | 11/14/2025 | 1 | 4,000 | PL-7002 |
12/12/2025 | 12/12/2025 | 1 | 4,000 | PL-7002 |
11/29/2024 | 11/29/2024 | 1 | 4,000 | PL-7003 |
12/27/2024 | 12/27/2024 | 1 | 4,000 | PL-7003 |
1/10/2025 | 1/10/2025 | 1 | 4,000 | PL-7003 |
2/14/2025 | 2/14/2025 | 1 | 4,000 | PL-7003 |
3/17/2025 | 3/17/2025 | 1 | 4,000 | PL-7003 |
4/7/2025 | 4/7/2025 | 1 | 4,000 | PL-7003 |
5/16/2025 | 5/16/2025 | 1 | 4,000 | PL-7003 |
6/20/2025 | 6/20/2025 | 1 | 4,000 | PL-7003 |
7/18/2025 | 7/18/2025 | 1 | 4,000 | PL-7003 |
8/8/2025 | 8/8/2025 | 1 | 4,000 | PL-7003 |
9/12/2025 | 9/12/2025 | 1 | 4,000 | PL-7003 |
10/10/2025 | 10/10/2025 | 1 | 4,000 | PL-7003 |
11/14/2025 | 11/14/2025 | 1 | 4,000 | PL-7003 |
12/12/2025 | 12/12/2025 | 1 | 4,000 | PL-7003 |
12/7/2024 | 12/7/2024 | 1 | 4,000 | MS-4007 |
1/3/2025 | 1/3/2025 | 1 | 4,000 | MS-4007 |
2/7/2025 | 2/7/2025 | 1 | 4,000 | MS-4007 |
3/3/2025 | 3/3/2025 | 1 | 4,000 | MS-4007 |
4/4/2025 | 4/4/2025 | 1 | 4,000 | MS-4007 |
5/2/2025 | 5/2/2025 | 1 | 4,000 | MS-4007 |
6/9/2025 | 6/9/2025 | 1 | 4,000 | MS-4007 |
7/9/2025 | 7/9/2025 | 1 | 4,000 | MS-4007 |
8/8/2025 | 8/8/2025 | 1 | 4,000 | MS-4007 |
9/8/2025 | 9/8/2025 | 1 | 4,000 | MS-4007 |
10/6/2025 | 10/6/2025 | 1 | 4,000 | MS-4007 |
11/10/2025 | 11/10/2025 | 1 | 4,000 | MS-4007 |
12/12/2025 | 12/12/2025 | 1 | 4,000 | MS-4007 |
12/23/2024 | 12/26/2024 | 1 | 4,000 | AI-3018 |
1/3/2025 | 1/3/2025 | 1 | 4,000 | AI-3018 |
2/7/2025 | 2/7/2025 | 1 | 4,000 | AI-3018 |
3/10/2025 | 3/10/2025 | 1 | 4,000 | AI-3018 |
4/11/2025 | 4/11/2025 | 1 | 4,000 | AI-3018 |
5/2/2025 | 5/2/2025 | 1 | 4,000 | AI-3018 |
6/9/2025 | 6/9/2025 | 1 | 4,000 | AI-3018 |
7/4/2025 | 7/4/2025 | 1 | 4,000 | AI-3018 |
8/8/2025 | 8/8/2025 | 1 | 4,000 | AI-3018 |
9/5/2025 | 9/5/2025 | 1 | 4,000 | AI-3018 |
10/10/2025 | 10/10/2025 | 1 | 4,000 | AI-3018 |
11/17/2025 | 11/17/2025 | 1 | 4,000 | AI-3018 |
12/15/2025 | 12/15/2025 | 1 | 4,000 | AI-3018 |
12/28/2025 | 12/28/2025 | 1 | 4,000 | SC-5006 |
1/17/2025 | 1/17/2025 | 1 | 4,000 | SC-5006 |
2/14/2025 | 2/14/2025 | 1 | 4,000 | SC-5006 |
3/10/2025 | 3/10/2025 | 1 | 4,000 | SC-5006 |
4/7/2025 | 4/7/2025 | 1 | 4,000 | SC-5006 |
5/6/2025 | 5/6/2025 | 1 | 4,000 | SC-5006 |
6/9/2025 | 6/9/2025 | 1 | 4,000 | SC-5006 |
7/18/2025 | 7/18/2025 | 1 | 4,000 | SC-5006 |
8/15/2025 | 8/15/2025 | 1 | 4,000 | SC-5006 |
9/5/2025 | 9/5/2025 | 1 | 4,000 | SC-5006 |
10/17/2025 | 10/17/2025 | 1 | 4,000 | SC-5006 |
11/14/2025 | 11/14/2025 | 1 | 4,000 | SC-5006 |
12/15/2025 | 12/15/2025 | 1 | 4,000 | SC-5006 |
12/9/2024 | 12/9/2024 | 1 | 4,000 | MS-4009 |
1/6/2025 | 1/6/2025 | 1 | 4,000 | MS-4009 |
2/14/2025 | 2/14/2025 | 1 | 4,000 | MS-4009 |
3/10/2025 | 3/10/2025 | 1 | 4,000 | MS-4009 |
4/18/2025 | 4/18/2025 | 1 | 4,000 | MS-4009 |
5/13/2025 | 5/13/2025 | 1 | 4,000 | MS-4009 |
6/20/2025 | 6/20/2025 | 1 | 4,000 | MS-4009 |
7/18/2025 | 7/18/2025 | 1 | 4,000 | MS-4009 |
8/15/2025 | 8/15/2025 | 1 | 4,000 | MS-4009 |
9/19/2025 | 9/19/2025 | 1 | 4,000 | MS-4009 |
10/17/2025 | 10/17/2025 | 1 | 4,000 | MS-4009 |
11/21/2025 | 11/21/2025 | 1 | 4,000 | MS-4009 |
11/19/2025 | 11/19/2025 | 1 | 4,000 | MS-4009 |
12/23/2024 | 12/23/2024 | 1 | 4,000 | PL-7008 |
1/17/2025 | 1/17/2025 | 1 | 4,000 | PL-7008 |
2/24/2025 | 2/24/2025 | 1 | 4,000 | PL-7008 |
3/21/2025 | 3/21/2025 | 1 | 4,000 | PL-7008 |
4/25/2025 | 4/25/2025 | 1 | 4,000 | PL-7008 |
5/30/2025 | 5/30/2025 | 1 | 4,000 | PL-7008 |
6/27/2025 | 6/27/2025 | 1 | 4,000 | PL-7008 |
7/25/2025 | 7/25/2025 | 1 | 4,000 | PL-7008 |
8/22/2025 | 8/22/2025 | 1 | 4,000 | PL-7008 |
9/29/2025 | 9/29/2025 | 1 | 4,000 | PL-7008 |
10/20/2025 | 10/20/2025 | 1 | 4,000 | PL-7008 |
11/17/2025 | 11/17/2025 | 1 | 4,000 | PL-7008 |
12/26/2025 | 12/26/2025 | 1 | 4,000 | PL-7008 |
12/16/2024 | 12/16/2024 | 1 | 4,000 | MS-4010 |
1/10/2025 | 1/10/2025 | 1 | 4,000 | MS-4010 |
2/17/2025 | 2/17/2025 | 1 | 4,000 | MS-4010 |
3/17/2025 | 3/17/2025 | 1 | 4,000 | MS-4010 |
4/21/2025 | 4/21/2025 | 1 | 4,000 | MS-4010 |
5/23/2025 | 5/23/2025 | 1 | 4,000 | MS-4010 |
6/23/2025 | 6/23/2025 | 1 | 4,000 | MS-4010 |
7/21/2025 | 7/21/2025 | 1 | 4,000 | MS-4010 |
8/18/2025 | 8/18/2025 | 1 | 4,000 | MS-4010 |
9/26/2025 | 9/26/2025 | 1 | 4,000 | MS-4010 |
10/24/2025 | 10/24/2025 | 1 | 4,000 | MS-4010 |
11/24/2025 | 11/24/2025 | 1 | 4,000 | MS-4010 |
12/22/2025 | 12/22/2025 | 1 | 4,000 | MS-4010 |
12/27/2024 | 12/27/2024 | 1 | 4,000 | MS-4015 |
1/17/2025 | 1/17/2025 | 1 | 4,000 | MS-4015 |
2/24/2025 | 2/24/2025 | 1 | 4,000 | MS-4015 |
3/21/2025 | 3/21/2025 | 1 | 4,000 | MS-4015 |
4/21/2025 | 4/21/2025 | 1 | 4,000 | MS-4015 |
5/23/2025 | 5/23/2025 | 1 | 4,000 | MS-4015 |
6/27/2025 | 6/27/2025 | 1 | 4,000 | MS-4015 |
7/25/2025 | 7/25/2025 | 1 | 4,000 | MS-4015 |
8/22/2025 | 8/22/2025 | 1 | 4,000 | MS-4015 |
9/22/2025 | 9/22/2025 | 1 | 4,000 | MS-4015 |
10/27/2025 | 10/27/2025 | 1 | 4,000 | MS-4015 |
11/24/2025 | 11/24/2025 | 1 | 4,000 | MS-4015 |
12/22/2025 | 12/22/2025 | 1 | 4,000 | MS-4015 |
12/23/2024 | 12/23/2024 | 1 | 4,000 | MD-4011 |
1/20/2025 | 1/202/2025 | 1 | 4,000 | MD-4011 |
2/21/2025 | 2/21/2025 | 1 | 4,000 | MD-4011 |
3/24/2025 | 3/24/2025 | 1 | 4,000 | MD-4011 |
4/18/2025 | 4/18/2025 | 1 | 4,000 | MD-4011 |
5/23/2025 | 5/23/2025 | 1 | 4,000 | MD-4011 |
6/23/2025 | 6/23/2025 | 1 | 4,000 | MD-4011 |
7/25/2025 | 7/25/2025 | 1 | 4,000 | MD-4011 |
8/22/2025 | 8/22/2025 | 1 | 4,000 | MD-4011 |
9/26/2025 | 9/26/2025 | 1 | 4,000 | MD-4011 |
10/24/2025 | 10/24/2025 | 1 | 4,000 | MD-4011 |
11/28/2025 | 11/28/2025 | 1 | 4,000 | MD-4011 |
12/26/2025 | 12/26/2025 | 1 | 4,000 | MD-4011 |
1 | 10,000 | ERTV-01 | ||
10/28/2024 | 10/30/2024 | 3 | 26,000 | ERTV-02 |
11/11/2024 | 11/13/2024 | 3 | 26,000 | ERTV-02 |
12/16/2024 | 12/18/2024 | 3 | 26,000 | ERTV-02 |
8/13/2025 | 8/15/2025 | 3 | 26,000 | ERTV-02 |
9/24/2025 | 9/26/2025 | 3 | 26,000 | ERTV-02 |
10/15/2025 | 10/17/2025 | 3 | 26,000 | ERTV-02 |
11/26/2025 | 11/28/2025 | 3 | 26,000 | ERTV-02 |
12/17/2025 | 12/19/2025 | 3 | 26,000 | ERTV-02 |
10/16/2024 | 10/18/2024 | 3 | 26,000 | ERTV-03 |
8/20/2025 | 8/22/2025 | 3 | 26,000 | ERTV-03 |
11/17/2025 | 11/19/2025 | 3 | 26,000 | ERTV-03 |
10/20/2025 | 10/22/2025 | 3 | 26,000 | ERTV-04 |
11/25/2024 | 11/27/2024 | 3 | 26,000 | ERTV-05 |
9/3/2025 | 9/5/2025 | 3 | 26,000 | ERTV-05 |
12/24/2025 | 12/26/2025 | 3 | 26,000 | ERTV-05 |
5 | 90,000 | VSFT7 | ||
1 | 66,000 | VSTS7 | ||
3 | 40,000 | VSD8(T) | ||
25,000 | SRMICM82 | |||
6/10/2024 | 6/12/2024 | 3 | 40,000 | VSKDM7(T) |
4 | 49,000 | TKGIEICM110 | ||
6/24/2024 | 6/28/2024 | 5 | 60,000 | NSXTICM32(T) |
60,000 | NSXICM64(T) | |||
60,000 | VCDICM104(T) | |||
25,000 | VRASSSODM86(T) | |||
60,000 | VSANICM8(T) | |||
40,000 | VSTDCM8(T) | |||
60,000 | WS1DM22(T) | |||
90,000 | WS1DMUEMT22(T) | |||
5 | 66,000 | NSXTD32 | ||
9/23/2024 | 9/27/2024 | 5 | 60,000 | VROICM86(T) |
10/2/2023 | 10/6/2023 | 5 | 66,000 | NSXTTO32 |
10/16/2023 | 10/20/2023 | 5 | 60,000 | HDM8 |
11/6/2023 | 11/10/2023 | 5 | 60,000 | HDM8 |
25,000 | HIA8 | |||
7/23/2024 | 7/26/2024 | 4 | 49,000 | KFCO(T) |
10/7/2024 | 10/8/2024 | 2 | 10,800 | AWS-CPE(T) |
11/14/2024 | 11/15/2024 | 2 | 10,800 | AWS-CPE(T) |
12/19/2024 | 12/20/2024 | 2 | 10,800 | AWS-CPE(T) |
1/16/2025 | 1/17/2025 | 2 | 10,800 | AWS-CPE(T) |
2/13/2025 | 2/14/2025 | 2 | 10,800 | AWS-CPE(T) |
3/13/2025 | 3/14/2025 | 2 | 10,800 | AWS-CPE(T) |
6/4/2025 | 6/5/2025 | 2 | 10,800 | AWS-CPE(T) |
6/16/2025 | 6/17/2025 | 2 | 10,800 | AWS-CPE(T) |
7/15/2025 | 7/16/2025 | 2 | 10,800 | AWS-CPE(T) |
8/5/2025 | 8/6/2025 | 2 | 10,800 | AWS-CPE(T) |
11/22/2024 | 11/22/2024 | 1 | 10,800 | AWS-CPE |
12/6/2024 | 12/6/2024 | 1 | 10,800 | AWS-CPE |
1/21/2025 | 1/21/2025 | 1 | 5,900 | AWS-EBL |
2/25/2025 | 2/25/2025 | 1 | 5,900 | AWS-EBL |
1/20/2025 | 1/20/2025 | 1 | 5,900 | AWS-EFS |
2/21/2025 | 2/21/2025 | 1 | 5,900 | AWS-EFS |
10/9/2024 | 10/11/2024 | 3 | 32,400 | AWS-ARC(T) |
10/29/2024 | 10/31/2024 | 3 | 32,400 | AWS-ARC(T) |
11/27/2024 | 11/29/2024 | 3 | 32,400 | AWS-ARC(T) |
1/13/2025 | 1/15/2025 | 3 | 32,400 | AWS-ARC(T) |
2/5/2025 | 2/7/2025 | 3 | 32,400 | AWS-ARC(T) |
5/27/2025 | 5/29/2025 | 3 | 32,400 | AWS-ARC(T) |
6/18/2025 | 6/20/2025 | 3 | 32,400 | AWS-ARC(T) |
7/2/2025 | 7/4/2025 | 3 | 32,400 | AWS-ARC(T) |
8/20/2025 | 8/22/2025 | 3 | 32,400 | AWS-ARC(T) |
9/17/2025 | 9/19/2025 | 3 | 32,400 | AWS-ARC(T) |
10/15/2025 | 10/17/2025 | 3 | 32,400 | AWS-ARC(T) |
11/19/2025 | 11/21/2025 | 3 | 32,400 | AWS-ARC(T) |
12/17/2025 | 12/19/2025 | 3 | 32,400 | AWS-ARC(T) |
10,800 | AWS-WBP | |||
32,400 | AWS-ADA | |||
10,800 | AWS-AWB | |||
54,000 | AWS-ARA | |||
11/20/2024 | 11/22/2024 | 3 | 32,400 | AWS-COA(T) |
12/2/2024 | 12/4/2024 | 3 | 32,400 | AWS-COA(T) |
1/22/2025 | 1/24/2025 | 3 | 32,400 | AWS-COA(T) |
3/12/2025 | 3/14/2025 | 3 | 32,400 | AWS-COA(T) |
5/28/2025 | 5/30/2025 | 3 | 32,400 | AWS-COA(T) |
6/18/2025 | 6/20/2025 | 3 | 32,400 | AWS-COA(T) |
7/15/2025 | 7/17/2025 | 3 | 32,400 | AWS-COA(T) |
8/27/2025 | 8/29/2025 | 3 | 32,400 | AWS-COA(T) |
9/24/2025 | 9/26/2025 | 3 | 32,400 | AWS-COA(T) |
10/27/2025 | 10/29/2025 | 3 | 32,400 | AWS-COA(T) |
11/19/2025 | 11/21/2025 | 3 | 32,400 | AWS-COA(T) |
12/24/2025 | 12/26/2025 | 3 | 32,400 | AWS-COA(T) |
21,600 | AWS-FIN | |||
32,400 | AWS-CFM | |||
10/16/2024 | 10/18/2024 | 3 | 32,400 | AWS-DWA |
11/20/2024 | 11/22/2024 | 3 | 32,400 | AWS-DWA |
12/18/2024 | 12/20/2024 | 3 | 32,400 | AWS-DWA |
8/20/2025 | 8/22/2025 | 3 | 32,400 | AWS-DWA |
9/17/2025 | 9/19/2025 | 3 | 32,400 | AWS-DWA |
10/15/2025 | 10/17/2025 | 3 | 32,400 | AWS-DWA |
11/19/2025 | 11/21/2025 | 3 | 32,400 | AWS-DWA |
12/17/2025 | 12/19/2025 | 3 | 32,400 | AWS-DWA |
10,800 | AWS-BLK | |||
10,800 | AWS-BDA | |||
10,800 | AWS-BBD | |||
10,800 | AWS-BSD | |||
21,600 | AWS-AVA | |||
10/28/2024 | 10/30/2024 | 3 | 32,400 | AWS-PDD |
11/20/2024 | 11/22/2024 | 3 | 32,400 | AWS-PDD |
12/25/2024 | 12/27/2024 | 3 | 32,400 | AWS-PDD |
8/27/2025 | 8/29/2025 | 3 | 32,400 | AWS-PDD |
9/24/2025 | 9/26/2025 | 3 | 32,400 | AWS-PDD |
10/27/2025 | 10/29/2025 | 3 | 32,400 | AWS-PDD |
11/19/2025 | 11/21/2025 | 3 | 32,400 | AWS-PDD |
12/24/2025 | 12/26/2025 | 3 | 32,400 | AWS-PDD |
11/11/2024 | 11/13/2024 | 3 | 32,400 | AWS-DEV |
12/16/2024 | 12/18/2024 | 3 | 32,400 | AWS-DEV |
8/20/2025 | 8/22/2025 | 3 | 32,400 | AWS-DEV |
9/17/2025 | 9/19/2025 | 3 | 32,400 | AWS-DEV |
10/15/2025 | 10/17/2025 | 3 | 32,400 | AWS-DEV |
11/19/2025 | 11/21/2025 | 3 | 32,400 | AWS-DEV |
12/17/2025 | 12/19/2025 | 3 | 32,400 | AWS-DEV |
32,400 | AWS-ADE | |||
10/28/2024 | 10/30/2024 | 3 | 32,400 | AWS-DOP |
11/20/2024 | 11/22/2024 | 3 | 32,400 | AWS-DOP |
12/25/2024 | 12/27/2024 | 3 | 32,400 | AWS-DOP |
8/27/2025 | 8/29/2025 | 3 | 32,400 | AWS-DOP |
9/24/2025 | 9/26/2025 | 3 | 32,400 | AWS-DOP |
10/27/2025 | 10/29/2025 | 3 | 32,400 | AWS-DOP |
11/19/2025 | 11/21/2025 | 3 | 32,400 | AWS-DOP |
12/24/2025 | 12/26/2025 | 3 | 32,400 | AWS-DOP |
8/26/2024 | 8/26/2024 | 1 | 10,800 | AWS-DEL |
9/17/2024 | 9/17/2024 | 1 | 10,800 | AWS-DEL |
10/15/2024 | 10/15/2024 | 1 | 10,800 | AWS-DEL |
11/19/2024 | 11/19/2024 | 1 | 10,800 | AWS-DEL |
10/16/2024 | 10/18/2024 | 3 | 32,400 | AWS-MLE |
11/20/2024 | 11/22/2024 | 3 | 32,400 | AWS-MLE |
8/26/2025 | 8/28/2025 | 3 | 32,400 | AWS-MLE |
9/17/2025 | 9/19/2025 | 3 | 32,400 | AWS-MLE |
10/15/2025 | 10/17/2025 | 3 | 32,400 | AWS-MLE |
11/19/2025 | 11/21/2025 | 3 | 32,400 | AWS-MLE |
10,800 | AWS-PDS | |||
43,200 | AWS-MLP | |||
32,400 | AWS-SSD | |||
21,600 | AWS-VSE | |||
10/15/2024 | 10/15/2024 | 1 | 10,800 | AWS-MES |
11/18/2024 | 11/18/2024 | 1 | 10,800 | AWS-MES |
12/16/2024 | 12/16/2024 | 1 | 10,800 | AWS-MES |
1/13/2025 | 1/13/2025 | 1 | 10,800 | AWS-MES |
2/13/2025 | 2/13/2025 | 1 | 10,800 | AWS-MES |
3/14/2025 | 3/14/2025 | 1 | 10,800 | AWS-MES |
4/23/2025 | 4/23/2025 | 1 | 10,800 | AWS-MES |
5/19/2025 | 5/19/2025 | 1 | 10,800 | AWS-MES |
6/20/2025 | 6/20/2025 | 1 | 10,800 | AWS-MES |
7/1/2025 | 7/1/2025 | 1 | 10,800 | AWS-MES |
8/4/2025 | 8/4/2025 | 1 | 10,800 | AWS-MES |
9/15/2025 | 9/16/2025 | 2 | 10,800 | AWS-MES |
10/14/2025 | 10/15/2025 | 2 | 10,800 | AWS-MES |
11/14/2025 | 11/15/2025 | 2 | 10,800 | AWS-MES |
12/15/2025 | 12/16/2025 | 2 | 10,800 | AWS-MES |
10/16/2024 | 10/18/2024 | 3 | 32,400 | AWS-MGA |
6/18/2025 | 6/20/2025 | 3 | 32,400 | AWS-MGA |
8/6/2025 | 8/8/2025 | 3 | 32,400 | AWS-MGA |
10/15/2025 | 10/17/2025 | 3 | 32,400 | AWS-MGA |
11/19/2024 | 11/19/2024 | 1 | 10,800 | AWS-SES |
12/6/2024 | 12/6/2024 | 1 | 10,800 | AWS-SES |
1/13/2025 | 1/13/2025 | 1 | 10,800 | AWS-SES |
2/13/2025 | 2/13/2025 | 1 | 10,800 | AWS-SES |
3/14/2025 | 3/14/2025 | 1 | 10,800 | AWS-SES |
4/23/2025 | 4/23/2025 | 1 | 10,800 | AWS-SES |
5/19/2025 | 5/19/2025 | 1 | 10,800 | AWS-SES |
6/20/2025 | 6/20/2025 | 1 | 10,800 | AWS-SES |
7/18/2025 | 7/18/2025 | 1 | 10,800 | AWS-SES |
8/19/2025 | 8/19/2025 | 1 | 10,800 | AWS-SES |
9/15/2025 | 9/15/2025 | 1 | 10,800 | AWS-SES |
10/14/2025 | 10/14/2025 | 1 | 10,800 | AWS-SES |
11/14/2025 | 11/14/2025 | 1 | 10,800 | AWS-SES |
12/15/2025 | 12/15/2025 | 1 | 10,800 | AWS-SES |
8/27/2025 | 8/29/2025 | 3 | 32,400 | AWS-SEN |
9/24/2025 | 9/26/2025 | 3 | 32,400 | AWS-SEN |
10/27/2025 | 10/29/2025 | 3 | 32,400 | AWS-SEN |
11/19/2025 | 11/21/2025 | 3 | 32,400 | AWS-SEN |
12/24/2025 | 12/26/2025 | 3 | 32,400 | AWS-SEN |
10,800 | AWS-SGS | |||
10,800 | AWS-SBP | |||
10/28/2024 | 10/28/2024 | 1 | 10,800 | AWS-TES(T) |
11/18/2024 | 11/18/2024 | 1 | 10,800 | AWS-TES(T) |
12/6/2024 | 12/6/2024 | 1 | 10,800 | AWS-TES(T) |
12/20/2024 | 12/20/2024 | 1 | 10,800 | AWS-TES(T) |
1/23/2025 | 1/23/2025 | 1 | 10,800 | AWS-TES(T) |
2/27/2025 | 2/27/2025 | 1 | 10,800 | AWS-TES(T) |
4/29/2025 | 4/29/2025 | 1 | 10,800 | AWS-TES(T) |
5/26/2025 | 5/26/2025 | 1 | 10,800 | AWS-TES(T) |
6/17/2025 | 6/17/2025 | 1 | 10,800 | AWS-TES(T) |
7/1/2025 | 7/1/2025 | 1 | 10,800 | AWS-TES(T) |
7/14/2025 | 7/14/2025 | 1 | 10,800 | AWS-TES(T) |
8/19/2025 | 8/19/2025 | 1 | 10,800 | AWS-TES(T) |
8/25/2025 | 8/25/2025 | 1 | 10,800 | AWS-TES(T) |
9/16/2025 | 9/16/2025 | 1 | 10,800 | AWS-TES(T) |
10/14/2025 | 10/14/2025 | 1 | 10,800 | AWS-TES(T) |
11/6/2025 | 11/6/2025 | 1 | 10,800 | AWS-TES(T) |
12/9/2025 | 12/9/2025 | 1 | 10,800 | AWS-TES(T) |
11/29/2024 | 11/29/2024 | 0.5 | 10,800 | AWS-AIE |
12/16/2024 | 12/16/2024 | 0.5 | 10,800 | AWS-AIE |
10/2/2024 | 10/4/2024 | 3 | 45,000 | D1101651GC10 |
11/6/2024 | 11/8/2024 | 3 | 45,000 | D1101651GC10 |
12/11/2024 | 12/13/2024 | 3 | 45,000 | D1101651GC10 |
1/20/2025 | 1/22/2025 | 3 | 45,000 | D1101651GC10 |
4/21/2025 | 4/23/2025 | 3 | 45,000 | D1101651GC10 |
7/21/2025 | 7/23/2025 | 3 | 45,000 | D1101651GC10 |
10/20/2025 | 10/22/2025 | 3 | 45,000 | D1101651GC10 |
11/11/2024 | 11/15/2024 | 5 | 57,900 | D107509GC10 |
12/9/2024 | 12/13/2024 | 5 | 57,900 | D107509GC10 |
1/13/2025 | 1/17/2025 | 5 | 57,900 | D107509GC10 |
2/17/2025 | 2/21/2025 | 5 | 57,900 | D107509GC10 |
3/10/2025 | 3/14/2025 | 5 | 57,900 | D107509GC10 |
4/7/2025 | 4/11/2025 | 5 | 57,900 | D107509GC10 |
5/5/2025 | 5/9/2025 | 5 | 57,900 | D107509GC10 |
6/9/2025 | 6/13/2025 | 5 | 57,900 | D107509GC10 |
7/14/2025 | 7/18/2025 | 5 | 57,900 | D107509GC10 |
8/4/2025 | 8/8/2025 | 5 | 57,900 | D107509GC10 |
9/1/2025 | 9/5/2025 | 5 | 57,900 | D107509GC10 |
9/29/2025 | 10/3/2025 | 5 | 57,900 | D107509GC10 |
11/3/2025 | 11/7/2025 | 5 | 57,900 | D107509GC10 |
12/15/2025 | 12/19/2025 | 5 | 57,900 | D107509GC10 |
1/20/2025 | 1/24/2025 | 5 | 46,200 | D107511GC10 |
3/17/2025 | 3/21/2025 | 5 | 46,200 | D107511GC10 |
4/21/2025 | 4/25/2025 | 5 | 46,200 | D107511GC10 |
5/19/2025 | 5/23/2025 | 5 | 46,200 | D107511GC10 |
6/16/2025 | 6/20/2025 | 5 | 46,200 | D107511GC10 |
7/21/2025 | 7/25/2025 | 5 | 46,200 | D107511GC10 |
8/18/2025 | 8/22/2025 | 5 | 46,200 | D107511GC10 |
9/8/2025 | 9/12/2025 | 5 | 46,200 | D107511GC10 |
10/6/2025 | 10/10/2025 | 5 | 46,200 | D107511GC10 |
11/10/2025 | 11/14/2025 | 5 | 46,200 | D107511GC10 |
12/22/2025 | 12/26/2025 | 5 | 46,200 | D107511GC10 |
4/17/2025 | 4/18/2025 | 2 | 40,000 | D1105780GC10 |
6/5/2025 | 6/6/2025 | 2 | 40,000 | D1105780GC10 |
8/14/2025 | 8/15/2025 | 2 | 40,000 | D1105780GC10 |
10/21/2025 | 10/22/2025 | 2 | 40,000 | D1105780GC10 |
12/3/2025 | 12/4/2025 | 2 | 40,000 | D1105780GC10 |
4/17/2025 | 4/18/2025 | 2 | 40,000 | D1109439GC10 |
6/5/2025 | 6/6/2025 | 2 | 40,000 | D1109439GC10 |
8/14/2025 | 8/15/2025 | 2 | 40,000 | D1109439GC10 |
10/21/2025 | 10/22/2025 | 2 | 40,000 | D1109439GC10 |
12/3/2025 | 12/4/2025 | 2 | 40,000 | D1109439GC10 |
4/7/2025 | 4/11/2025 | 5 | 75,000 | D1106069GC10 |
6/9/2025 | 6/13/2025 | 5 | 75,000 | D1106069GC10 |
8/4/2025 | 8/8/2025 | 5 | 75,000 | D1106069GC10 |
10/6/2025 | 10/10/2025 | 5 | 75,000 | D1106069GC10 |
12/15/2025 | 12/19/2025 | 5 | 75,000 | D1106069GC10 |
10/28/2024 | 11/1/2024 | 5 | 57,900 | D108103GC20 |
11/18/2024 | 11/22/2024 | 5 | 57,900 | D108103GC20 |
12/23/2024 | 12/27/2024 | 5 | 57,900 | D108103GC20 |
1/27/2025 | 1/31/2025 | 5 | 57,900 | D108103GC20 |
3/24/2025 | 3/28/2025 | 5 | 57,900 | D108103GC20 |
5/26/2025 | 5/30/2025 | 5 | 57,900 | D108103GC20 |
6/23/2025 | 6/27/2025 | 5 | 57,900 | D108103GC20 |
8/25/2025 | 8/29/2025 | 5 | 57,900 | D108103GC20 |
9/15/2025 | 9/19/2025 | 5 | 57,900 | D108103GC20 |
10/27/2025 | 10/31/2025 | 5 | 57,900 | D108103GC20 |
11/17/2025 | 11/21/2025 | 5 | 57,900 | D108103GC20 |
11/12/2024 | 11/15/2024 | 4 | 58,600 | D108654GC10 |
12/10/2024 | 12/13/2024 | 4 | 58,600 | D108654GC10 |
1/28/2025 | 1/31/2025 | 4 | 58,600 | D108654GC10 |
4/22/2025 | 4/25/2025 | 4 | 58,600 | D108654GC10 |
7/22/2025 | 7/25/2025 | 4 | 58,600 | D108654GC10 |
5 | 56,500 | D78848GC20 | ||
10/15/2024 | 10/18/2024 | 4 | 58,600 | D108634GC10 |
11/19/2024 | 11/22/2024 | 4 | 58,600 | D108634GC10 |
12/17/2024 | 12/20/2024 | 4 | 58,600 | D108634GC10 |
1/14/2025 | 1/17/2025 | 4 | 58,600 | D108634GC10 |
2/10/2025 | 2/14/2025 | 4 | 58,600 | D108634GC10 |
3/11/2025 | 3/14/2025 | 4 | 58,600 | D108634GC10 |
4/22/2025 | 4/25/2025 | 4 | 58,600 | D108634GC10 |
5/13/2025 | 5/16/2025 | 4 | 58,600 | D108634GC10 |
6/17/2025 | 6/20/2025 | 4 | 58,600 | D108634GC10 |
7/15/2025 | 7/18/2025 | 4 | 58,600 | D108634GC10 |
8/11/2025 | 8/15/2025 | 4 | 58,600 | D108634GC10 |
9/16/2025 | 9/19/2025 | 4 | 58,600 | D108634GC10 |
10/14/2025 | 10/17/2025 | 4 | 58,600 | D108634GC10 |
11/11/2025 | 11/14/2025 | 4 | 58,600 | D108634GC10 |
12/16/2025 | 12/19/2025 | 4 | 58,600 | D108634GC10 |
11/4/2024 | 11/5/2024 | 2 | 40,000 | D108638GC10 |
12/2/2024 | 12/3/2024 | 2 | 40,000 | D108638GC10 |
2/13/2025 | 2/14/2025 | 2 | 40,000 | D108638GC10 |
4/17/2025 | 4/18/2025 | 2 | 40,000 | D108638GC10 |
6/5/2025 | 6/6/2025 | 2 | 40,000 | D108638GC10 |
8/14/2025 | 8/15/2025 | 2 | 40,000 | D108638GC10 |
10/16/2025 | 10/17/2025 | 2 | 40,000 | D108638GC10 |
10/7/2024 | 10/11/2024 | 5 | 75,000 | D108774GC20 |
11/18/2024 | 11/22/2024 | 5 | 75,000 | D108774GC20 |
12/16/2024 | 12/20/2024 | 5 | 75,000 | D108774GC20 |
1/13/2025 | 1/17/2025 | 5 | 75,000 | D108774GC20 |
2/17/2025 | 2/21/2025 | 5 | 75,000 | D108774GC20 |
3/10/2025 | 3/14/2025 | 5 | 75,000 | D108774GC20 |
4/21/2025 | 4/25/2025 | 5 | 75,000 | D108774GC20 |
5/19/2025 | 5/23/2025 | 5 | 75,000 | D108774GC20 |
6/16/2025 | 6/20/2025 | 5 | 75,000 | D108774GC20 |
7/14/2025 | 7/18/2025 | 5 | 75,000 | D108774GC20 |
8/18/2025 | 8/22/2025 | 5 | 75,000 | D108774GC20 |
9/15/2025 | 9/19/2025 | 5 | 75,000 | D108774GC20 |
11/10/2025 | 11/14/2025 | 5 | 75,000 | D108774GC20 |
12/15/2025 | 12/19/2025 | 5 | 75,000 | D108774GC20 |
10/28/2024 | 11/1/2024 | 5 | 75,000 | D108630GC10 |
11/25/2024 | 11/29/2024 | 5 | 75,000 | D108630GC10 |
12/23/2024 | 12/27/2024 | 5 | 75,000 | D108630GC10 |
1/13/2025 | 1/17/2025 | 5 | 75,000 | D108630GC10 |
2/17/2025 | 2/21/2025 | 5 | 75,000 | D108630GC10 |
3/10/2025 | 3/14/2025 | 5 | 75,000 | D108630GC10 |
4/21/2025 | 4/25/2025 | 5 | 75,000 | D108630GC10 |
5/19/2025 | 5/23/2025 | 5 | 75,000 | D108630GC10 |
6/16/2025 | 6/20/2025 | 5 | 75,000 | D108630GC10 |
7/14/2025 | 7/18/2025 | 5 | 75,000 | D108630GC10 |
8/18/2025 | 8/22/2025 | 5 | 75,000 | D108630GC10 |
9/15/2025 | 9/19/2025 | 5 | 75,000 | D108630GC10 |
11/10/2025 | 11/14/2025 | 5 | 75,000 | D108630GC10 |
12/15/2025 | 12/19/2025 | 5 | 75,000 | D108630GC10 |
2/13/2025 | 2/14/2025 | 2 | 40,000 | D1106095GC10 |
4/17/2025 | 4/18/2025 | 2 | 40,000 | D1106095GC10 |
6/5/2025 | 6/6/2025 | 2 | 40,000 | D1106095GC10 |
10/16/2025 | 10/17/2025 | 2 | 40,000 | D1106095GC10 |
2/19/2025 | 2/21/2025 | 3 | 45,000 | D1101948GC10 |
5/28/2025 | 5/30/2025 | 3 | 45,000 | D1101948GC10 |
8/20/2025 | 8/22/2025 | 3 | 45,000 | D1101948GC10 |
2/13/2025 | 2/14/2025 | 2 | 39,200 | D1101944GC10 |
5/26/2025 | 5/27/2025 | 2 | 39,200 | D1101944GC10 |
8/18/2025 | 8/19/2025 | 2 | 39,200 | D1101944GC10 |
10/28/2024 | 11/1/2024 | 5 | 70,500 | D110148GC10 |
11/25/2024 | 11/29/2024 | 5 | 70,500 | D110148GC10 |
12/16/2024 | 12/20/2024 | 5 | 70,500 | D110148GC10 |
1/13/2025 | 1/17/2025 | 5 | 70,500 | D110148GC10 |
2/17/2025 | 2/21/2025 | 5 | 70,500 | D110148GC10 |
3/10/2025 | 3/14/2025 | 5 | 70,500 | D110148GC10 |
5/26/2025 | 5/30/2025 | 5 | 70,500 | D110148GC10 |
6/9/2025 | 6/13/2025 | 5 | 70,500 | D110148GC10 |
7/14/2025 | 7/18/2025 | 5 | 70,500 | D110148GC10 |
8/4/2025 | 8/8/2025 | 5 | 70,500 | D110148GC10 |
9/8/2025 | 9/12/2025 | 5 | 70,500 | D110148GC10 |
10/6/2025 | 10/10/2025 | 5 | 70,500 | D110148GC10 |
11/17/2025 | 11/21/2025 | 5 | 70,500 | D110148GC10 |
12/15/2025 | 12/19/2025 | 5 | 70,500 | D110148GC10 |
10/7/2024 | 10/7/2024 | 1 | 2,500 | OF-19-NF(T) |
11/4/2024 | 11/4/2024 | 1 | 2,500 | OF-19-NF(T) |
12/6/2024 | 12/6/2024 | 1 | 2,500 | OF-19-NF(T) |
1/6/2025 | 1/6/2025 | 1 | 2,500 | OF-19-NF(T) |
2/3/2025 | 2/3/2025 | 1 | 2,500 | OF-19-NF(T) |
3/10/2025 | 3/10/2025 | 1 | 2,500 | OF-19-NF(T) |
4/4/2025 | 4/4/2025 | 1 | 2,500 | OF-19-NF(T) |
5/2/2025 | 5/2/2025 | 1 | 2,500 | OF-19-NF(T) |
6/9/2025 | 6/9/2025 | 1 | 2,500 | OF-19-NF(T) |
7/9/2025 | 7/9/2025 | 1 | 2,500 | OF-19-NF(T) |
8/11/2025 | 8/11/2025 | 1 | 2,500 | OF-19-NF(T) |
9/5/2025 | 9/5/2025 | 1 | 2,500 | OF-19-NF(T) |
10/6/2025 | 10/6/2025 | 1 | 2,500 | OF-19-NF(T) |
11/10/2025 | 11/10/2025 | 1 | 2,500 | OF-19-NF(T) |
12/4/2025 | 12/4/2025 | 1 | 2,500 | OF-19-NF(T) |
10/15/2024 | 10/16/2024 | 2 | 5,000 | WD-19-01(T) |
11/14/2024 | 11/15/2024 | 2 | 5,000 | WD-19-01(T) |
12/9/2024 | 12/10/2024 | 2 | 5,000 | WD-19-01(T) |
1/16/2025 | 1/17/2025 | 2 | 5,000 | WD-19-01(T) |
2/13/2025 | 2/14/2025 | 2 | 5,000 | WD-19-01(T) |
3/24/2025 | 3/25/2025 | 2 | 5,000 | WD-19-01(T) |
4/10/2025 | 4/11/2025 | 2 | 5,000 | WD-19-01(T) |
5/6/2025 | 5/7/2025 | 2 | 5,000 | WD-19-01(T) |
6/10/2025 | 6/11/2025 | 2 | 5,000 | WD-19-01(T) |
7/8/2025 | 7/9/2025 | 2 | 5,000 | WD-19-01(T) |
8/4/2025 | 8/5/2025 | 2 | 5,000 | WD-19-01(T) |
9/15/2025 | 9/16/2025 | 2 | 5,000 | WD-19-01(T) |
10/14/2025 | 10/15/2025 | 2 | 5,000 | WD-19-01(T) |
11/13/2025 | 11/14/2025 | 2 | 5,000 | WD-19-01(T) |
12/8/2025 | 12/9/2025 | 2 | 5,000 | WD-19-01(T) |
10/17/2024 | 10/18/2024 | 2 | 5,000 | WD-19-02(T) |
11/18/2024 | 11/19/2024 | 2 | 5,000 | WD-19-02(T) |
12/19/2024 | 12/20/2024 | 2 | 5,000 | WD-19-02(T) |
1/23/2025 | 1/24/2025 | 2 | 5,000 | WD-19-02(T) |
2/10/2025 | 2/11/2025 | 2 | 5,000 | WD-19-02(T) |
3/26/2025 | 3/27/2025 | 2 | 5,000 | WD-19-02(T) |
4/17/2025 | 4/18/2025 | 2 | 5,000 | WD-19-02(T) |
5/8/2025 | 5/9/2025 | 2 | 5,000 | WD-19-02(T) |
6/12/2025 | 6/13/2025 | 2 | 5,000 | WD-19-02(T) |
7/17/2025 | 7/18/2025 | 2 | 5,000 | WD-19-02(T) |
8/18/2025 | 8/19/2025 | 2 | 5,000 | WD-19-02(T) |
9/22/2025 | 9/23/2025 | 2 | 5,000 | WD-19-02(T) |
10/16/2025 | 10/17/2025 | 2 | 5,000 | WD-19-02(T) |
11/17/2025 | 11/18/2025 | 2 | 5,000 | WD-19-02(T) |
12/18/2025 | 12/19/2025 | 2 | 5,000 | WD-19-02(T) |
10/15/2024 | 10/16/2024 | 2 | 5,000 | EX-19-01(T) |
11/14/2024 | 11/15/2024 | 2 | 5,000 | EX-19-01(T) |
12/9/2024 | 12/10/2024 | 2 | 5,000 | EX-19-01(T) |
1/16/2025 | 1/17/2025 | 2 | 5,000 | EX-19-01(T) |
2/27/2025 | 2/28/2025 | 2 | 5,000 | EX-19-01(T) |
3/24/2025 | 3/25/2025 | 2 | 5,000 | EX-19-01(T) |
4/10/2025 | 4/11/2025 | 2 | 5,000 | EX-19-01(T) |
5/6/2025 | 5/7/2025 | 2 | 5,000 | EX-19-01(T) |
6/10/2025 | 6/11/2025 | 2 | 5,000 | EX-19-01(T) |
7/8/2025 | 7/9/2025 | 2 | 5,000 | EX-19-01(T) |
8/5/2025 | 8/6/2025 | 2 | 5,000 | EX-19-01(T) |
9/15/2025 | 9/16/2025 | 2 | 5,000 | EX-19-01(T) |
10/14/2025 | 10/15/2025 | 2 | 5,000 | EX-19-01(T) |
11/13/2025 | 11/14/2025 | 2 | 5,000 | EX-19-01(T) |
12/8/2025 | 12/9/2025 | 2 | 5,000 | EX-19-01(T) |
10/17/2024 | 10/18/2024 | 2 | 5,000 | EX-19-02(T) |
11/18/2024 | 11/19/2024 | 2 | 5,000 | EX-19-02(T) |
12/19/2024 | 12/20/2024 | 2 | 5,000 | EX-19-02(T) |
1/13/2025 | 1/14/2025 | 2 | 5,000 | EX-19-02(T) |
2/28/2025 | 3/1/2025 | 2 | 5,000 | EX-19-02(T) |
3/26/2025 | 3/27/2025 | 2 | 5,000 | EX-19-02(T) |
4/17/2025 | 4/18/2025 | 2 | 5,000 | EX-19-02(T) |
5/8/2025 | 5/9/2025 | 2 | 5,000 | EX-19-02(T) |
6/12/2025 | 6/13/2025 | 2 | 5,000 | EX-19-02(T) |
7/17/2025 | 7/18/2025 | 2 | 5,000 | EX-19-02(T) |
8/21/2025 | 8/22/2025 | 2 | 5,000 | EX-19-02(T) |
9/22/2025 | 9/23/2025 | 2 | 5,000 | EX-19-02(T) |
10/16/2025 | 10/17/2025 | 2 | 5,000 | EX-19-02(T) |
11/17/2025 | 11/18/2025 | 2 | 5,000 | EX-19-02(T) |
12/18/2025 | 12/19/2025 | 2 | 5,000 | EX-19-02(T) |
10/25/2024 | 10/25/2024 | 1 | 2,500 | EX-19-03(T) |
11/25/2024 | 11/25/2024 | 1 | 2,500 | EX-19-03(T) |
12/23/2024 | 12/23/2024 | 1 | 2,500 | EX-19-03(T) |
1/27/2025 | 1/27/2025 | 1 | 2,500 | EX-19-03(T) |
2/27/2025 | 2/27/2025 | 1 | 2,500 | EX-19-03(T) |
3/28/2025 | 3/28/2025 | 1 | 2,500 | EX-19-03(T) |
4/18/2025 | 4/18/2025 | 1 | 2,500 | EX-19-03(T) |
5/13/2025 | 5/13/2025 | 1 | 2,500 | EX-19-03(T) |
6/30/2025 | 6/30/2025 | 1 | 2,500 | EX-19-03(T) |
7/21/2025 | 7/21/2025 | 1 | 2,500 | EX-19-03(T) |
8/29/2025 | 8/29/2025 | 1 | 2,500 | EX-19-03(T) |
9/22/2025 | 9/22/2025 | 1 | 2,500 | EX-19-03(T) |
10/24/2025 | 10/24/2025 | 1 | 2,500 | EX-19-03(T) |
11/24/2025 | 11/24/2025 | 1 | 2,500 | EX-19-03(T) |
12/22/2025 | 12/22/2025 | 1 | 2,500 | EX-19-03(T) |
10/7/2024 | 10/8/2024 | 2 | 5,000 | PP-19-01(T) |
11/4/2024 | 11/5/2024 | 2 | 5,000 | PP-19-01(T) |
12/9/2024 | 12/10/2024 | 2 | 5,000 | PP-19-01(T) |
1/13/2025 | 1/14/2025 | 2 | 5,000 | PP-19-01(T) |
2/27/2025 | 2/28/2025 | 2 | 5,000 | PP-19-01(T) |
3/24/2025 | 3/25/2025 | 2 | 5,000 | PP-19-01(T) |
4/10/2025 | 4/11/2025 | 2 | 5,000 | PP-19-01(T) |
5/13/2025 | 5/14/2025 | 2 | 5,000 | PP-19-01(T) |
6/10/2025 | 6/11/2025 | 2 | 5,000 | PP-19-01(T) |
7/8/2025 | 7/9/2025 | 2 | 5,000 | PP-19-01(T) |
8/4/2025 | 8/5/2025 | 2 | 5,000 | PP-19-01(T) |
9/8/2025 | 9/9/2025 | 2 | 5,000 | PP-19-01(T) |
10/6/2025 | 10/7/2025 | 2 | 5,000 | PP-19-01(T) |
11/3/2025 | 11/4/2025 | 2 | 5,000 | PP-19-01(T) |
12/8/2025 | 12/9/2025 | 2 | 5,000 | PP-19-01(T) |
10/15/2024 | 10/16/2024 | 2 | 5,000 | PP-19-02(T) |
11/14/2024 | 11/15/2024 | 2 | 5,000 | PP-19-02(T) |
12/19/2024 | 12/20/2024 | 2 | 5,000 | PP-19-02(T) |
1/16/2025 | 1/17/2025 | 2 | 5,000 | PP-19-02(T) |
2/24/2025 | 2/25/2025 | 2 | 5,000 | PP-19-02(T) |
3/26/2025 | 3/27/2025 | 2 | 5,000 | PP-19-02(T) |
4/17/2025 | 4/18/2025 | 2 | 5,000 | PP-19-02(T) |
5/15/2025 | 5/16/2025 | 2 | 5,000 | PP-19-02(T) |
6/12/2025 | 6/13/2025 | 2 | 5,000 | PP-19-02(T) |
7/17/2025 | 7/18/2025 | 2 | 5,000 | PP-19-02(T) |
8/18/2025 | 8/19/2025 | 2 | 5,000 | PP-19-02(T) |
9/15/2025 | 9/16/2025 | 2 | 5,000 | PP-19-02(T) |
10/14/2025 | 10/15/2025 | 2 | 5,000 | PP-19-02(T) |
11/13/2025 | 11/14/2025 | 2 | 5,000 | PP-19-02(T) |
12/18/2025 | 12/19/2025 | 2 | 5,000 | PP-19-02(T) |
10/2/2024 | 10/4/2024 | 3 | 7,500 | PJ-19-US(T) |
11/6/2024 | 11/8/2024 | 3 | 7,500 | PJ-19-US(T) |
12/2/2024 | 12/4/2024 | 3 | 7,500 | PJ-19-US(T) |
1/8/2025 | 1/10/2025 | 3 | 7,500 | PJ-19-US(T) |
2/19/2025 | 2/21/2025 | 3 | 7,500 | PJ-19-US(T) |
3/12/2025 | 3/14/2025 | 3 | 7,500 | PJ-19-US(T) |
4/8/2025 | 4/10/2025 | 3 | 7,500 | PJ-19-US(T) |
5/7/2025 | 5/9/2025 | 3 | 7,500 | PJ-19-US(T) |
6/4/2025 | 6/6/2025 | 3 | 7,500 | PJ-19-US(T) |
7/7/2025 | 7/9/2025 | 3 | 7,500 | PJ-19-US(T) |
8/13/2025 | 8/15/2025 | 3 | 7,500 | PJ-19-US(T) |
9/3/2025 | 9/5/2025 | 3 | 7,500 | PJ-19-US(T) |
10/1/2025 | 10/3/2025 | 3 | 7,500 | PJ-19-US(T) |
11/5/2025 | 11/7/2025 | 3 | 7,500 | PJ-19-US(T) |
12/1/2025 | 12/3/2025 | 3 | 7,500 | PJ-19-US(T) |
10/25/2024 | 10/25/2024 | 1 | 2,500 | OL-19-01(T) |
11/25/2024 | 11/25/2024 | 1 | 2,500 | OL-19-01(T) |
12/23/2024 | 12/23/2024 | 1 | 2,500 | OL-19-01(T) |
1/20/2025 | 1/20/2025 | 1 | 2,500 | OL-19-01(T) |
2/28/2025 | 2/28/2025 | 1 | 2,500 | OL-19-01(T) |
3/24/2025 | 3/24/2025 | 1 | 2,500 | OL-19-01(T) |
4/11/2025 | 4/11/2025 | 1 | 2,500 | OL-19-01(T) |
5/16/2025 | 5/16/2025 | 1 | 2,500 | OL-19-01(T) |
6/30/2025 | 6/30/2025 | 1 | 2,500 | OL-19-01(T) |
7/21/2025 | 7/21/2025 | 1 | 2,500 | OL-19-01(T) |
8/25/2025 | 8/25/2025 | 1 | 2,500 | OL-19-01(T) |
9/22/2025 | 9/22/2025 | 1 | 2,500 | OL-19-01(T) |
10/24/2025 | 10/24/2025 | 1 | 2,500 | OL-19-01(T) |
11/24/2025 | 11/24/2025 | 1 | 2,500 | OL-19-01(T) |
12/22/2025 | 12/22/2025 | 1 | 2,500 | OL-19-01(T) |
4 | 56,400 | D101287GC10 | ||
6/4/2024 | 6/7/2024 | 4 | 49,000 | D90871GC20 |
7/2/2024 | 7/5/2024 | 4 | 49,000 | D90871GC20 |
8/13/2024 | 8/16/2024 | 4 | 49,000 | D90871GC20 |
9/10/2024 | 9/13/2024 | 4 | 49,000 | D90871GC20 |
10/1/2024 | 10/4/2024 | 4 | 49,000 | D90871GC20 |
11/5/2024 | 11/8/2024 | 4 | 49,000 | D90871GC20 |
12/10/2024 | 12/13/2024 | 4 | 49,000 | D90871GC20 |
5 | 56,500 | D66376GC51 | ||
1/20/2025 | 1/24/2025 | 5 | 57,900 | D61762GC51 |
3/17/2025 | 3/21/2025 | 5 | 57,900 | D61762GC51 |
5/26/2025 | 5/30/2025 | 5 | 57,900 | D61762GC51 |
7/21/2025 | 7/25/2025 | 5 | 57,900 | D61762GC51 |
9/22/2025 | 9/26/2025 | 5 | 57,900 | D61762GC51 |
11/17/2025 | 11/21/2025 | 5 | 57,900 | D61762GC51 |
11/5/2024 | 11/8/2024 | 4 | 57,000 | D109197GC10 |
12/10/2024 | 12/13/2024 | 4 | 57,000 | D109197GC10 |
2/18/2025 | 2/21/2025 | 4 | 57,000 | D109197GC10 |
4/22/2025 | 4/25/2025 | 4 | 57,000 | D109197GC10 |
6/24/2025 | 6/27/2025 | 4 | 57,000 | D109197GC10 |
8/19/2025 | 8/22/2025 | 4 | 57,000 | D109197GC10 |
10/20/2025 | 10/24/2025 | 4 | 57,000 | D109197GC10 |
12/16/2025 | 12/19/2025 | 4 | 57,000 | D109197GC10 |
2/17/2025 | 2/21/2025 | 5 | 57,900 | D66378GC50 |
4/21/2025 | 4/25/2025 | 5 | 57,900 | D66378GC50 |
6/23/2025 | 6/27/2025 | 5 | 57,900 | D66378GC50 |
8/18/2025 | 8/22/2025 | 5 | 57,900 | D66378GC50 |
12/15/2025 | 12/19/2025 | 5 | 57,900 | D66378GC50 |
6/27/2024 | 6/28/2024 | 2 | 36,900 | D108463GC10 |
7/30/2024 | 7/31/2024 | 2 | 36,900 | D108463GC10 |
8/29/2024 | 8/30/2024 | 2 | 36,900 | D108463GC10 |
9/23/2024 | 9/24/2024 | 2 | 36,900 | D108463GC10 |
10/24/2024 | 10/25/2024 | 2 | 36,900 | D108463GC10 |
11/28/2024 | 11/29/2024 | 2 | 36,900 | D108463GC10 |
12/23/2024 | 12/24/2024 | 2 | 36,900 | D108463GC10 |
6/10/2024 | 6/14/2024 | 5 | 75,000 | D1103970GC10 |
7/1/2024 | 7/5/2024 | 5 | 75,000 | D1103970GC10 |
8/5/2024 | 8/9/2024 | 5 | 75,000 | D1103970GC10 |
9/2/2024 | 9/6/2024 | 5 | 75,000 | D1103970GC10 |
9/30/2024 | 10/4/2024 | 5 | 75,000 | D1103970GC10 |
11/4/2024 | 11/8/2024 | 5 | 75,000 | D1103970GC10 |
12/9/2024 | 12/13/2024 | 5 | 75,000 | D1103970GC10 |
6/10/2024 | 6/14/2024 | 5 | 75,000 | D107122GC10 |
7/1/2024 | 7/5/2024 | 5 | 75,000 | D107122GC10 |
8/5/2024 | 8/9/2024 | 5 | 75,000 | D107122GC10 |
9/2/2024 | 9/6/2024 | 5 | 75,000 | D107122GC10 |
9/30/2024 | 10/4/2024 | 5 | 75,000 | D107122GC10 |
11/4/2024 | 11/8/2024 | 5 | 75,000 | D107122GC10 |
12/9/2024 | 12/13/2024 | 5 | 75,000 | D107122GC10 |
11/11/2024 | 11/15/2024 | 5 | 60,000 | D83529GC10 |
12/16/2024 | 12/20/2024 | 5 | 60,000 | D83529GC10 |
1/20/2025 | 1/24/2025 | 5 | 60,000 | D83529GC10 |
3/17/2025 | 3/21/2025 | 5 | 60,000 | D83529GC10 |
5/26/2025 | 5/30/2025 | 5 | 60,000 | D83529GC10 |
7/21/2025 | 7/25/2025 | 5 | 60,000 | D83529GC10 |
9/22/2025 | 9/26/2025 | 5 | 60,000 | D83529GC10 |
11/17/2025 | 11/21/2025 | 5 | 60,000 | D83529GC10 |
11/18/2024 | 11/22/2024 | 5 | 60,000 | D84840GC10 |
12/23/2024 | 12/27/2024 | 5 | 60,000 | D84840GC10 |
2/17/2025 | 2/21/2025 | 5 | 60,000 | D84840GC10 |
4/21/2025 | 4/25/2025 | 5 | 60,000 | D84840GC10 |
6/23/2025 | 6/27/2025 | 5 | 60,000 | D84840GC10 |
8/18/2025 | 8/22/2025 | 5 | 60,000 | D84840GC10 |
6/20/2024 | 6/21/2024 | 2 | 39,200 | D80897GC10 |
7/8/2024 | 7/9/2024 | 2 | 39,200 | D80897GC10 |
8/19/2024 | 8/20/2024 | 2 | 39,200 | D80897GC10 |
9/16/2024 | 9/17/2024 | 2 | 39,200 | D80897GC10 |
10/7/2024 | 10/8/2024 | 2 | 39,200 | D80897GC10 |
11/14/2024 | 11/15/2024 | 2 | 39,200 | D80897GC10 |
12/19/2024 | 12/20/2024 | 2 | 39,200 | D80897GC10 |
10/28/2024 | 10/30/2024 | 3 | 45,000 | D108305GC30 |
11/20/2024 | 11/22/2024 | 3 | 45,000 | D108305GC30 |
12/25/2024 | 12/27/2024 | 3 | 45,000 | D108305GC30 |
1/22/2025 | 1/24/2025 | 3 | 45,000 | D108305GC30 |
2/24/2025 | 2/26/2025 | 3 | 45,000 | D108305GC30 |
3/17/2025 | 3/19/2025 | 3 | 45,000 | D108305GC30 |
5/26/2025 | 5/28/2025 | 3 | 45,000 | D108305GC30 |
6/18/2025 | 6/20/2025 | 3 | 45,000 | D108305GC30 |
7/21/2025 | 7/23/2025 | 3 | 45,000 | D108305GC30 |
8/20/2025 | 8/22/2025 | 3 | 45,000 | D108305GC30 |
9/24/2025 | 9/26/2025 | 3 | 45,000 | D108305GC30 |
10/27/2025 | 10/29/2025 | 3 | 45,000 | D108305GC30 |
11/19/2025 | 11/21/2025 | 3 | 45,000 | D108305GC30 |
12/24/2025 | 12/26/2025 | 3 | 45,000 | D108305GC30 |
10/2/2024 | 10/4/2024 | 3 | 42,300 | D85767GC20 |
11/6/2024 | 11/8/2024 | 3 | 42,300 | D85767GC20 |
12/2/2024 | 12/4/2024 | 3 | 42,300 | D85767GC20 |
1/8/2025 | 1/10/2025 | 3 | 42,300 | D85767GC20 |
2/5/2025 | 2/7/2025 | 3 | 42,300 | D85767GC20 |
3/5/2025 | 3/7/2025 | 3 | 42,300 | D85767GC20 |
6/4/2025 | 6/6/2025 | 3 | 42,300 | D85767GC20 |
7/2/2025 | 7/4/2025 | 3 | 42,300 | D85767GC20 |
8/6/2025 | 8/8/2025 | 3 | 42,300 | D85767GC20 |
9/3/2025 | 9/5/2025 | 3 | 42,300 | D85767GC20 |
10/1/2025 | 10/3/2025 | 3 | 42,300 | D85767GC20 |
11/5/2025 | 11/7/2025 | 3 | 42,300 | D85767GC20 |
12/1/2025 | 12/3/2025 | 3 | 42,300 | D85767GC20 |
10/9/2024 | 10/11/2024 | 3 | 42,300 | D82658GC20 |
11/11/2024 | 11/13/2024 | 3 | 42,300 | D82658GC20 |
12/11/2024 | 12/13/2024 | 3 | 42,300 | D82658GC20 |
1/13/2025 | 1/15/2025 | 3 | 42,300 | D82658GC21 |
2/19/2025 | 2/21/2025 | 3 | 42,300 | D82658GC22 |
3/10/2025 | 3/12/2025 | 3 | 42,300 | D82658GC23 |
6/9/2025 | 6/11/2025 | 3 | 42,300 | D82658GC24 |
7/7/2025 | 7/9/2025 | 3 | 42,300 | D82658GC25 |
8/13/2025 | 8/15/2025 | 3 | 42,300 | D82658GC26 |
9/10/2025 | 9/12/2025 | 3 | 42,300 | D82658GC27 |
10/8/2025 | 10/10/2025 | 3 | 42,300 | D82658GC28 |
11/11/2025 | 11/13/2025 | 3 | 42,300 | D82658GC29 |
12/10/2025 | 12/12/2025 | 3 | 42,300 | D82658GC30 |
6/10/2024 | 6/14/2024 | 5 | 56,500 | D80151GC30 |
7/1/2024 | 7/5/2024 | 5 | 56,500 | D80151GC30 |
8/5/2024 | 8/9/2024 | 5 | 56,500 | D80151GC30 |
9/2/2024 | 9/6/2024 | 5 | 56,500 | D80151GC30 |
10/7/2024 | 10/11/2024 | 5 | 56,500 | D80151GC30 |
11/4/2024 | 11/8/2024 | 5 | 56,500 | D80151GC30 |
12/9/2024 | 12/13/2024 | 5 | 56,500 | D80151GC30 |
6/24/2024 | 6/28/2024 | 5 | 56,500 | D80155GC30 |
7/15/2024 | 7/19/2024 | 5 | 56,500 | D80155GC30 |
8/19/2024 | 8/23/2024 | 5 | 56,500 | D80155GC30 |
9/16/2024 | 9/20/2024 | 5 | 56,500 | D80155GC30 |
10/28/2024 | 11/1/2024 | 5 | 56,500 | D80155GC30 |
11/18/2024 | 11/22/2024 | 5 | 56,500 | D80155GC30 |
12/23/2024 | 12/27/2024 | 5 | 56,500 | D80155GC30 |
6/17/2024 | 6/19/2024 | 3 | 42,300 | D83173GC30 |
7/23/2024 | 7/25/2024 | 3 | 42,300 | D83173GC30 |
8/13/2024 | 8/15/2024 | 3 | 42,300 | D83173GC30 |
9/11/2024 | 9/13/2024 | 3 | 42,300 | D83173GC30 |
10/15/2024 | 10/17/2024 | 3 | 42,300 | D83173GC30 |
11/11/2024 | 11/13/2024 | 3 | 42,300 | D83173GC30 |
12/16/2024 | 12/18/2024 | 3 | 42,300 | D83173GC30 |
6/27/2024 | 6/28/2024 | 2 | 36,900 | D83177GC30 |
7/18/2024 | 7/19/2024 | 2 | 36,900 | D83177GC30 |
8/19/2024 | 8/20/2024 | 2 | 36,900 | D83177GC30 |
9/16/2024 | 9/17/2024 | 2 | 36,900 | D83177GC30 |
10/31/2024 | 11/1/2024 | 2 | 36,900 | D83177GC30 |
11/18/2024 | 11/19/2024 | 2 | 36,900 | D83177GC30 |
12/23/2024 | 12/24/2024 | 2 | 36,900 | D83177GC30 |
10/7/2024 | 10/11/2024 | 5 | 96,500 | D100928GC20 |
11/4/2024 | 11/8/2024 | 5 | 96,500 | D100928GC20 |
12/9/2024 | 12/13/2024 | 5 | 96,500 | D100928GC20 |
1/6/2025 | 1/10/2025 | 5 | 96,500 | |
2/3/2025 | 2/7/2025 | 5 | 96,500 | |
3/3/2025 | 3/7/2025 | 5 | 96,500 | |
6/9/2025 | 6/13/2025 | 5 | 96,500 | |
6/30/2025 | 7/4/2025 | 5 | 96,500 | |
8/4/2025 | 8/8/2025 | 5 | 96,500 | |
9/1/2025 | 9/5/2025 | 5 | 96,500 | |
10/6/2025 | 10/10/2025 | 5 | 96,500 | |
11/3/2025 | 11/7/2025 | 5 | 96,500 | |
12/8/2025 | 12/12/2025 | 5 | 96,500 | |
10/7/2024 | 10/11/2024 | 5 | 98,500 | D95507GC20(T) |
11/11/2024 | 11/15/2024 | 5 | 98,500 | D95507GC20(T) |
12/16/2024 | 12/20/2024 | 5 | 98,500 | D95507GC20(T) |
1/13/2025 | 1/17/2025 | 5 | 98,500 | D95507GC20(T) |
2/17/2025 | 2/21/2025 | 5 | 98,500 | D95507GC20(T) |
3/10/2025 | 3/14/2025 | 5 | 98,500 | D95507GC20(T) |
6/16/2025 | 6/20/2025 | 5 | 98,500 | D95507GC20(T) |
7/14/2025 | 7/18/2025 | 5 | 98,500 | D95507GC20(T) |
8/18/2025 | 8/22/2025 | 5 | 98,500 | D95507GC20(T) |
9/8/2025 | 9/12/2025 | 5 | 98,500 | D95507GC20(T) |
10/6/2025 | 10/10/2025 | 5 | 98,500 | D95507GC20(T) |
11/17/2025 | 11/21/2025 | 5 | 98,500 | D95507GC20(T) |
12/15/2025 | 12/19/2025 | 5 | 98,500 | D95507GC20(T) |
10/28/2024 | 11/1/2024 | 5 | 96,500 | D95503GC10 |
11/18/2024 | 11/22/2024 | 5 | 96,500 | D95503GC10 |
12/23/2024 | 12/27/2024 | 5 | 96,500 | D95503GC10 |
1/20/2025 | 1/24/2025 | 5 | 96,500 | D95503GC10 |
2/24/2025 | 2/28/2025 | 5 | 96,500 | D95503GC10 |
3/17/2025 | 3/21/2025 | 5 | 96,500 | D95503GC10 |
5/26/2025 | 5/30/2025 | 5 | 96,500 | D95503GC10 |
6/23/2025 | 6/27/2025 | 5 | 96,500 | D95503GC10 |
7/14/2025 | 7/18/2025 | 5 | 96,500 | D95503GC10 |
8/25/2025 | 8/29/2025 | 5 | 96,500 | D95503GC10 |
9/15/2025 | 9/19/2025 | 5 | 96,500 | D95503GC10 |
10/27/2025 | 10/31/2025 | 5 | 96,500 | D95503GC10 |
11/17/2025 | 11/21/2025 | 5 | 96,500 | D95503GC10 |
12/22/2025 | 12/26/2025 | 5 | 96,500 | D95503GC10 |
10/28/2024 | 10/30/2024 | 3 | 55,350 | D1104288GC10 |
11/25/2024 | 11/27/2024 | 3 | 55,350 | D1104288GC10 |
12/25/2024 | 12/27/2024 | 3 | 55,350 | D1104288GC10 |
1/27/2025 | 1/29/2025 | 3 | 55,350 | D1104288GC10 |
2/24/2025 | 2/26/2025 | 3 | 55,350 | D1104288GC10 |
3/26/2025 | 3/28/2025 | 3 | 55,350 | D1104288GC10 |
5/26/2025 | 5/28/2025 | 3 | 55,350 | D1104288GC10 |
6/23/2025 | 6/25/2025 | 3 | 55,350 | D1104288GC10 |
7/21/2025 | 7/23/2025 | 3 | 55,350 | D1104288GC10 |
8/25/2025 | 8/27/2025 | 3 | 55,350 | D1104288GC10 |
9/24/2025 | 9/26/2025 | 3 | 55,350 | D1104288GC10 |
10/27/2025 | 10/29/2025 | 3 | 55,350 | D1104288GC10 |
11/24/2025 | 11/26/2025 | 3 | 55,350 | D1104288GC10 |
12/22/2025 | 12/24/2025 | 3 | 55,350 | D1104288GC10 |
10/7/2024 | 10/11/2024 | 5 | 57,900 | D1101679GC10 |
11/4/2024 | 11/8/2024 | 5 | 57,900 | D1101679GC10 |
12/9/2024 | 12/13/2024 | 5 | 57,900 | D1101679GC10 |
1/20/2025 | 1/24/2025 | 5 | 57,900 | D1101679GC10 |
3/17/2025 | 3/21/2025 | 5 | 57,900 | D1101679GC10 |
5/26/2025 | 5/30/2025 | 5 | 57,900 | D1101679GC10 |
7/21/2025 | 7/25/2025 | 5 | 57,900 | D1101679GC10 |
9/22/2025 | 9/26/2025 | 5 | 57,900 | D1101679GC10 |
11/17/2025 | 11/21/2025 | 5 | 57,900 | D1101679GC10 |
10/28/2024 | 11/1/2024 | 5 | 57,900 | D1101997GC10 |
11/18/2024 | 11/22/2024 | 5 | 57,900 | D1101997GC10 |
12/23/2024 | 12/27/2024 | 5 | 57,900 | D1101997GC10 |
2/17/2025 | 2/21/2025 | 5 | 57,900 | D1101997GC10 |
4/21/2025 | 4/25/2025 | 5 | 57,900 | D1101997GC10 |
6/23/2025 | 6/27/2025 | 5 | 57,900 | D1101997GC10 |
8/18/2025 | 8/22/2025 | 5 | 57,900 | D1101997GC10 |
12/15/2025 | 12/19/2025 | 5 | 57,900 | D1101997GC10 |
10/28/2024 | 10/30/2024 | 3 | 45,000 | D1102677GC10 |
11/20/2024 | 11/22/2024 | 3 | 45,000 | D1102677GC10 |
12/25/2024 | 12/27/2024 | 3 | 45,000 | D1102677GC10 |
3/17/2025 | 3/19/2025 | 3 | 45,000 | D1102677GC10 |
5/26/2025 | 5/28/2025 | 3 | 45,000 | D1102677GC10 |
7/21/2025 | 7/23/2025 | 3 | 45,000 | D1102677GC10 |
9/22/2025 | 9/24/2025 | 3 | 45,000 | D1102677GC10 |
10/20/2025. | 10/22/2025 | 3 | 45,000 | D1102677GC10 |
10/31/2024 | 11/1/2024 | 2 | 40,000 | D1102679GC10 |
11/18/2024 | 11/19/2024 | 2 | 40,000 | D1102679GC10 |
12/23/2024 | 12/24/2024 | 2 | 40,000 | D1102679GC10 |
3/20/2025 | 3/21/2025 | 2 | 40,000 | D1102679GC10 |
5/29/2025 | 5/30/2025 | 2 | 40,000 | D1102679GC10 |
7/24/2025 | 7/25/2025 | 2 | 40,000 | D1102679GC10 |
9/25/2025 | 9/26/2025 | 2 | 40,000 | D1102679GC10 |
10/20/2025 | 10/21/2025 | 2 | 40,000 | D1102679GC10 |
10/7/2024 | 10/11/2024 | 5 | 57,900 | D108642GC10 |
11/18/2024 | 11/22/2024 | 5 | 57,900 | D108642GC10 |
12/16/2024 | 12/20/2024 | 5 | 57,900 | D108642GC10 |
1/13/2025 | 1/17/2025 | 5 | 57,900 | D108642GC10 |
2/17/2025 | 2/21/2025 | 5 | 57,900 | D108642GC10 |
3/10/2025 | 3/14/2025 | 5 | 57,900 | D108642GC10 |
4/7/2025 | 4/11/2025 | 5 | 57,900 | D108642GC10 |
5/5/2025 | 5/9/2025 | 5 | 57,900 | D108642GC10 |
6/9/2025 | 6/13/2025 | 5 | 57,900 | D108642GC10 |
7/14/2025 | 7/18/2025 | 5 | 57,900 | D108642GC10 |
8/4/2025 | 8/8/2025 | 5 | 57,900 | D108642GC10 |
9/1/2025 | 9/5/2025 | 5 | 57,900 | D108642GC10 |
9/29/2025 | 10/3/2025 | 5 | 57,900 | D108642GC10 |
11/3/2025 | 11/7/2025 | 5 | 57,900 | D108642GC10 |
12/15/2025 | 12/19/2025 | 5 | 57,900 | D108642GC10 |
10/7/2024 | 10/11/2024 | 5 | 57,900 | D108650GC10(T) |
11/18/2024 | 11/22/2024 | 5 | 57,900 | D108650GC10(T) |
12/16/2024 | 12/20/2024 | 5 | 57,900 | D108650GC10(T) |
1/13/2025 | 1/17/2025 | 5 | 57,900 | D108650GC10(T) |
2/17/2025 | 2/21/2025 | 5 | 57,900 | D108650GC10(T) |
3/10/2025 | 3/14/2025 | 5 | 57,900 | D108650GC10(T) |
4/7/2025 | 4/11/2025 | 5 | 57,900 | D108650GC10(T) |
5/5/2025 | 5/9/2025 | 5 | 57,900 | D108650GC10(T) |
6/9/2025 | 6/13/2025 | 5 | 57,900 | D108650GC10(T) |
7/14/2025 | 7/18/2025 | 5 | 57,900 | D108650GC10(T) |
8/4/2025 | 8/8/2025 | 5 | 57,900 | D108650GC10(T) |
9/1/2025 | 9/5/2025 | 5 | 57,900 | D108650GC10(T) |
9/29/2025 | 10/3/2025 | 5 | 57,900 | D108650GC10(T) |
11/3/2025 | 11/7/2025 | 5 | 57,900 | D108650GC10(T) |
12/15/2025 | 12/19/2025 | 5 | 57,900 | D108650GC10(T) |
10/28/2024 | 10/30/2024 | 3 | 45,000 | D1101121C10 |
11/25/2024 | 11/27/2024 | 3 | 45,000 | D1101121C10 |
12/25/2024 | 12/27/2024 | 3 | 45,000 | D1101121C10 |
1/22/2025 | 1/24/2025 | 3 | 45,000 | D1101121C10 |
3/19/2025 | 3/21/2025 | 3 | 45,000 | D1101121C10 |
4/23/2025 | 4/25/2025 | 3 | 45,000 | D1101121C10 |
5/21/2025 | 5/23/2025 | 3 | 45,000 | D1101121C10 |
6/18/2025 | 6/20/2025 | 3 | 45,000 | D1101121C10 |
7/21/2025 | 7/23/2025 | 3 | 45,000 | D1101121C10 |
8/25/2025 | 8/27/2025 | 3 | 45,000 | D1101121C10 |
9/8/2025 | 9/10/2025 | 3 | 45,000 | D1101121C10 |
10/6/2025 | 10/8/2025 | 3 | 45,000 | D1101121C10 |
11/10/2025 | 11/12/2025 | 3 | 45,000 | D1101121C10 |
1/21/2025 | 1/24/2025 | 4 | 57,000 | D108648GC10 |
3/18/2025 | 3/21/2025 | 4 | 57,000 | D108648GC10 |
4/22/2025 | 4/25/2025 | 4 | 57,000 | D108648GC10 |
5/20/2025 | 5/23/2025 | 4 | 57,000 | D108648GC10 |
6/17/2025 | 6/20/2025 | 4 | 57,000 | D108648GC10 |
7/22/2025 | 7/25/2025 | 4 | 57,000 | D108648GC10 |
8/19/2025 | 8/22/2025 | 4 | 57,000 | D108648GC10 |
9/9/2025 | 9/12/2025 | 4 | 57,000 | D108648GC10 |
10/7/2025 | 10/10/2025 | 4 | 57,000 | D108648GC10 |
11/11/2025 | 11/14/2025 | 4 | 57,000 | D108648GC10 |
12/23/2025 | 12/26/2025 | 4 | 57,000 | D108648GC10 |
10/31/2024 | 11/1/2024 | 2 | 36,900 | D105112GC10 |
11/28/2024 | 11/29/2024 | 2 | 36,900 | D105112GC10 |
12/23/2024 | 12/24/2024 | 2 | 36,900 | D105112GC10 |
1/21/2025 | 2 | 36,900 | D105112GC10 | |
3/18/2025 | 2 | 36,900 | D105112GC10 | |
4/22/2025 | 2 | 36,900 | D105112GC10 | |
5/22/2025 | 2 | 36,900 | D105112GC10 | |
6/26/2025 | 2 | 36,900 | D105112GC10 | |
7/29/2025 | 2 | 36,900 | D105112GC10 | |
8/28/2025 | 2 | 36,900 | D105112GC10 | |
9/22/2025 | 2 | 36,900 | D105112GC10 | |
10/30/2025 | 2 | 36,900 | D105112GC10 | |
11/24/2025 | 2 | 36,900 | D105112GC10 | |
12/22/2025 | 2 | 36,900 | D105112GC10 | |
58,600 | D1102675GC10 | |||
11/4/2024 | 11/8/2024 | 5 | 57,900 | D1102121GC10 |
12/9/2024 | 12/13/2024 | 5 | 57,900 | D1102121GC10 |
1/20/2025 | 1/24/2025 | 5 | 57,900 | D1102121GC10 |
4/21/2025 | 4/25/2025 | 5 | 57,900 | D1102121GC10 |
7/21/2025 | 7/25/2025 | 5 | 57,900 | D1102121GC10 |
10/20/2025 | 10/24/2025 | 5 | 57,900 | D1102121GC10 |
10/7/2024 | 10/11/2024 | 5 | 57,900 | D1102817GC10 |
11/11/2024 | 11/15/2024 | 5 | 57,900 | D1102817GC10 |
12/16/2024 | 12/20/2024 | 5 | 57,900 | D1102817GC10 |
2/17/2025 | 2/21/2025 | 5 | 57,900 | D1102817GC10 |
5/26/2025 | 5/30/2025 | 5 | 57,900 | D1102817GC10 |
8/18/2025 | 8/22/2025 | 5 | 57,900 | D1102817GC10 |
11/17/2025 | 11/21/2025 | 5 | 57,900 | D1102817GC10 |
10/28/2024 | 11/1/2024 | 5 | 57,900 | D1102819GC10 |
11/18/2024 | 11/22/2024 | 5 | 57,900 | D1102819GC10 |
12/23/2024 | 11/27/2024 | 5 | 57,900 | D1102819GC10 |
3/17/2025 | 3/21/2025 | 5 | 57,900 | D1102819GC10 |
6/23/2025 | 6/27/2025 | 5 | 57,900 | D1102819GC10 |
9/22/2025 | 9/26/2025 | 5 | 57,900 | D1102819GC10 |
12/15/2025 | 12/19/2025 | 5 | 57,900 | D1102819GC10 |
11/4/2024 | 11/8/2024 | 5 | 56,500 | D72898GC60 |
12/9/2024 | 12/13/2024 | 5 | 56,500 | D72898GC60 |
1/6/2025 | 1/10/2025 | 5 | 56,500 | D72898GC60 |
2/3/2025 | 2/7/2025 | 5 | 56,500 | D72898GC60 |
3/3/2025 | 3/7/2025 | 5 | 56,500 | D72898GC60 |
6/9/2025 | 6/13/2025 | 5 | 56,500 | D72898GC60 |
6/30/2025 | 7/4/2025 | 5 | 56,500 | D72898GC60 |
8/4/2025 | 8/8/2025 | 5 | 56,500 | D72898GC60 |
9/1/2025 | 9/5/2025 | 5 | 56,500 | D72898GC60 |
10/29/2025 | 10/3/2025 | 5 | 56,500 | D72898GC60 |
11/3/2025 | 11/7/2025 | 5 | 56,500 | D72898GC60 |
12/8/2025 | 12/12/2025 | 5 | 56,500 | D72898GC60 |
10/7/2024 | 10/11/2024 | 5 | 56,500 | D72967GC60 |
11/18/2024 | 11/22/2024 | 5 | 56,500 | D72967GC60 |
12/23/2024 | 12/27/2024 | 5 | 56,500 | D72967GC60 |
1/20/2025 | 1/24/2025 | 5 | 56,500 | D72967GC60 |
2/17/2025 | 2/21/2025 | 5 | 56,500 | D72967GC60 |
3/17/2025 | 3/21/2025 | 5 | 56,500 | D72967GC60 |
5/26/2025 | 5/30/2025 | 5 | 56,500 | D72967GC60 |
6/23/2025 | 6/27/2025 | 5 | 56,500 | D72967GC60 |
7/14/2025 | 7/18/2025 | 5 | 56,500 | D72967GC60 |
8/18/2025 | 8/22/2025 | 5 | 56,500 | D72967GC60 |
9/15/2025 | 9/19/2025 | 5 | 56,500 | D72967GC60 |
10/6/2025 | 10/10/2025 | 5 | 56,500 | D72967GC60 |
11/17/2025 | 11/21/2025 | 5 | 56,500 | D72967GC60 |
12/22/2025 | 12/26/2025 | 5 | 56,500 | D72967GC60 |
3/10/2025 | 3/14/2025 | 5 | 98,500 | D1101652GC10 |
6/16/2025 | 6/20/2025 | 5 | 98,500 | D1101652GC10 |
9/15/2025 | 9/19/2025 | 5 | 98,500 | D1101652GC10 |
12/15/2025 | 12/19/2025 | 5 | 98,500 | D1101652GC10 |
3/10/2025 | 3/14/2025 | 5 | 98,500 | SD1101652GC10 |
6/16/2025 | 6/20/2025 | 5 | 98,500 | SD1101652GC10 |
9/15/2025 | 9/19/2025 | 5 | 98,500 | SD1101652GC10 |
12/15/2025 | 12/19/2025 | 5 | 98,500 | SD1101652GC10 |
29,900 | D1103971GC20 | |||
56,500 | D1104310GC10 | |||
56,500 | D1102590GC10 | |||
56,500 | D1103613GC20 | |||
56,500 | D1104294GC10 | |||
56,500 | D1102591GC10 | |||
1/13/2025 | 1/17/2025 | 5 | 75,000 | D1106070GC10 |
2/17/2025 | 2/21/2025 | 5 | 75,000 | D1106070GC10 |
3/10/2025 | 3/14/2025 | 5 | 75,000 | D1106070GC10 |
4/7/2025 | 4/11/2025 | 5 | 75,000 | D1106070GC10 |
5/5/2025 | 5/9/2025 | 5 | 75,000 | D1106070GC10 |
6/9/2025 | 6/13/2025 | 5 | 75,000 | D1106070GC10 |
7/14/2025 | 7/18/2025 | 5 | 75,000 | D1106070GC10 |
8/4/2025 | 8/8/2025 | 5 | 75,000 | D1106070GC10 |
9/1/2025 | 9/5/2025 | 5 | 75,000 | D1106070GC10 |
9/29/2025 | 10/3/2025 | 5 | 75,000 | D1106070GC10 |
11/3/2025 | 11/7/2025 | 5 | 75,000 | D1106070GC10 |
12/15/2025 | 12/19/2025 | 5 | 75,000 | D1106070GC10 |
11/25/2024 | 11/25/2024 | 1 | 29,900 | D1101117GC10 |
12/2/2024 | 12/2/2024 | 1 | 29,900 | D1101117GC10 |
1/28/2025 | 1/28/2025 | 1 | 29,900 | D1101117GC10 |
3/25/2025 | 3/25/2025 | 1 | 29,900 | D1101117GC10 |
5/27/2025 | 5/27/2025 | 1 | 29,900 | D1101117GC10 |
8/26/2025 | 8/26/2025 | 1 | 29,900 | D1101117GC10 |
11/25/2025 | 11/25/2025 | 1 | 29,900 | D1101117GC10 |
11/26/2024 | 11/26/2024 | 1 | 29,900 | D1101118GC10 |
12/3/2024 | 12/3/2024 | 1 | 29,900 | D1101118GC10 |
1/29/2025 | 1/29/2025 | 1 | 29,900 | D1101118GC10 |
3/26/2025 | 3/26/2025 | 1 | 29,900 | D1101118GC10 |
5/28/2025 | 5/28/2025 | 1 | 29,900 | D1101118GC10 |
8/27/2025 | 8/27/2025 | 1 | 29,900 | D1101118GC10 |
11/26/2025 | 11/26/2025 | 1 | 29,900 | D1101118GC10 |
11/27/2024 | 11/27/2024 | 1 | 29,900 | D1101119GC10 |
12/4/2024 | 12/4/2024 | 1 | 29,900 | D1101119GC10 |
1/30/2025 | 1/30/2025 | 1 | 29,900 | D1101119GC10 |
3/27/2025 | 3/27/2025 | 1 | 29,900 | D1101119GC10 |
5/29/2025 | 5/29/2025 | 1 | 29,900 | D1101119GC10 |
8/28/2025 | 8/28/2025 | 1 | 29,900 | D1101119GC10 |
11/27/2025 | 11/27/2025 | 1 | 29,900 | D1101119GC10 |
11/28/2024 | 11/28/2024 | 1 | 29,900 | D1101120GC10 |
12/6/2024 | 12/6/2024 | 1 | 29,900 | D1101120GC10 |
1/31/2025 | 1/31/2025 | 1 | 29,900 | D1101120GC10 |
3/28/2025 | 3/28/2025 | 1 | 29,900 | D1101120GC10 |
5/30/2025 | 5/29/2025 | 1 | 29,900 | D1101120GC10 |
8/29/2025 | 8/15/2025 | 1 | 29,900 | D1101120GC10 |
11/28/2025 | 11/28/2025 | 1 | 29,900 | D1101120GC10 |
2/13/2025 | 2/14/2025 | 2 | 39,200 | D108519GC20 |
11/11/2024 | 11/15/2024 | 5 | 70,500 | D90846GC10 |
12/16/2024 | 12/20/2024 | 5 | 70,500 | D90846GC10 |
1/27/2025 | 1/31/2025 | 5 | 70,500 | D90846GC10 |
2/17/2025 | 2/21/2025 | 5 | 70,500 | D90846GC10 |
3/10/2025 | 3/14/2025 | 5 | 70,500 | D90846GC10 |
6/9/2025 | 6/13/2025 | 5 | 70,500 | D90846GC10 |
7/14/2025 | 7/18/2025 | 5 | 70,500 | D90846GC10 |
8/18/2025 | 8/22/2025 | 5 | 70,500 | D90846GC10 |
9/15/2025 | 9/19/2025 | 5 | 70,500 | D90846GC10 |
9/29/2025 | 10/3/2025 | 5 | 70,500 | D90846GC10 |
11/17/2025 | 11/21/2025 | 5 | 70,500 | D90846GC10 |
12/15/2025 | 12/19/2025 | 5 | 70,500 | D90846GC10 |
1/20/2025 | 1/24/2025 | 5 | 60,000 | D79655GC30 |
3/17/2025 | 3/21/2025 | 5 | 60,000 | D79655GC30 |
7/21/2025 | 7/25/2025 | 5 | 60,000 | D79655GC30 |
11/17/2025 | 11/21/2025 | 5 | 60,000 | D79655GC30 |
2/19/2025 | 2/21/2025 | 3 | 45,000 | D106776GC10 |
5/28/2025 | 5/30/2025 | 3 | 45,000 | D106776GC10 |
8/20/2025 | 8/22/2025 | 3 | 45,000 | D106776GC10 |
12/17/2025 | 12/19/2025 | 3 | 45,000 | D106776GC10 |
2/13/2025 | 2/14/2025 | 2 | 39,200 | D56259GC20 |
5/26/2025 | 5/27/2025 | 2 | 39,200 | D56259GC20 |
8/18/2025 | 8/19/2025 | 2 | 39,200 | D56259GC20 |
12/15/2025 | 12/16/2025 | 2 | 39,200 | D56259GC20 |
1/22/2025 | 1/26/2025 | 5 | 62,000 | 3627 |
2/19/2025 | 2/23/2025 | 5 | 62,000 | 3627 |
3/25/2025 | 3/29/2025 | 5 | 62,000 | 3627 |
4/22/2025 | 4/26/2025 | 5 | 62,000 | 3627 |
5/27/2025 | 5/31/2025 | 5 | 62,000 | 3627 |
6/24/2025 | 6/28/2025 | 5 | 62,000 | 3627 |
7/1/2025 | 7/5/2025 | 5 | 62,000 | 3627 |
8/26/2025 | 8/30/2025 | 5 | 62,000 | 3627 |
9/23/2025 | 9/27/2025 | 5 | 62,000 | 3627 |
10/28/2025 | 11/1/2025 | 5 | 62,000 | 3627 |
11/25/2025 | 11/29/2025 | 5 | 62,000 | 3627 |
12/23/2025 | 12/27/2025 | 5 | 62,000 | 3627 |
1/22/2025 | 1/26/2025 | 5 | 62,000 | 10025 |
2/19/2025 | 2/23/2025 | 5 | 62,000 | 10025 |
3/25/2025 | 3/29/2025 | 5 | 62,000 | 10025 |
4/22/2025 | 4/26/2025 | 5 | 62,000 | 10025 |
5/27/2025 | 5/31/2025 | 5 | 62,000 | 10025 |
6/24/2025 | 6/28/2025 | 5 | 62,000 | 10025 |
7/1/2025 | 7/5/2025 | 5 | 62,000 | 10025 |
8/26/2025 | 8/30/2025 | 5 | 62,000 | 10025 |
9/23/2025 | 9/27/2025 | 5 | 62,000 | 10025 |
10/28/2025 | 11/1/2025 | 5 | 62,000 | 10025 |
11/25/2025 | 11/29/2025 | 5 | 62,000 | 10025 |
12/23/2025 | 12/27/2025 | 5 | 62,000 | 10025 |
10/7/2024 | 10/8/2024 | 2 | 25,000 | 2444 |
11/4/2024 | 11/5/2024 | 2 | 25,000 | 2444 |
12/9/2024 | 12/10/2024 | 2 | 25,000 | 2444 |
1/6/2025 | 1/7/2025 | 2 | 25,000 | 2444 |
2/3/2025 | 2/4/2025 | 2 | 25,000 | 2444 |
3/3/2025 | 3/4/2025 | 2 | 25,000 | 2444 |
4/3/2025 | 4/4/2025 | 2 | 25,000 | 2444 |
5/8/2025 | 5/9/2025 | 2 | 25,000 | 2444 |
6/12/2025 | 6/13/2025 | 2 | 25,000 | 2444 |
7/3/2025 | 7/4/2025 | 2 | 25,000 | 2444 |
8/31/2025 | 9/1/2025 | 2 | 25,000 | 2444 |
9/1/2025 | 9/2/2025 | 2 | 25,000 | 2444 |
10/29/2025 | 10/30/2025 | 2 | 25,000 | 2444 |
11/3/2025 | 11/4/2025 | 2 | 25,000 | 2444 |
12/8/2025 | 12/9/2025 | 2 | 25,000 | 2444 |
10/9/2024 | 10/11/2024 | 3 | 39,500 | 2446 |
11/6/2024 | 11/8/2024 | 3 | 39,500 | 2446 |
12/11/2024 | 12/13/2024 | 3 | 39,500 | 2446 |
1/8/2025 | 1/10/2025 | 3 | 39,500 | 2446 |
2/5/2025 | 2/7/2025 | 3 | 39,500 | 2446 |
3/5/2025 | 3/7/2025 | 3 | 39,500 | 2446 |
4/2/2025 | 4/4/2025 | 3 | 39,500 | 2446 |
5/14/2025 | 5/16/2025 | 3 | 39,500 | 2446 |
6/9/2025 | 6/11/2025 | 3 | 39,500 | 2446 |
7/7/2025 | 7/9/2025 | 3 | 39,500 | 2446 |
8/6/2025 | 8/8/2025 | 3 | 39,500 | 2446 |
9/3/2025 | 9/5/2025 | 3 | 39,500 | 2446 |
10/1/2025 | 10/3/2025 | 3 | 39,500 | 2446 |
11/5/2025 | 11/7/2025 | 3 | 39,500 | 2446 |
12/10/2025 | 12/12/2025 | 3 | 39,500 | 2446 |
10/28/2024 | 11/1/2024 | 5 | 62,000 | 6639 |
11/25/2024 | 11/29/2024 | 5 | 62,000 | 6639 |
12/23/2024 | 12/27/2024 | 5 | 62,000 | 6639 |
1/20/2025 | 1/24/2025 | 5 | 62,000 | 6639 |
2/17/2025 | 2/21/2025 | 5 | 62,000 | 6639 |
3/24/2025 | 3/28/2025 | 5 | 62,000 | 6639 |
4/21/2025 | 4/25/2025 | 5 | 62,000 | 6639 |
5/26/2025 | 5/30/2025 | 5 | 62,000 | 6639 |
6/23/2025 | 6/27/2025 | 5 | 62,000 | 6639 |
7/14/2025 | 7/18/2025 | 5 | 62,000 | 6639 |
8/25/2025 | 8/29/2025 | 5 | 62,000 | 6639 |
9/22/2025 | 9/26/2025 | 5 | 62,000 | 6639 |
10/27/2025 | 10/31/2025 | 5 | 62,000 | 6639 |
11/24/2025 | 11/28/2025 | 5 | 62,000 | 6639 |
12/22/2025 | 12/26/2025 | 5 | 62,000 | 6639 |
10/15/2024 | 10/18/2024 | 4 | 52,000 | DP0119 |
11/19/2024 | 11/22/2024 | 4 | 52,000 | DP0119 |
12/17/2024 | 12/20/2024 | 4 | 52,000 | DP0119 |
1/14/2025 | 1/17/2025 | 4 | 52,000 | DP0119 |
2/18/2025 | 2/21/2025 | 4 | 52,000 | DP0119 |
3/18/2025 | 3/21/2025 | 4 | 52,000 | DP0119 |
4/8/2025 | 4/11/2025 | 4 | 52,000 | DP0119 |
5/13/2025 | 5/16/2025 | 4 | 52,000 | DP0119 |
6/17/2025 | 6/20/2025 | 4 | 52,000 | DP0119 |
7/22/2025 | 7/25/2025 | 4 | 52,000 | DP0119 |
8/19/2025 | 8/22/2025 | 4 | 52,000 | DP0119 |
9/16/2025 | 9/19/2025 | 4 | 52,000 | DP0119 |
10/14/2025 | 10/17/2025 | 4 | 52,000 | DP0119 |
11/18/2025 | 11/21/2025 | 4 | 52,000 | DP0119 |
12/16/2025 | 12/19/2025 | 4 | 52,000 | DP0119 |
10/28/2024 | 11/1/2024 | 5 | 62,000 | 7956 |
11/25/2024 | 11/29/2024 | 5 | 62,000 | 7956 |
12/23/2024 | 12/27/2024 | 5 | 62,000 | 7956 |
1/20/2025 | 1/24/2025 | 5 | 62,000 | 7956 |
2/17/2025 | 2/21/2025 | 5 | 62,000 | 7956 |
3/24/2025 | 3/28/2025 | 5 | 62,000 | 7956 |
4/21/2025 | 4/25/2025 | 5 | 62,000 | 7956 |
5/26/2025 | 5/30/2025 | 5 | 62,000 | 7956 |
6/23/2025 | 6/27/2025 | 5 | 62,000 | 7956 |
7/14/2025 | 7/18/2025 | 5 | 62,000 | 7956 |
8/25/2025 | 8/29/2025 | 5 | 62,000 | 7956 |
9/22/2025 | 9/26/2025 | 5 | 62,000 | 7956 |
10/27/2025 | 10/31/2025 | 5 | 62,000 | 7956 |
11/24/2025 | 11/28/2025 | 5 | 62,000 | 7956 |
12/22/2025 | 12/26/2025 | 5 | 62,000 | 7956 |
10/7/2024 | 10/11/2024 | 5 | 62,000 | 8256 |
11/18/2024 | 11/22/2024 | 5 | 62,000 | 8256 |
12/16/2024 | 12/20/2024 | 5 | 62,000 | 8256 |
1/13/2025 | 1/17/2025 | 5 | 62,000 | 8256 |
2/17/2025 | 2/21/2025 | 5 | 62,000 | 8256 |
3/17/2025 | 3/21/2025 | 5 | 62,000 | 8256 |
3/31/2025 | 4/4/2025 | 5 | 62,000 | 8256 |
5/19/2025 | 5/23/2025 | 5 | 62,000 | 8256 |
6/16/2025 | 6/20/2025 | 5 | 62,000 | 8256 |
7/21/2025 | 7/25/2025 | 5 | 62,000 | 8256 |
8/18/2025 | 8/22/2025 | 5 | 62,000 | 8256 |
9/15/2025 | 9/19/2025 | 5 | 62,000 | 8256 |
10/6/2025 | 10/10/2025 | 5 | 62,000 | 8256 |
11/17/2025 | 11/21/2025 | 5 | 62,000 | 8256 |
12/15/2025 | 12/19/2025 | 5 | 62,000 | 8256 |
10/7/2024 | 10/11/2024 | 5 | 62,000 | 8296 |
11/11/2024 | 11/15/2024 | 5 | 62,000 | 8296 |
12/9/2024 | 12/13/2024 | 5 | 62,000 | 8296 |
1/6/2025 | 1/10/2025 | 5 | 62,000 | 8296 |
2/3/2025 | 2/7/2025 | 5 | 62,000 | 8296 |
3/10/2025 | 3/14/2025 | 5 | 62,000 | 8296 |
4/1/2025 | 4/5/2025 | 5 | 62,000 | 8296 |
5/19/2025 | 5/23/2025 | 5 | 62,000 | 8296 |
6/9/2025 | 6/13/2025 | 5 | 62,000 | 8296 |
6/30/2025 | 7/4/2025 | 5 | 62,000 | 8296 |
8/4/2025 | 8/8/2025 | 5 | 62,000 | 8296 |
9/1/2025 | 9/5/2025 | 5 | 62,000 | 8296 |
10/6/2025 | 10/10/2025 | 5 | 62,000 | 8296 |
11/10/2025 | 11/14/2025 | 5 | 62,000 | 8296 |
12/8/2025 | 12/12/2025 | 5 | 62,000 | 8296 |
10/7/2024 | 10/11/2024 | 5 | 62,000 | 8726(T) |
11/4/2024 | 11/8/2024 | 5 | 62,000 | 8726(T) |
12/16/2024 | 12/20/2024 | 5 | 62,000 | 8726(T) |
1/13/2025 | 1/17/2025 | 5 | 62,000 | 8726(T) |
2/17/2025 | 2/21/2025 | 5 | 62,000 | 8726(T) |
3/17/2025 | 3/21/2025 | 5 | 62,000 | 8726(T) |
4/21/2025 | 4/25/2025 | 5 | 62,000 | 8726(T) |
5/19/2025 | 5/23/2025 | 5 | 62,000 | 8726(T) |
6/16/2025 | 6/20/2025 | 5 | 62,000 | 8726(T) |
7/14/2025 | 7/18/2025 | 5 | 62,000 | 8726(T) |
8/18/2025 | 8/22/2025 | 5 | 62,000 | 8726(T) |
9/15/2025 | 9/19/2025 | 5 | 62,000 | 8726(T) |
10/6/2025 | 10/10/2025 | 5 | 62,000 | 8726(T) |
11/17/2025 | 11/21/2025 | 5 | 62,000 | 8726(T) |
12/15/2025 | 12/19/2025 | 5 | 62,000 | 8726(T) |
10/28/2024 | 11/1/2024 | 5 | 62,000 | 9005(T) |
11/25/2024 | 11/29/2024 | 5 | 62,000 | 9005(T) |
12/23/2024 | 12/27/2024 | 5 | 62,000 | 9005(T) |
1/20/2025 | 1/24/2025 | 5 | 62,000 | 9005(T) |
2/24/2025 | 2/28/2025 | 5 | 62,000 | 9005(T) |
3/24/2025 | 3/28/2025 | 5 | 62,000 | 9005(T) |
4/21/2025 | 4/25/2025 | 5 | 62,000 | 9005(T) |
5/26/2025 | 5/30/2025 | 5 | 62,000 | 9005(T) |
6/23/2025 | 6/27/2025 | 5 | 62,000 | 9005(T) |
7/14/2025 | 7/18/2025 | 5 | 62,000 | 9005(T) |
8/25/2025 | 8/29/2025 | 5 | 62,000 | 9005(T) |
9/22/2025 | 9/26/2025 | 5 | 62,000 | 9005(T) |
10/27/2025 | 10/31/2025 | 5 | 62,000 | 9005(T) |
11/24/2025 | 11/28/2025 | 5 | 62,000 | 9005(T) |
12/22/2025 | 12/26/2025 | 5 | 62,000 | 9005(T) |
52,000 | 375(T) | |||
52,000 | 3225 | |||
62,000 | DP0095 | |||
62,000 | 6235 | |||
25,000 | DP0112 | |||
39,500 | DP0096 | |||
39,500 | 7622 | |||
39,500 | 7402 | |||
39,500 | 3221 | |||
52,000 | 8937 | |||
62,000 | HA0390 | |||
25,000 | DP0092 | |||
62,000 | DP0099 | |||
62,000 | DP0098 | |||
62,000 | 3100 | |||
52,000 | 9264 | |||
39,500 | 7545 | |||
62,000 | 6667 | |||
10/9/2024 | 10/11/2024 | 3 | 36,000 | TMAOCP(T) |
11/6/2024 | 11/8/2024 | 3 | 36,000 | TMAOCP(T) |
12/2/2024 | 12/4/2024 | 3 | 36,000 | TMAOCP(T) |
1/6/2025 | 1/8/2025 | 3 | 36,000 | TMAOCP(T) |
2/5/2025 | 2/7/2025 | 3 | 36,000 | TMAOCP(T) |
3/5/2025 | 3/7/2025 | 3 | 36,000 | TMAOCP(T) |
4/1/2025 | 4/3/2025 | 3 | 36,000 | TMAOCP(T) |
5/7/2025 | 5/9/2025 | 3 | 36,000 | TMAOCP(T) |
6/4/2025 | 6/6/2025 | 3 | 36,000 | TMAOCP(T) |
7/2/2025 | 7/4/2025 | 3 | 36,000 | TMAOCP(T) |
8/6/2025 | 8/8/2025 | 3 | 36,000 | TMAOCP(T) |
9/3/2025 | 9/5/2025 | 3 | 36,000 | TMAOCP(T) |
10/8/2025 | 10/10/2025 | 3 | 36,000 | TMAOCP(T) |
11/5/2025 | 11/7/2025 | 3 | 36,000 | TMAOCP(T) |
12/1/2025 | 12/3/2025 | 3 | 36,000 | TMAOCP(T) |
10/9/2024 | 10/11/2024 | 3 | 36,000 | TMDSCP20(T) |
11/11/2024 | 11/13/2024 | 3 | 36,000 | TMDSCP20(T) |
12/11/2024 | 12/13/2024 | 3 | 36,000 | TMDSCP20(T) |
1/13/2025 | 1/15/2025 | 3 | 36,000 | TMDSCP20(T) |
2/17/2025 | 2/19/2025 | 3 | 36,000 | TMDSCP20(T) |
3/12/2025 | 3/14/2025 | 3 | 36,000 | TMDSCP20(T) |
4/9/2025 | 4/11/2025 | 3 | 36,000 | TMDSCP20(T) |
5/14/2025 | 5/16/2025 | 3 | 36,000 | TMDSCP20(T) |
6/11/2025 | 6/13/2025 | 3 | 36,000 | TMDSCP20(T) |
7/7/2025 | 7/9/2025 | 3 | 36,000 | TMDSCP20(T) |
8/13/2025 | 8/15/2025 | 3 | 36,000 | TMDSCP20(T) |
9/10/2025 | 9/12/2025 | 3 | 36,000 | TMDSCP20(T) |
10/15/2025 | 10/17/2025 | 3 | 36,000 | TMDSCP20(T) |
11/10/2025 | 11/12/2025 | 3 | 36,000 | TMDSCP20(T) |
12/17/2025 | 12/19/2025 | 3 | 36,000 | TMDSCP20(T) |
10/28/2024 | 10/30/2024 | 3 | 36,000 | TMDDCP(T) |
11/20/2024 | 11/22/2024 | 3 | 36,000 | TMDDCP(T) |
12/16/2024 | 12/18/2024 | 3 | 36,000 | TMDDCP(T) |
1/20/2025 | 1/22/2025 | 3 | 36,000 | TMDDCP(T) |
2/26/2025 | 2/28/2025 | 3 | 36,000 | TMDDCP(T) |
3/19/2025 | 3/21/2025 | 3 | 36,000 | TMDDCP(T) |
4/23/2025 | 4/25/2025 | 3 | 36,000 | TMDDCP(T) |
5/21/2025 | 5/23/2025 | 3 | 36,000 | TMDDCP(T) |
6/18/2025 | 6/20/2025 | 3 | 36,000 | TMDDCP(T) |
7/16/2025 | 7/18/2025 | 3 | 36,000 | TMDDCP(T) |
8/20/2025 | 8/22/2025 | 3 | 36,000 | TMDDCP(T) |
9/24/2025 | 9/26/2025 | 3 | 36,000 | TMDDCP(T) |
10/28/2025 | 10/30/2025 | 3 | 36,000 | TMDDCP(T) |
11/19/2025 | 11/21/2025 | 3 | 36,000 | TMDDCP(T) |
12/22/2025 | 12/24/2025 | 3 | 36,000 | TMDDCP(T) |
CT-ITF+(T) | ||||
10/7/2024 | 10/11/2024 | 5 | 48,000 | CT01-Network+(T) |
11/11/2024 | 11/15/2024 | 5 | 48,000 | CT01-Network+(T) |
12/9/2024 | 12/13/2024 | 5 | 48,000 | CT01-Network+(T) |
1/20/2025 | 1/24/2025 | 5 | 48,000 | CT01-Network+(T) |
4/21/2025 | 4/25/2025 | 5 | 48,000 | CT01-Network+(T) |
7/21/2025 | 7/25/2025 | 5 | 48,000 | CT01-Network+(T) |
10/6/2025 | 10/10/2025 | 5 | 48,000 | CT01-Network+(T) |
10/15/2024 | 10/17/2024 | 3 | 39,000 | CT02-Network+(T) |
11/20/2024 | 11/22/2024 | 3 | 39,000 | CT02-Network+(T) |
12/16/2024 | 12/18/2024 | 3 | 39,000 | CT02-Network+(T) |
1/29/2025 | 1/31/2025 | 3 | 39,000 | CT02-Network+(T) |
4/28/2025 | 4/30/2025 | 3 | 39,000 | CT02-Network+(T) |
6/30/2025 | 7/2/2025 | 3 | 39,000 | CT02-Network+(T) |
10/15/2025 | 10/17/2025 | 3 | 39,000 | CT02-Network+(T) |
10/7/2024 | 10/11/2024 | 5 | 48,000 | CT01-Security+(T) |
11/4/2024 | 11/8/2024 | 5 | 48,000 | CT01-Security+(T) |
12/9/2024 | 12/13/2024 | 5 | 48,000 | CT01-Security+(T) |
1/20/2025 | 1/24/2025 | 5 | 48,000 | CT01-Security+(T) |
4/21/2025 | 4/25/2025 | 5 | 48,000 | CT01-Security+(T) |
7/21/2025 | 7/25/2025 | 5 | 48,000 | CT01-Security+(T) |
10/6/2025 | 10/10/2025 | 5 | 48,000 | CT01-Security+(T) |
10/16/2024 | 10/18/2024 | 3 | 39,000 | CT02-Security+(T) |
11/13/2024 | 11/15/2024 | 3 | 39,000 | CT02-Security+(T) |
12/18/2024 | 12/20/2024 | 3 | 39,000 | CT02-Security+(T) |
1/29/2025 | 1/31/2025 | 3 | 39,000 | CT02-Security+(T) |
4/28/2025 | 4/30/2025 | 3 | 39,000 | CT02-Security+(T) |
7/30/2025 | 8/1/2025 | 3 | 39,000 | CT02-Security+(T) |
10/15/2025 | 10/17/2025 | 3 | 39,000 | CT02-Security+(T) |
10/7/2024 | 10/11/2024 | 5 | 45,000 | CT-Cloud+(T) |
11/18/2024 | 11/22/2024 | 5 | 45,000 | CT-Cloud+(T) |
12/16/2024 | 12/20/2024 | 5 | 45,000 | CT-Cloud+(T) |
2/3/2025 | 2/7/2025 | 5 | 45,000 | CT-Cloud+(T) |
5/19/2025 | 5/23/2025 | 5 | 45,000 | CT-Cloud+(T) |
8/18/2025 | 8/22/2025 | 5 | 45,000 | CT-Cloud+(T) |
11/10/2025 | 11/14/2025 | 5 | 45,000 | CT-Cloud+(T) |
2/3/2025 | 2/7/2025 | 5 | 48,000 | CT-Data+ |
5/19/2025 | 5/23/2025 | 5 | 48,000 | CT-Data+ |
8/18/2025 | 8/8/2025 | 5 | 48,000 | CT-Data+ |
11/10/2025 | 11/7/2025 | 5 | 48,000 | CT-Data+ |
3/3/2025 | 3/7/2025 | 5 | 54,000 | CT-DataSys+ |
6/16/2025 | 6/20/2025 | 5 | 54,000 | CT-DataSys+ |
9/1/2025 | 9/5/2025 | 5 | 54,000 | CT-DataSys+ |
12/15/2025 | 12/19/2025 | 5 | 54,000 | CT-DataSys+ |
2/3/2025 | 2/7/2025 | 5 | 45,000 | CT-Linux+ |
5/19/2025 | 5/23/2025 | 5 | 45,000 | CT-Linux+ |
8/18/2025 | 8/22/2025 | 5 | 45,000 | CT-Linux+ |
11/10/2025 | 11/14/2025 | 5 | 45,000 | CT-Linux+ |
10/7/2024 | 10/11/2024 | 5 | 45,000 | CT-Server+(T) |
11/18/2024 | 11/22/2024 | 5 | 45,000 | CT-Server+(T) |
12/16/2024 | 12/20/2024 | 5 | 45,000 | CT-Server+(T) |
2/17/2025 | 2/21/2025 | 5 | 45,000 | CT-Server+(T) |
5/26/2025 | 5/30/2025 | 5 | 45,000 | CT-Server+(T) |
8/25/2025 | 8/29/2025 | 5 | 45,000 | CT-Server+(T) |
11/17/2025 | 11/21/2025 | 5 | 45,000 | CT-Server+(T) |
10/7/2024 | 10/11/2024 | 5 | 48,000 | CT01-CySA+(T) |
11/4/2024 | 11/8/2024 | 5 | 48,000 | CT01-CySA+(T) |
12/9/2024 | 12/13/2024 | 5 | 48,000 | CT01-CySA+(T) |
3/10/2025 | 3/14/2025 | 5 | 48,000 | CT01-CySA+(T) |
6/9/2025 | 6/13/2025 | 5 | 48,000 | CT01-CySA+(T) |
9/8/2025 | 9/12/2025 | 5 | 48,000 | CT01-CySA+(T) |
12/15/2025 | 12/19/2025 | 5 | 48,000 | CT01-CySA+(T) |
10/16/2024 | 10/18/2024 | 3 | 39,000 | CT02-CySA+(T) |
11/25/2024 | 11/27/2024 | 3 | 39,000 | CT02-CySA+(T) |
12/11/2024 | 12/13/2024 | 3 | 39,000 | CT02-CySA+(T) |
3/19/2025 | 3/21/2025 | 3 | 39,000 | CT02-CySA+(T) |
6/18/2025 | 6/20/2025 | 3 | 39,000 | CT02-CySA+(T) |
9/17/2025 | 9/19/2025 | 3 | 39,000 | CT02-CySA+(T) |
12/22/2025 | 12/24/2025 | 3 | 39,000 | CT02-CySA+(T) |
10/7/2024 | 10/11/2024 | 5 | 48,000 | CT-PenTest+(T) |
12/23/2024 | 12/27/2024 | 5 | 48,000 | CT-PenTest+(T) |
1/27/2025 | 1/31/2025 | 5 | 48,000 | CT-PenTest+(T) |
3/24/2025 | 3/28/2025 | 5 | 48,000 | CT-PenTest+(T) |
6/23/2025 | 6/27/2025 | 5 | 48,000 | CT-PenTest+(T) |
9/22/2025 | 9/26/2025 | 5 | 48,000 | CT-PenTest+(T) |
12/15/2025 | 12/19/2025 | 5 | 48,000 | CT-PenTest+(T) |
10/28/2024 | 11/1/2024 | 5 | 54,000 | CT-CASP+(T) |
11/18/2024 | 11/22/2024 | 5 | 54,000 | CT-CASP+(T) |
12/9/2024 | 12/13/2024 | 5 | 54,000 | CT-CASP+(T) |
1/27/2025 | 1/31/2025 | 5 | 54,000 | CT-CASP+(T) |
4/21/2025 | 4/25/2025 | 5 | 54,000 | CT-CASP+(T) |
7/21/2025 | 7/25/2025 | 5 | 54,000 | CT-CASP+(T) |
10/27/2025 | 10/31/2025 | 5 | 54,000 | CT-CASP+(T) |
10/2/2024 | 10/4/2024 | 3 | 28,000 | CT-Cloud Essentials+(T) |
1/22/2025 | 1/24/2025 | 3 | 28,000 | CT-Cloud Essentials+(T) |
4/21/2025 | 4/25/2025 | 3 | 28,000 | CT-Cloud Essentials+(T) |
7/21/2025 | 7/25/2025 | 3 | 28,000 | CT-Cloud Essentials+(T) |
10/6/2025 | 10/8/2025 | 3 | 28,000 | CT-Cloud Essentials+(T) |
10/2/2024 | 10/4/2024 | 3 | 45,000 | CT-Project+(T) |
1/15/2025 | 1/17/2025 | 3 | 45,000 | CT-Project+(T) |
3/30/2025 | 4/4/2025 | 3 | 45,000 | CT-Project+(T) |
7/14/2025 | 7/18/2025 | 3 | 45,000 | CT-Project+(T) |
9/29/2025 | 10/3/2025 | 3 | 45,000 | CT-Project+(T) |
11/25/2024 | 11/29/2024 | 5 | 42,000 | CT-A+(T) |
12/16/2024 | 12/20/2024 | 5 | 42,000 | CT-A+(T) |
1/13/2025 | 1/17/2025 | 5 | 42,000 | CT-A+(T) |
4/7/2025 | 4/11/2025 | 5 | 42,000 | CT-A+(T) |
7/14/2025 | 7/18/2025 | 5 | 42,000 | CT-A+(T) |
9/29/2025 | 10/3/2025 | 5 | 42,000 | CT-A+(T) |
10/28/2024 | 10/30/2024 | 3 | 22,000 | ITIL-V4(T) |
11/20/2024 | 11/22/2024 | 3 | 22,000 | ITIL-V4(T) |
12/25/2024 | 12/27/2024 | 3 | 22,000 | ITIL-V4(T) |
1/16/2025 | 1/17/2025 | 2 | 22,000 | ITIL-V4(T) |
2/20/2025 | 2/21/2025 | 2 | 22,000 | ITIL-V4(T) |
3/27/2025 | 3/28/2025 | 2 | 22,000 | ITIL-V4(T) |
4/24/2025 | 4/25/2025 | 2 | 22,000 | ITIL-V4(T) |
5/29/2025 | 5/30/2025 | 2 | 22,000 | ITIL-V4(T) |
6/19/2025 | 6/20/2025 | 2 | 22,000 | ITIL-V4(T) |
7/17/2025 | 7/18/2025 | 2 | 22,000 | ITIL-V4(T) |
8/28/2025 | 8/29/2025 | 2 | 22,000 | ITIL-V4(T) |
9/25/2025 | 9/26/2025 | 2 | 22,000 | ITIL-V4(T) |
10/30/2025 | 10/31/2025 | 2 | 22,000 | ITIL-V4(T) |
11/20/2025 | 11/21/2025 | 2 | 22,000 | ITIL-V4(T) |
12/25/2025 | 12/26/2025 | 2 | 22,000 | ITIL-V4(T) |
10/28/2024 | 10/30/2024 | 3 | 48,500 | ITIL-V4+Exam(T) |
11/20/2024 | 11/22/2024 | 3 | 48,500 | ITIL-V4+Exam(T) |
12/25/2024 | 12/27/2024 | 3 | 48,500 | ITIL-V4+Exam(T) |
1/16/2025 | 1/17/2025 | 2 | 48,500 | ITIL-V4+Exam(T) |
2/20/2025 | 2/21/2025 | 2 | 48,500 | ITIL-V4+Exam(T) |
3/27/2025 | 3/28/2025 | 2 | 48,500 | ITIL-V4+Exam(T) |
4/24/2025 | 4/25/2025 | 2 | 48,500 | ITIL-V4+Exam(T) |
5/29/2025 | 5/30/2025 | 2 | 48,500 | ITIL-V4+Exam(T) |
6/19/2025 | 6/20/2025 | 2 | 48,500 | ITIL-V4+Exam(T) |
7/17/2025 | 7/18/2025 | 2 | 48,500 | ITIL-V4+Exam(T) |
8/28/2025 | 8/29/2025 | 2 | 48,500 | ITIL-V4+Exam(T) |
9/25/2025 | 9/26/2025 | 2 | 48,500 | ITIL-V4+Exam(T) |
10/30/2025 | 10/31/2025 | 2 | 48,500 | ITIL-V4+Exam(T) |
11/20/2025 | 11/21/2025 | 2 | 48,500 | ITIL-V4+Exam(T) |
12/25/2025 | 12/26/2025 | 2 | 48,500 | ITIL-V4+Exam(T) |
10/9/2024 | 10/11/2024 | 3 | 9,500 | PYTN102(T) |
11/11/2024 | 11/13/2024 | 3 | 9,500 | PYTN102(T) |
12/11/2024 | 12/13/2024 | 3 | 9,500 | PYTN102(T) |
1/8/2025 | 1/10/2025 | 3 | 9,500 | PYTN102(T) |
2/19/2025 | 2/21/2025 | 3 | 9,500 | PYTN102(T) |
3/10/2025 | 3/12/2025 | 3 | 9,500 | PYTN102(T) |
4/9/2025 | 4/11/2025 | 3 | 9,500 | PYTN102(T) |
5/14/2025 | 5/16/2025 | 3 | 9,500 | PYTN102(T) |
6/9/2025 | 6/11/2025 | 3 | 9,500 | PYTN102(T) |
7/7/2025 | 7/9/2025 | 3 | 9,500 | PYTN102(T) |
8/13/2025 | 8/15/2025 | 3 | 9,500 | PYTN102(T) |
9/10/2025 | 9/12/2025 | 3 | 9,500 | PYTN102(T) |
10/8/2025 | 10/10/2025 | 3 | 9,500 | PYTN102(T) |
11/12/2025 | 11/14/2025 | 3 | 9,500 | PYTN102(T) |
12/17/2025 | 12/19/2025 | 3 | 9,500 | PYTN102(T) |
10/9/2024 | 10/11/2024 | 3 | 7,500 | PYTN103(T) |
11/11/2024 | 11/13/2024 | 3 | 7,500 | PYTN103(T) |
12/11/2024 | 12/13/2024 | 3 | 7,500 | PYTN103(T) |
1/8/2025 | 1/10/2025 | 3 | 7,500 | PYTN103(T) |
2/19/2025 | 2/21/2025 | 3 | 7,500 | PYTN103(T) |
3/10/2025 | 3/12/2025 | 3 | 7,500 | PYTN103(T) |
4/9/2025 | 4/11/2025 | 3 | 7,500 | PYTN103(T) |
5/14/2025 | 5/16/2025 | 3 | 7,500 | PYTN103(T) |
6/9/2025 | 6/11/2025 | 3 | 7,500 | PYTN103(T) |
7/7/2025 | 7/9/2025 | 3 | 7,500 | PYTN103(T) |
8/13/2025 | 8/15/2025 | 3 | 7,500 | PYTN103(T) |
9/10/2025 | 9/12/2025 | 3 | 7,500 | PYTN103(T) |
10/8/2025 | 10/10/2025 | 3 | 7,500 | PYTN103(T) |
11/12/2025 | 11/14/2025 | 3 | 7,500 | PYTN103(T) |
12/17/2025 | 12/19/2025 | 3 | 7,500 | PYTN103(T) |
10/28/2024 | 11/1/2024 | 5 | 19,900 | PYTN104(T) |
11/25/2024 | 11/29/2024 | 5 | 19,900 | PYTN104(T) |
12/23/2024 | 12/27/2024 | 5 | 19,900 | PYTN104(T) |
1/20/2025 | 1/24/2025 | 5 | 19,900 | PYTN104(T) |
2/24/2025 | 2/28/2025 | 5 | 19,900 | PYTN104(T) |
3/24/2025 | 3/28/2025 | 5 | 19,900 | PYTN104(T) |
4/21/2025 | 4/25/2025 | 5 | 19,900 | PYTN104(T) |
5/26/2025 | 5/30/2025 | 5 | 19,900 | PYTN104(T) |
6/23/2025 | 6/27/2025 | 5 | 19,900 | PYTN104(T) |
7/14/2025 | 7/18/2025 | 5 | 19,900 | PYTN104(T) |
8/25/2025 | 8/29/2025 | 5 | 19,900 | PYTN104(T) |
9/22/2025 | 9/26/2025 | 5 | 19,900 | PYTN104(T) |
10/27/2025 | 10/31/2025 | 5 | 19,900 | PYTN104(T) |
11/24/2025 | 11/28/2025 | 5 | 19,900 | PYTN104(T) |
12/22/2025 | 12/26/2025 | 5 | 19,900 | PYTN104(T) |
9/30/2024 | 10/4/2024 | 5 | 35,000 | PMP7(T) |
4/23/2025 | 4/25/2025 | 5 | 35,000 | PMP7(T) |
6/14/2024 | 6/14/2024 | 1 | 4,000 | AI-050T00 |
7/15/2024 | 7/15/2024 | 1 | 4,000 | AI-050T00 |
8/16/2024 | 8/16/2024 | 1 | 4,000 | AI-050T00 |
9/9/2024 | 9/9/2024 | 1 | 4,000 | AI-050T00 |
10/18/2024 | 10/18/2024 | 1 | 4,000 | AI-050T00 |
11/11/2024 | 11/11/2024 | 1 | 4,000 | AI-050T00 |
12/16/2024 | 12/16/2024 | 1 | 4,000 | AI-050T00 |
10/18/2024 | 10/18/2024 | 1 | 4,000 | DP-601T00 |
11/11/2024 | 11/11/2024 | 1 | 4,000 | DP-601T00 |
12/16/2024 | 12/16/2024 | 1 | 4,000 | DP-601T00 |
1/17/2025 | 1/17/2025 | 1 | 4,000 | DP-601T00 |
2/14/2025 | 2/14/2025 | 1 | 4,000 | DP-601T00 |
3/14/2025 | 3/14/2025 | 1 | 4,000 | DP-601T00 |
4/18/2025 | 4/18/2025 | 1 | 4,000 | DP-601T00 |
5/19/2025 | 5/19/2025 | 1 | 4,000 | DP-601T00 |
6/13/2025 | 6/13/2025 | 1 | 4,000 | DP-601T00 |
7/14/2025 | 7/14/2025 | 1 | 4,000 | DP-601T00 |
8/15/2025 | 8/15/2025 | 1 | 4,000 | DP-601T00 |
9/15/2025 | 9/15/2025 | 1 | 4,000 | DP-601T00 |
10/17/2025 | 10/17/2025 | 1 | 4,000 | DP-601T00 |
11/14/2025 | 11/14/2025 | 1 | 4,000 | DP-601T00 |
12/15/2025 | 12/15/2025 | 1 | 4,000 | DP-601T00 |
10/22/2024 | 10/22/2024 | 1 | 4,000 | DP-604T00 |
11/15/2024 | 11/15/2024 | 1 | 4,000 | DP-604T00 |
12/13/2024 | 12/13/2024 | 1 | 4,000 | DP-604T00 |
1/6/2025 | 1/6/2025 | 1 | 4,000 | DP-604T00 |
2/14/2025 | 2/14/2025 | 1 | 4,000 | DP-604T00 |
3/7/2025 | 3/7/2025 | 1 | 4,000 | DP-604T00 |
4/11/2025 | 4/11/2025 | 1 | 4,000 | DP-604T00 |
5/2/2025 | 5/2/2025 | 1 | 4,000 | DP-604T00 |
6/6/2025 | 6/6/2025 | 1 | 4,000 | DP-604T00 |
7/14/2025 | 7/14/2025 | 1 | 4,000 | DP-604T00 |
8/15/2025 | 8/15/2025 | 1 | 4,000 | DP-604T00 |
9/12/2025 | 9/12/2025 | 1 | 4,000 | DP-604T00 |
10/10/2025 | 10/10/2025 | 1 | 4,000 | DP-604T00 |
11/7/2025 | 11/7/2025 | 1 | 4,000 | DP-604T00 |
12/12/2025 | 12/12/2025 | 1 | 4,000 | DP-604T00 |
2 | 4,000 | IC-002T00 | ||
10/8/2024 | 10/11/2024 | 4 | 16,000 | DP-203T00 |
11/12/2024 | 11/15/2024 | 4 | 16,000 | DP-203T00 |
12/10/2024 | 12/13/2024 | 4 | 16,000 | DP-203T00 |
1/14/2025 | 1/17/2025 | 4 | 16,000 | DP-203T00 |
2/10/2025 | 2/14/2025 | 4 | 16,000 | DP-203T00 |
3/11/2025 | 3/14/2025 | 4 | 16,000 | DP-203T00 |
4/1/2025 | 4/4/2025 | 4 | 16,000 | DP-203T00 |
5/13/2025 | 5/16/2025 | 4 | 16,000 | DP-203T00 |
6/17/2025 | 6/20/2025 | 4 | 16,000 | DP-203T00 |
7/15/2025 | 7/18/2025 | 4 | 16,000 | DP-203T00 |
8/26/2025 | 8/29/2025 | 4 | 16,000 | DP-203T00 |
9/9/2025 | 9/12/2025 | 4 | 16,000 | DP-203T00 |
10/7/2025 | 10/10/2025 | 4 | 16,000 | DP-203T00 |
11/11/2025 | 11/14/2025 | 4 | 16,000 | DP-203T00 |
12/9/2025 | 12/12/2025 | 4 | 16,000 | DP-203T00 |